Using Custom and Downloadable Fonts in Android
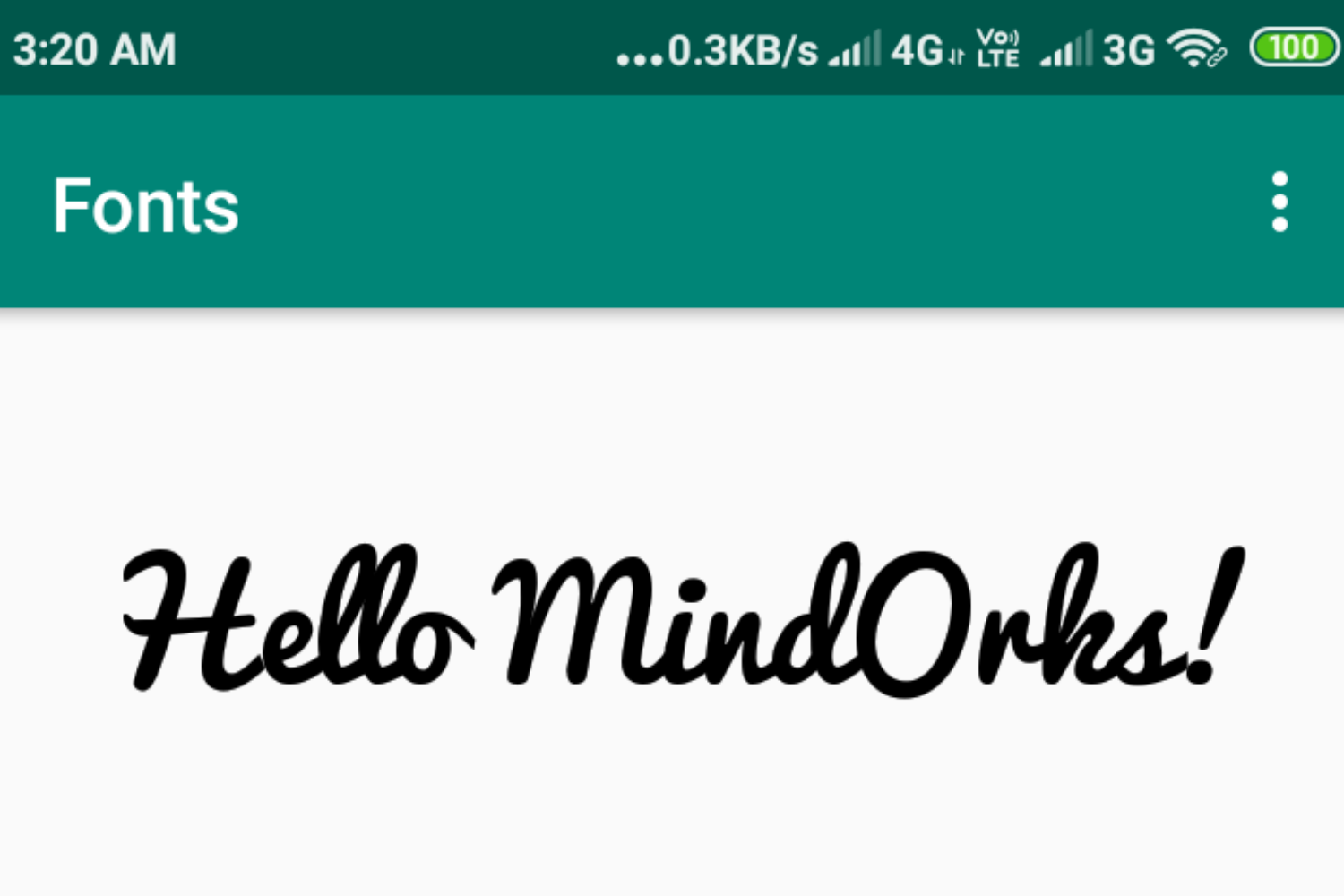
At the Google I/O 2017, Android O or simple Android Oreo was launched and it came with a lot of cool features. One of the really interesting features for developers was the new way to apply fonts right in your XML files. Yeah, you heard it right. Now there is no need of writing some piece of code for using the fonts. Also, you can choose from any of the thousands of fonts on Google Fonts and use them in your app.
So, in this blog, you will understand how to use Custom and Downloadable Fonts in Android?
