Parsing JSON in Android
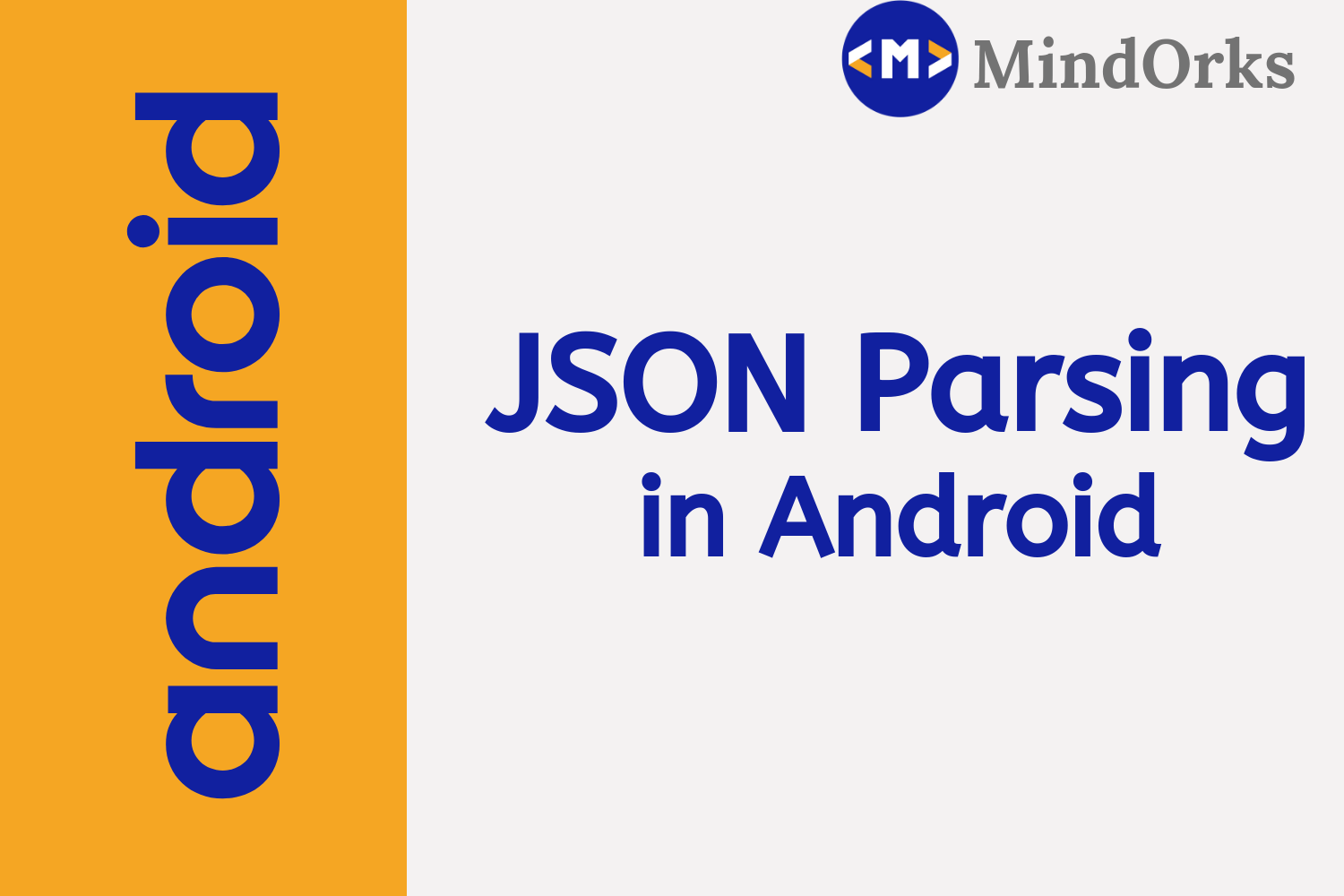
Sharing data over the Internet is a very common task. Today, almost every website is having some kind of user’s data or data for the user. For example, take the example of any online shopping website. Here in the website, the data can be the details of your past and upcoming order. These order details contain the name of the item, date of order placement, tracking id, the current status of the order and many more details of related to the order. These data are stored in a particular format. These formats can be XML file format or JSON file format.
When you want to use the data store using these file formats in the database, then you have to apply some kind of parsing of data in your Android Application. For JSON file you have to use the JSON parsing and for XML files, you can use the XML parsing.
