Processing and Parsing XML in Android
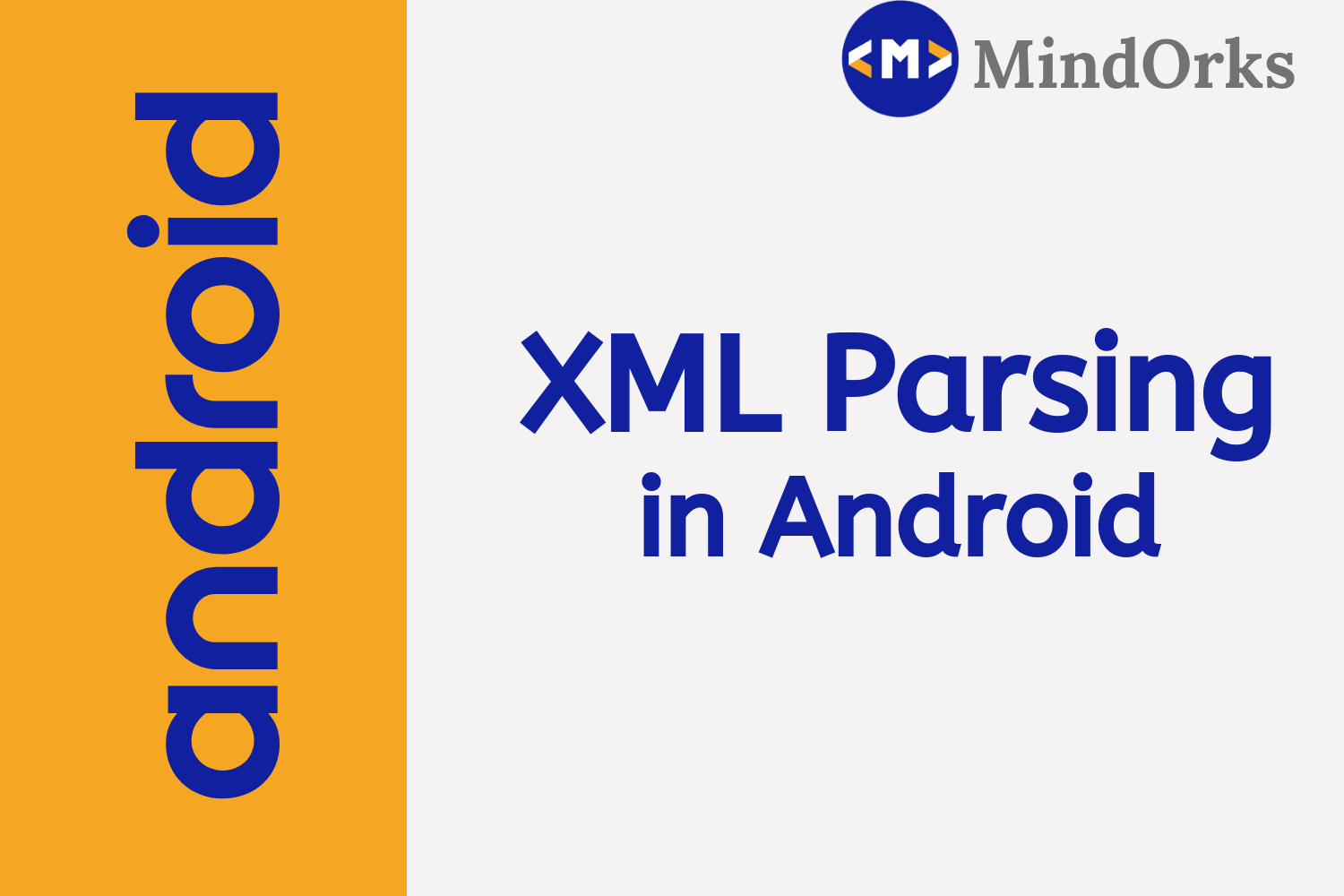
Sharing data over the internet is very popular. We share our information with the other users over the internet. Let’s take a very common example, since we all are developers and to be more precise, we all are Android Developers and we all must have visited the StackOverflow website whenever we are stuck into some problem in our projects and in every 99 out of 100 cases, we find the solution to our problem. The answer that we get from the StackOverflow website consists of various parts that we are less bothered about. In general, the answers or the questions present on the website contains the id of the question, type of the question, author name of the article or question, publishing data, URL and many other things are there on the website. But we want only meaningful data i.e. we want answers and the authors and nothing else.