The Older way of using Fonts
Before we move forward to look for the new or the latest way of using the font, let's revise the older way of using fonts. Before the release of Android O, fonts can be used in the following 2 ways :
- Using Typeface: One would typically need a custom view that extends the equivalent view were trying to apply a font to. In the custom view, one would create a Typeface and then call setTypeface (or a similar method, that, sets the typeface). One would also need to have the font file placed in the assets folder. The code in the custom view typically looks like:
TextView tv = (TextView)findViewById(R.id.textview1);
Typeface custom_font = Typeface.createFromAsset(getAssets(), "fonts/font-name.ttf");
tx.setTypeface(custom_font);
- Calligraphy Library : You can use some existing libraries for various fonts used in Android. One of them is Calligraphy Library .
Using Custom Font in Android
To work with Custom Font, you need to install the latest version of Android Studio 3.x. This is important as some of the features are not supported on Android Studio 2.x - for example, the font resource directory. Once you are done with the installation of the latest version of Android Studio, create a project and add a text view in any of the activity(This is the text view on which we are going to apply custom font). Then follow the below steps :
- Add a font directory to your project: In the Android View, right click on the res folder and go to New -> Android Resource Directory. Type font as the name of the font and select font as the resource type. Then click on Ok .
- Add the downloaded font to the font directory: Copy and Paste your font into res/font. I am using Pacifico font . You can get this font from FontSqirrel .
- Create a font-family XML file: You can create font families which contain a set of font files with their style and weight details. To create a new font family you need to create a new XML font resource. The benefit is that you can access it as a single unit instead of referencing individual font files for each style and weight. To create a font-family, right click on res/font and choose New -> Font Resource File and give any name. In my case, I am using my_custom_font.xml .
<?xml version="1.0" encoding="utf-8"?>
<font-family xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<!-- regular -->
<font
android:font="@font/Pacifico"
android:fontStyle="normal"
android:fontWeight="400"
app:font="@font/Pacifico"
app:fontStyle="normal"
app:fontWeight="400" />
<!-- italic -->
<font
android:font="@font/Pacifico"
android:fontStyle="italic"