In this blog, we will learn how to parse the JSON format data and use it in our Android Application. If you want to do the XML parsing then after reading this blog, you can refer to our blog of XML parsing . So, let’s get started with JSON parsing.
What is JSON?
JSON stands for JavaScript Object Notation. It is used to interchange data from the server to the desired place. XML parsing is more complex as compared to JSON parsing. Apart from this, JSON is light weighted, structured and is an independent data exchange format that is used to parse data. The best part about JSON is that, nowadays, most of the APIs that are available to us are sending data in the form of JSON and in order to receive that data from API, you have to do JSON parsing and it is very easy to do JSON parsing.
In order to manipulate JSON data and to play with JSON data, Android provides four classes. These classes are:
- JSONObject
- JSONArray
- JSONStringer
- JSONTokenizer
JSON Example
Before moving on to JSON parsing, we have look how the actual JSON looks like. Also, we will look upon the elements of JSON data and then move on to the JSON parsing example.
So, let’s understand how the JSON file looks like. Following is an example of a simple JSON file:
[
{
"id": 912345678901,
"text": "How do I read JSON on Android?",
"geo": null,
"user": {
"name": "android_newb",
"followers_count": 41
}
},
{
"id": 912345678902,
"text": "@android_newb just use android.util.JsonReader!",
"geo": [50.454722, -104.606667],
"user": {
"name": "jesse",
"followers_count": 2
}
}
]
In the above example, we are having the data of users of a mobile application. The information present in this file is the id of the user, text created by the user, name of the user and the total number of followers of a particular user. Basically, there are four elements of a
912345678901
particular JSON file. These are:
- JSON Array: In the JSON file, you can put the data in the form of JSON array i.e. it behaves like simple array i.e. you can store data one after the other and can access that data using a zero-based indexing. Inside the JSON array, you can put values like String, boolean, Integer, float, etc. Apart from these, you can put some other JSON array or JSON object in a particular JSON array. Square bracket ([]) represents a JSON array.
- JSON Object: You can store data in JSON using JSON object also i.e. you can store data by assigning some key and values to a particular data. For example, the key can be id and the value can be “ 912345678901 ”. Curly brackets ({}) represents a JSON object.
- Key: A normal JSON object contains a String value that can be used to retrieve a particular data from the JSON object. For example, in the above example, keys can be id, text, geo, name, and followers_count .
- Values: Values can be in any primitive data and is the value corresponding to a particular key. For example, in the above example, the value for id is 912345678901, value for name is android_newb and so on.
JSON Array Parsing Functions
Before parsing the JSON data, you have look for the JSON type i.e. you have to find if the JSON data is in the form of object or array. You can easily identify this by looking at your JSON file. For example, if the data start from a “ [ ” sign, then the data present is of the JSON array form and you have to use the getJSONArray() function. Following are the functions that can be used for JSON Array:
- get(int index): This function is used to get the value of object type present in the JSON array. If there is no JSON object then you will get a JSONException.
- getInt(int index): This function is used to get the value that is of integer type at a particular index. If not present then you will get JSONException.
- getDouble(int index): This function is used to get the value that is of double type at a particular index. If not present then you will get JSONException.
- getBoolean(int index): This function is used to get the value that is of boolean type at a particular index. If not present then you will get JSONException.
- getLong(int index): This function is used to get the value that is of long type at a particular index. If not present then you will get JSONException.
- getString(int index): This function is used to get the value that is of string type at a particular index. If not present then you will get JSONException.
- getJSONArray(int index): This function is used to get the value that is of array type at a particular index i.e we can have a JSON array in JSON array. If the array is not present then you will get JSONException.
- getJSONObject(int index): This function is used to get the value that is of object type at a particular index i.e we can have a JSON object in JSON array. If the object is not present then you will get JSONException.
- length(): This function is used to get the length of the array that you are getting from the server.
- opt(int index): This method will return the value in the JSONArray at a particular index. The value returned is of Object type. If the value doesn’t exist, then it will return null.
- optInt(int index): It returns the value of type integer at a particular index. If the value is not there, then it will return 0.
- optDouble(int index): It returns the value of type double at a particular index. If the value is not there, then it will return NaN.
- optBoolean(int index): It returns the value of type boolean at a particular index. If the value is not there, then it will return false.