The problem arises when you want to access the data of a particular website in your Android Application. This is because the data present on the websites are in JSON format or in XML format. So, in order to use that data or to extract the meaningful information from that data, you have to parse that XML file or a JSON file.
So, in this blog, we will learn how to process and parse the XML data in Android. So, let’s get started.
What is XML?
XML stands for Extensible Markup Language. It is a set of rules that are used to encode data or documents in a Machine-readable language. There are many websites that use an XML format to share data on the internet. For example, many blogging websites use an XML format to share data between users. So, XML parsing and processing become an important task to use that data in our Android Application.
XML elements
In general, an XML file consists of a number of elements that together make an XML file. They are the basic building blocks of XML document. These elements are used to store some text elements, attributes, media, etc. Below is the syntax of XML element:
<element-name attributes> Contents...</element-name>
Here, element-name is the name of the element and attributes are used to define the property of XML element. Following is an example of an XML document that is used to describe the data of the student:
<?xml version = “1.0”?>
<contactinfo>
<address category = “college”>
<name>John</name>
<College>MindOrks</College>
<mobile>0123456789</mobile>
</address>
</contactinfo>
We can broadly divide the XML elements in four parts:
- Prolog: It is the first line that contains the information about the XML file i.e. it is the first line of the XML file.
- Events: An XML file contains a number of events like document start, document end, Tag start, Tag end, etc.
- Text: In between the tags(opening and closing), there can be some text. For example, in the above example, “John” is the text.
- Attributes: These are the properties of XML elements.
XML Parsing
So, we have seen the introduction of XML and our next task is, How to parse the XML in Android? So, let’s find out.In Android, there are various parsers that can be used to parse the XML file in Android. Some of these are:
- XMLPullParser
- DOM Parser
- SAX Parser
Among these three parsers, XMLPullParser is the most commonly and most widely used parsers in Android. This is because XMLPullParser is very efficient and easy to use. Also, unlike the DOM Parser which loads the whole file in the memory and then parses it, the XMLPullParser consumes less memory.
Steps involved in XML parsing
Following are the steps that are involved in XML parsing:
- Analyze the feed: There are many cases when you will be provided with a number of data but you need only few of them. SO, before parsing the XML, your first step should be to find the desired element in the XML data. For example, here is example of XML file of StackOverflow:
<?xml version="1.0" encoding="utf-8"?>
<feed xmlns="http://www.w3.org/2005/Atom" xmlns:creativeCommons="http://backend.userland.com/creativeCommonsRssModule" ...">
<title type="text">newest questions tagged android - Stack Overflow</title>
...
<entry>
...
</entry>
<entry>
<id>http://stackoverflow.com/q/9439999</id>
<re:rank scheme="http://stackoverflow.com">0</re:rank>
<title type="text">Where is my data file?</title>
<category scheme="http://stackoverflow.com/feeds/tag?tagnames=android&sort=newest/tags" term="android"/>
<category scheme="http://stackoverflow.com/feeds/tag?tagnames=android&sort=newest/tags" term="file"/>
<author>
<name>cliff2310</name>
<uri>http://stackoverflow.com/users/1128925</uri>
</author>
<link rel="alternate" href="http://stackoverflow.com/questions/9439999/where-is-my-data-file" />
<published>2012-02-25T00:30:54Z</published>
<updated>2012-02-25T00:30:54Z</updated>
<summary type="html">
<p>I have an Application that requires a data file...</p>
</summary>
</entry>
<entry>
...
</entry>
...
</feed>
Here, if you want to extract the data present in the entry tag and the data of its sub tags then you should keep note of these things.
- Create XMLPullParser object: Our next step is to create the object of XMLPullParser and specify the file name for the XMLPullParser that contains XML. You can use a file or a stream. Following is the code to create an object of the XMLPullParser and then passing the file name for the XMLPullParser:
class StackOverflowXmlParser {
@Throws(XmlPullParserException::class, IOException::class)
fun parse(inputStream: InputStream): List<*> {
inputStream.use { inputStream ->
val parser: XmlPullParser = Xml.newPullParser()
parser.setFeature(XmlPullParser.FEATURE_PROCESS_NAMESPACES, false)
parser.setInput(inputStream, null)
parser.nextTag()
return readFeed(parser)
}
}
...