android:fontWeight="400"
app:font="@font/Pacifico"
app:fontStyle="italic"
app:fontWeight="400" />
<!-- bold -->
<font
android:font="@font/Pacifico"
android:fontStyle="normal"
android:fontWeight="700"
app:font="@font/Pacifico"
app:fontStyle="normal"
app:fontWeight="700" />
</font-family>
Note:
A really important thing to note is that we had to define attributes using both
android
and
app
namespaces. The
app
namespace is what ensures that the feature is backward compatible.
- Set the font in the XML: Now you can use the font-family attribute to set the font in XML.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="@font/my_custom_font"
android:text="Hello MindOrks!"
android:textColor="#000"/>
Below is the preview of the above code :
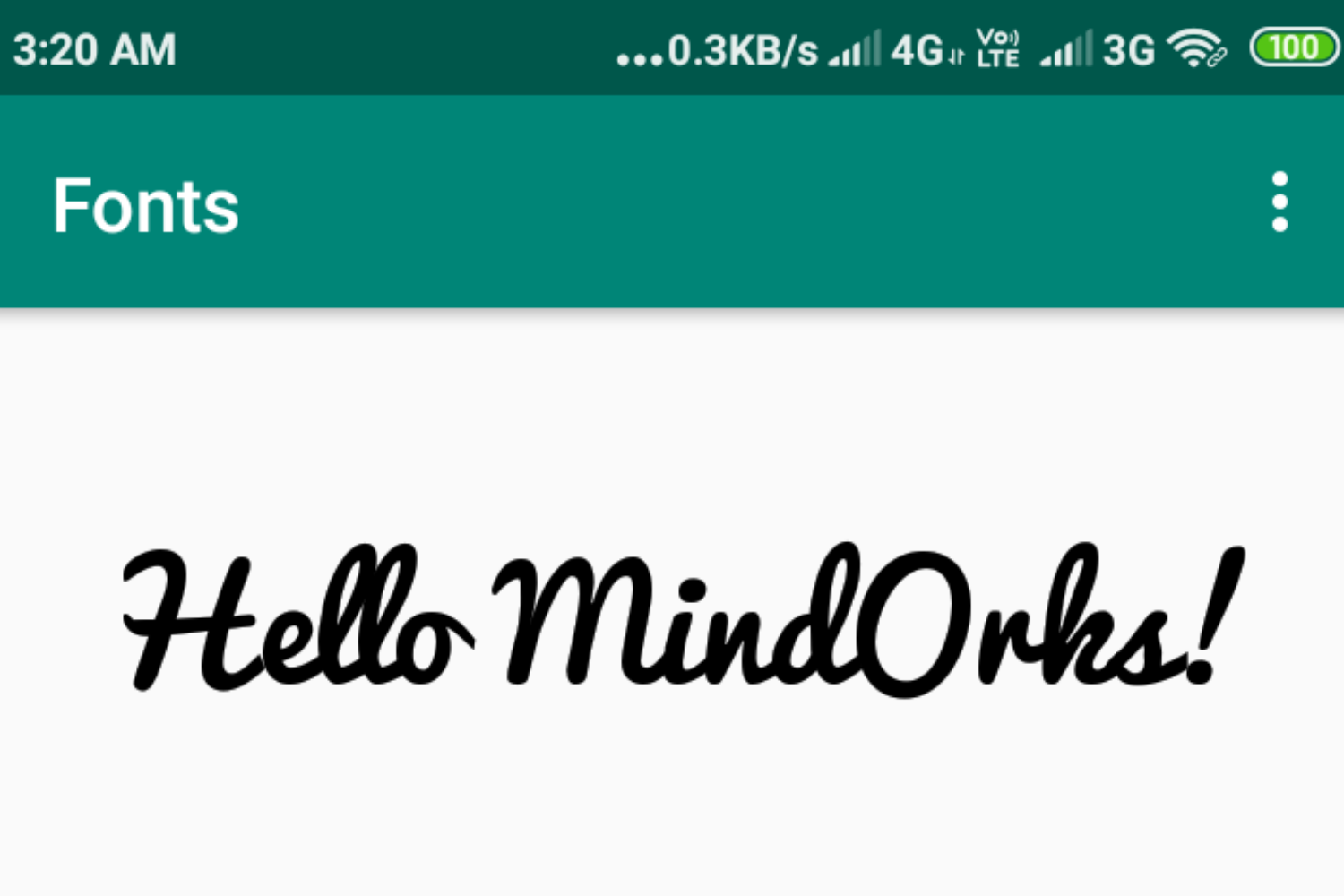
Downloadable Fonts
Now that we have seen how custom fonts work, let's jump onto another novality - downloadable font . Android 8.0 (API level 26) and Android Support Library 26 introduce support for APIs to request fonts from a provider application instead of bundling files into APK or letting the APK download fonts. A font provider application retrieves fonts and caches them locally so that other apps can request and share fonts. How cool is that!
The feature is available on devices running Android API version 14 and higher through the Support Library 26.
Picture courtesy : Android Developer website
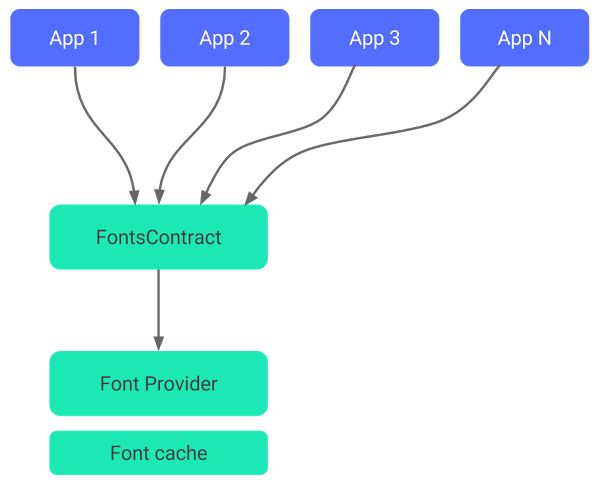
As you can see in the image above, apps using Downloadable Fonts make a FontRequest using the FontsContract API which retrieves the Typeface from the Font Provider. The Font Provider does not need to download fonts if it already exists in the Font Cache.
Benefits of downloadable fonts :
- Reduces the APK size
- Increase the app installation success rate
- Improves the overall system health as multiple APKs can share the same font through a provider. This saves users cellular data, phone memory, and disk space. In this model, the font is fetched over the network when needed.
In order to use the downloadable font in android studio, follow the below steps :
- If you want to use Android Studio to generate the required files, then you’ll need version 3.0+. Add the following (version 26+) to your module’s build.gradle:
implementation 'com.android.support:appcompat-v7:28.0.0'
- Select a text view in your app that you want to apply the font to and click on the fontFamily attribute under Attributes in the graphical layout
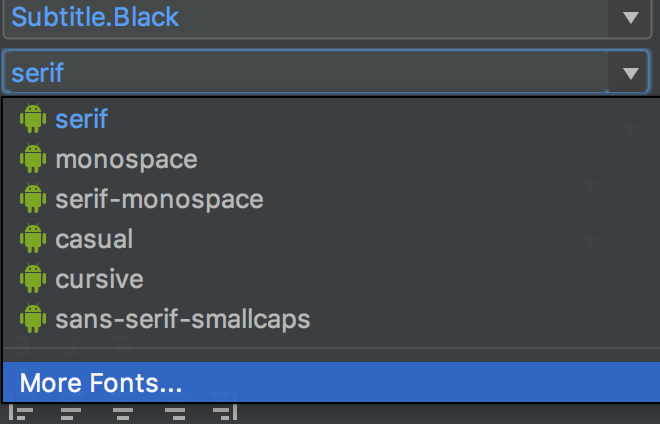
Select the “More Fonts…” at the bottom, which will open the dialog below.