- optLong(int index): It returns the value of type long at a particular index. If the value is not there, then it will return 0.
- optString(int index): It returns the value of type string at a particular index. If the value is not there, then it will return an empty string “”.
- optJSONArray(int index): It will return values of type JSONArray at a particular index. If the array is not there then it will return null.
- optJSONObject(int index): It will return values of type JSONObject at a particular index. If the array is not there then it will return null.
JSON Object Parsing Functions
When the data present in the JSON file is in the form of objects, then you have to use the JSONObject Parsing by using the getJSONObject() function. To find if the data is in Object form or not, just look at the starting of the JSON file, if you find curly braces i.e. “ { ” then the data is in object form. Following are the methods that can be used while working with the JSON object:
- get(int index): This function is used to get the value of object type present in the JSONObject. If there is no JSON object then you will get a JSONException.
- getInt(int index): This function is used to get the value that is of integer type at a particular index. If not present then you will get JSONException.
- getDouble(int index): This function is used to get the value that is of double type at a particular index. If not present then you will get JSONException.
- getBoolean(int index): This function is used to get the value that is of boolean type at a particular index. If not present then you will get JSONException.
- getLong(int index): This function is used to get the value that is of long type at a particular index. If not present then you will get JSONException.
- getString(int index): This function is used to get the value that is of string type at a particular index. If not present then you will get JSONException.
- getJSONArray(int index): This function is used to get the value that is of array type at a particular index i.e. we can have a JSON array in JSONObject. If the array is not present then you will get JSONException.
- getJSONObject(int index): This function is used to get the value that is of object type at a particular index i.e. we can have a JSON object in JSONObject. If the object is not present then you will get JSONException.
- length(): This function is used to find the total number of values present in the JSON file.
- opt(int index): This method will return the value of object type in the JSONObject at a particular index. If the value doesn’t exist, then it will return null.
- optInt(int index): It returns the value of type integer at a particular index. If the value is not there, then it will return 0.
- optDouble(int index): It returns the value of type double at a particular index. If the value is not there, then it will return NaN.
- optBoolean(int index): It returns the value of type boolean at a particular index. If the value is not there, then it will return false.
- optLong(int index): It returns the value of type long at a particular index. If the value is not there, then it will return 0.
- optString(int index): It returns the value of type string at a particular index. If the value is not there, then it will return an empty string “”.
- optJSONArray(int index): It will return values of type JSONArray at a particular index. If the array is not there then it will return null.
- optJSONObject(int index): It will return values of type JSONObject at a particular index. If the array is not there then it will return null.
JSON Parsing Example
We have learned the concept of JSON Parsing and its functions. Now, let’s try to implement the concept in our project.
Create a project in Android Studio by using the Empty Activity template.
After creating the project, our first step is to write the JSON file i.e. in order to work on the JSON file, we must have one JSON file. So, create an Assets folder in the java folder by right-clicking on it and then select new > Folder > Assets Folder . After creating the assets folder, you need to create a JSON file in this folder. So, right click on the Assets folder and then click new > file . Enter the file name of your choice, in my case it is example.json . Following is the content of the example.json file:
{
"users": [
{
"id": "1",
"name": "ABC",
"email": "abc@gmail.com",
"contact": {
"mobile1": "0123456789",
"mobile2":"0123456789",
"mobile3":"0123456789"
}
},
{
"id": "2",
"name": "XYZ",
"email": "xyz@gmail.com",
"contact": {
"mobile1": "0123456789",
"mobile2":"0123456789",
"mobile3":"0123456789"
}
}
]
}
Here, you can find that we are having the data of users. The data includes id, name, email and contact information of the users.
We will fetch the data from this file and will display the data on the RecyclerView.
Note: Here the data is present in the form of JSONObject. So, we will use the getJSONObject() function.
But, before moving to the coding part, let’s make the UI of the application. Here, we will be using the RecyclerView and CardView, so, add the dependencies of these in the app level build.gradle file:
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
After adding the dependencies, go to the activity_main.xml file and add the below code to have a RecyclerView in your application:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
After adding the RecyclerView in the MainActivity, let’s write the code for the items of the RecyclerView. So, create a new layout file in the res/layout folder and name it as recycler_layout.xml . Add the below code for the UI of the Recycler item:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/card_view"
android:layout_width="match_parent"
android:layout_margin="5dp"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="8dp">
<TextView
android:id="@+id/name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="name goes here"
android:textColor="#000000"
android:textSize="16sp" />
<TextView
android:id="@+id/email"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="email goes here"
android:textColor="#000000"
android:textSize="12sp" />
<TextView
android:id="@+id/mobileNo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="mobile goes here"
android:textColor="#000000"
android:textSize="12sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
So, we are done with the UI part. Now, let’s move on to the coding part.
