Using LocalBroadcastManager in Android
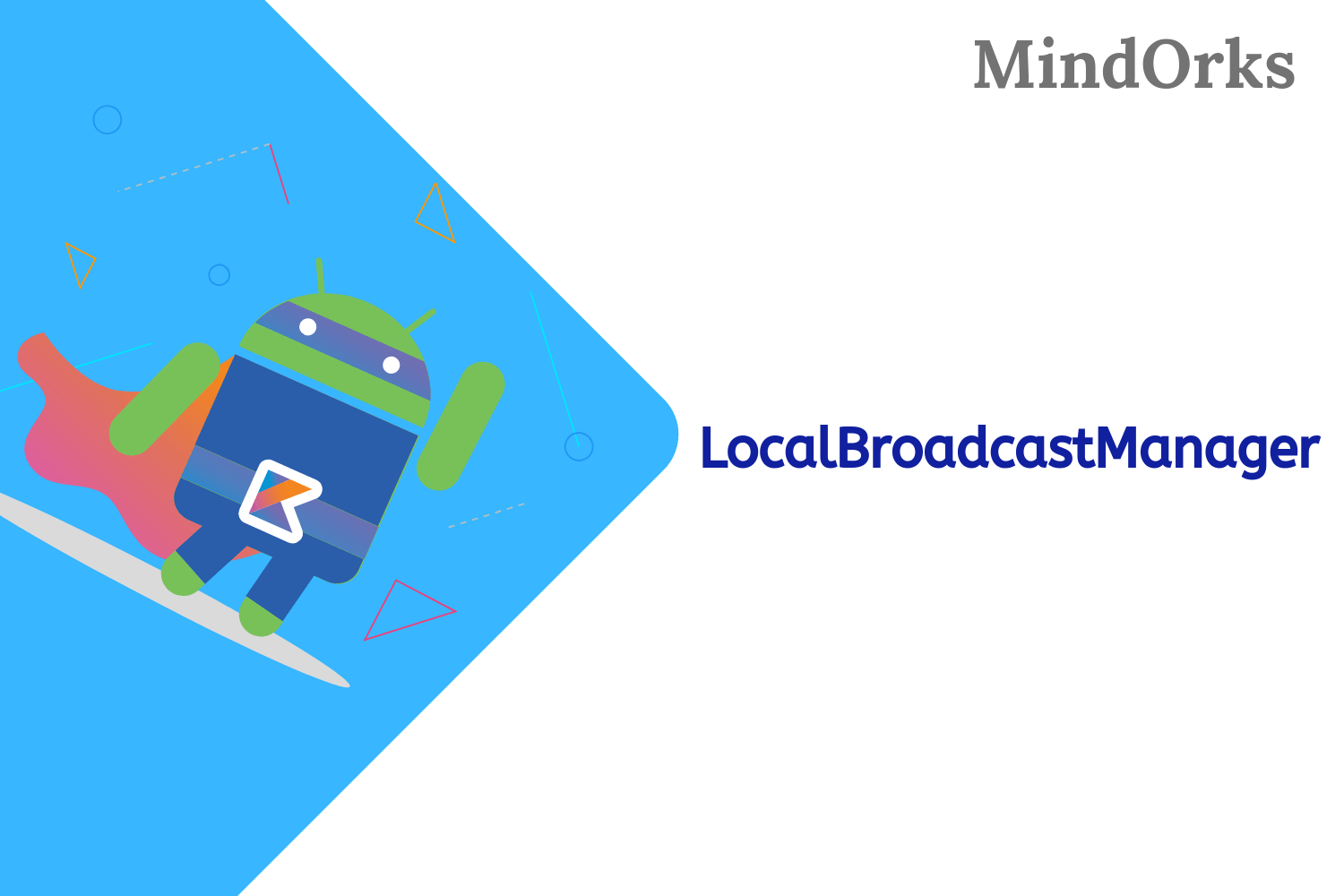
In Android, we use a Broadcast Receiver to send a particular message to every application that is associated with that specific Broadcast. It is same as that of Youtube channel subscription. If you have subscribed to a Youtube channel then whenever the creator of the channel will upload some video you will be notified about the same. So, in the same way, Android applications can subscribe or register to certain system events like battery low and in return, these applications will get notified whenever the subscribed event will occur. But these are Global Broadcasts and should not be used in every case because it has some demerits. So, in this blog, we will learn the concept of LocalBroadcastManager in Android. So, let’s get started.
How to use Broadcast?
Before getting into LocalBroadcastManager, let’s have a quick revision of how to use Broadcast in our application. The whole process of using Broadcast can be divided into two parts:
- Register Broadcast
- Receive Broadcast
Register Broadcast: Firstly, you have to register for a broadcast in your application. For example, if you want to receive the Battery low event, then you have to register this in your application. So, to register a particular broadcast in your application, you have two options, either you can register the event in the AndroidManifest.xml file of your application or you can register the broadcast via the Context.registerReceiver() method.
Receiver Broadcast: By extending the BroadcastReceiver abstract class, you can receive the broadcast in your application. After extending, all you need to do is override the onReceive() method and perform the required action in the onReceive() method because this onReceive() method will be called when a particular broadcast is received.
LocalBroadcastManager
If the communication is not between different applications on the Android device then is it suggested not to use the Global BroadcastManager because there can be some security holes while using Global Broadcastmanager and you don’t have to worry about this if you are using LocalBroadcastManager.LocalBroadcastManager is used to register and send a broadcast of intents to local objects in your process. It has lots of advantages:
- You broadcasting data will not leave your app. So, if there is some leakage in your app then you need not worry about that.
- Another thing that can be noted here is that other applications can’t send any kind of broadcasts to your app. So, you need not worry about security holes.
How to use LocalBroadcastReceiver?
To use LocalBroadcastReceiver, all you need to do is create an instance of LocalBroadcastManager, then send the broadcast and finally receive the broadcast. So, firstly, create an instance of the LocalBroadcastManager:
var localBroadcastManager = LocalBroadcastManager.getInstance(context)
Now, by using the sendBroadcast() method, you can send the broadcast as below:
val localIntent = Intent("YOUR_ACTION")
.putExtra("DATA", "MindOrks")
localBroadcastManager.sendBroadcast(localIntent)
Our final task is to receive the broadcast using the onReceive() method on MyBroadCastReceiver. You can perform the desired action after receiving the broadcast in the onReceive() method as below:
private val listener = MyBroadcastReceiver()
inner class MyBroadcastReceiver : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent){
when (intent.action) {
"YOUR_ACTION" -> {
val data = intent.getStringExtra("DATA")
Log.d("Your Received data : ", data)
}
else -> Toast.makeText(context, "Action Not Found", Toast.LENGTH_LONG).show()
}
}
}
Now, this will print an output in Logcat,
Your Received data : MindOrks
So, these are the three basic steps that can be done to use LocalBroadcastManager in our Android application.
Note:
- Here, you can perform multiple action in onReceive() of the broadcast Receiver based on different actions.
Conclusion
If you want some kind of broadcasting in your application then you should use the concept of LocalBroadcastManager and we should avoid using the Global Broadcast because for using Global Broadcast you have to ensure that there are no security holes that can leak your data to other applications.
Hope you learned how to use LocalBroadcastManager. To find more cool articles on Android, you can visit our blogging website and read some blogs on Android.
Keep Learning :)
Team MindOrks!