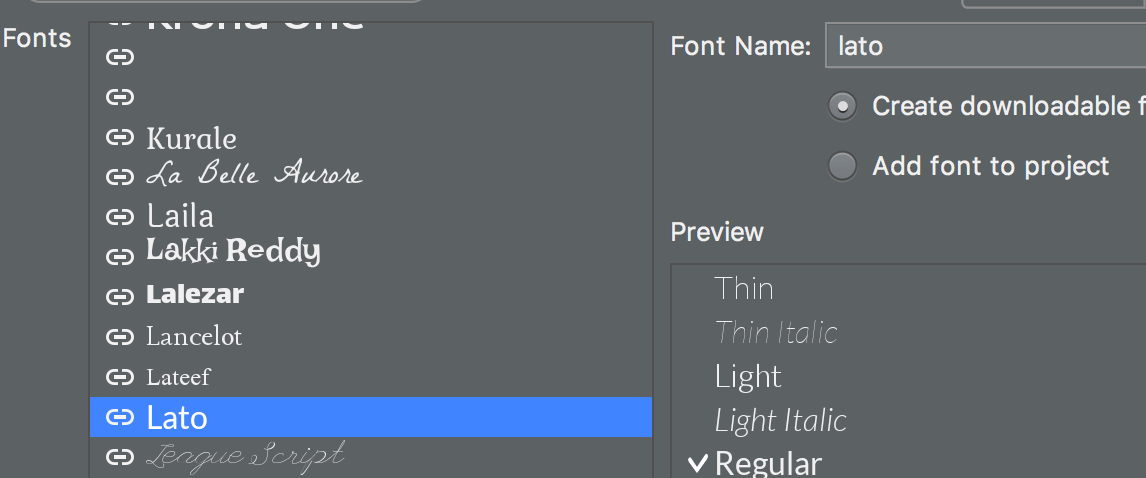
- Make sure to have “ Create downloadable font ” selected. This results in three files being downloaded — lato.xml, font_certs.xml and preloaded_fonts.xml.
lato.xml
This file contains the font attributes for loading a Typeface from the Google Fonts Provider Application.
<?xml version="1.0" encoding="utf-8"?>
<font-family xmlns:app="http://schemas.android.com/apk/res-auto"
app:fontProviderAuthority="com.google.android.gms.fonts"
app:fontProviderPackage="com.google.android.gms"
app:fontProviderQuery="Lato"
app:fontProviderCerts="@array/com_google_android_gms_fonts_certs">
</font-family>
font_certs.xml
The system uses these certificates to verify the font provider’s identity, to avoid getting fonts from an unknown source. If using the steps above, Android Studio should have automatically generated the string certificates for dev and prod in font_certs.xml below.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<array name="com_google_android_gms_fonts_certs">
<item>@array/com_google_android_gms_fonts_certs_dev</item>
<item>@array/com_google_android_gms_fonts_certs_prod</item>
</array>
<string-array name="com_google_android_gms_fonts_certs_dev">
<item>
<!-- string cert -->
</item>
</string-array>
<string-array name="com_google_android_gms_fonts_certs_prod">
<item>
<!-- string cert-->
</item>
</string-array>
</resources>
preloaded-fonts.xml
This file is referenced in the Android manifest which helps the framework pre-load fonts to avoid delays when the app is launched.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<array name="preloaded_fonts" translatable="false">
<item>@font/lato</item>
<item>@font/lato_bold</item>
</array>
</resources>
- Make sure this line is added to your app’s Manifest file, Android Studio should have done this automatically:
<meta-data
android:name="preloaded_fonts"
android:resource="@array/preloaded_fonts"/>
- Great, now you are ready to apply the fonts in XML!
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
....
<item name="android:fontFamily">@font/lato</item>
</style>
All I had to do was set the font family in the app’s theme to get TextViews throughout the app to change to Lato, including parts that were bold or italicized. However, if you want to configure the weights, you can follow the same steps to get Lato Bold using Android Studio, and change the weight manually in lato_bold.xml that you can then apply in XML layouts:
app:fontProviderQuery="name=Lato&weight=700" //can modify the weight here
That's all about the custom and downloadable fonts in android. Hope you like the blog.
Happy learning
Team MindOrks :)