Since we are using a RecyclerView, so, we need to add an Adapter class to fetch the data from the MainActivity and add it to the RecyclerView. So, go to your package and make a class. Add the below code for the Adapter:
class MyAdapter(private var context: Context,
private var personNames: ArrayList<String>,
private var emailIds: ArrayList<String>,
private var mobileNumbers: ArrayList<String>) : RecyclerView.Adapter<CustomAdapter.MyViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): MyViewHolder {
// inflate the Layout with the UI that we have created for the RecyclerView
val v = LayoutInflater.from(parent.context).inflate(R.layout.recycler_layout, parent, false)
return MyViewHolder(v)
}
override fun onBindViewHolder(holder: MyViewHolder, position: Int) {
// set the data in items of the RecyclerView
holder.name.text = personNames[position]
holder.email.text = emailIds[position]
holder.mobileNo.text = mobileNumbers[position]
}
override fun getItemCount(): Int {
//return the item count
return personNames.size
}
inner class MyViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
//connecting od with the text views
internal var name: TextView = itemView.findViewById(R.id.name) as TextView
internal var email: TextView = itemView.findViewById(R.id.email) as TextView
internal var mobileNo: TextView = itemView.findViewById(R.id.mobileNo) as TextView
}
}
Now, the last thing that is to be done is getting the values from the JSON file and then send that value to the MyAdapter to put that values on the RecyclerView. So, go to the MainActivity.kt file and add the below code:
class MainActivity : AppCompatActivity() {
// Creating ArrayList fto store person names, email Id's and mobile numbers
private var personNames = ArrayList<String>()
private var emailIds = ArrayList<String>()
private var mobileNumbers = ArrayList<String>()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the reference of RecyclerView that was created in the MainActivity
val recyclerView = findViewById(R.id.recyclerView) as RecyclerView
// set a LinearLayoutManager with default vertical orientation
val linearLayoutManager = LinearLayoutManager(applicationContext)
recyclerView.layoutManager = linearLayoutManager
try {
// since we have JSON object, so we are getting the object
//here we are calling a function and that function is returning the JSON object
val obj = JSONObject(loadJSONFromAsset())
// fetch JSONArray named users by using getJSONArray
val userArray = obj.getJSONArray("users")
// implement for loop for getting users data i.e. name, email and contact
for (i in 0 until userArray.length()) {
// create a JSONObject for fetching single user data
val userDetail = userArray.getJSONObject(i)
// fetch email and name and store it in arraylist
personNames.add(userDetail.getString("name"))
emailIds.add(userDetail.getString("email"))
// create a object for getting contact data from JSONObject
val contact = userDetail.getJSONObject("contact")
// fetch mobile number 1 and store it in arraylist of mobileNumber
mobileNumbers.add(contact.getString("mobile1"))
}
} catch (e: JSONException) {
//exception
e.printStackTrace()
}
// call the constructor of MyAdapter to send the reference and data to Adapter
val customAdapter = MyAdapter(this@MainActivity, personNames, emailIds, mobileNumbers)
recyclerView.adapter = customAdapter // set the Adapter to RecyclerView
}
private fun loadJSONFromAsset(): String? {
//function to load the JSON from the Asset and return the object
var json: String? = null
val charset: Charset = Charsets.UTF_8
try {
val `is` = assets.open("example.json")
val size = `is`.available()
val buffer = ByteArray(size)
`is`.read(buffer)
`is`.close()
json = String(buffer, charset)
} catch (ex: IOException) {
ex.printStackTrace()
return null
}
return json
}
}
Finally, run your application on the mobile device and try to see the output. Also, change the values in the example.json file and add more data to it.
Conclusion
In this blog, we learned about JSON parsing in Android. We saw what JSON is and how can we use the JSON data in our Android Application. We learned about various functions that are used to handle the JSON format data. At last, we did one example to understand the concepts in a better way. Hope you enjoyed this blog.If you want to learn XML parsing, then you can find the XML parsing blog on MindOrks .
Keep Learning :)
Team MindOrks!