}
- Read the desired data: Our last step is to parse the data of the XML file. The following readFeed() function is used to parse the XML file and extract only the values of entries and store it into a list:
@Throws(XmlPullParserException::class, IOException::class)
private fun readFeed(parser: XmlPullParser): List<Entry> {
val entries = mutableListOf<Entry>()
parser.require(XmlPullParser.START_TAG, ns, "feed")
while (parser.next() != XmlPullParser.END_TAG) {
if (parser.eventType != XmlPullParser.START_TAG) {
continue
}
// Starts by looking for the entry tag
if (parser.name == "entry") {
entries.add(readEntry(parser))
} else {
skip(parser)
}
}
return entries
}
Here, XMLPullParser.END_TAG is used to find if the parser has reached to the last tag of the file or not. If it has reached then return and it has not reached then we will check for the entry tag and if found then will add the value to the entries list. If the values are not found be to of entries type then we can skip the parser. At last, we return entries.
Following are the methods that can be used while parsing an XML file:
- getEventType(): This method is used to get the event type. For example, Document start, Document End, Tag start, Tag end, etc.
- getName(): This is used to get the tag name in a file. For example, in the above code, the tag name is entry .
- getAttributeValue(): This method is used to get the attribute value of a particular tag.
- getAttributeCount(): It returns the total number of attributes of the current tag.
- getAttributeName(int index): It returns the attribute name at a particular index.
- getColumnNumber(): It returns the current column number using a 0 based indexing.
- getName(): It returns the name of the tag.
- getText(): It returns the text present in a particular element.
XML parsing example
Now, let’s do one example to understand the concept of XML parsing ina very easy and clear manner.
Open Android Studio and create a project with Empty Activity template.
The very first step is to create the XML file. Either you create your own XML file or you can use mine. To create an XML file, go to java folder and then right click on it. Then click on New > Folder > Assets folder and then OK. One assets folder will be created for you. Now right click on that folder and create a file and name it as example.xml . Following is the XML content of example.xml file:
<?xml version="1.0"?>
<records>
<student>
<name>ABC</name>
<surname>ABC</surname>
<mobile>0123456789</mobile>
<section>A</section>
</student>
<student>
<name>XYZ</name>
<surname>XYZ</surname>
<mobile>0123456789</mobile>
<section>B</section>
</student>
<student>
<name>PQR</name>
<surname>PQR</surname>
<mobile>0123456789</mobile>
<section>C</section>
</student>
</records>
This is a simple example of student data containing the name, surname, mobile and section of the student. In this example, we will extract the name, surname and section of the student. We will ignore the mobile number.
Now, let’s code for the UI part of our application. Here we will display the desired data on a TextView. So, open the activity_main.xml file and add the below code:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
So, we are done with the UI part. Now, let’s write the code for the XML parsing. Below is the code for the MainActivity.kt file:
class MainActivity : AppCompatActivity() {
private lateinit var txtview: TextView
@SuppressLint("SetTextI18n")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
txtview = findViewById<TextView>(R.id.textView)
try {
val inputStream = assets.open("example.xml")
val dbFactory = DocumentBuilderFactory.newInstance()
val dBuilder = dbFactory.newDocumentBuilder()
val doc = dBuilder.parse(inputStream)
val element = doc.documentElement
element.normalize()
val nList = doc.getElementsByTagName("student")
for (i in 0 until nList.length) {
val node = nList.item(i)
if (node.nodeType === Node.ELEMENT_NODE) {
val element2 = node as Element
txtview.text = "" + txtview.text + "\nName : " + getValue("name", element2) + "\n"
txtview.text = "" + txtview.text + "Surname : " + getValue("surname", element2) + "\n"
txtview.text = "" + txtview.text + "Section : " + getValue("section", element2) + "\n"
txtview.text = "" + txtview.text + "-----------------------"
}
}
} catch (e: Exception) {
e.printStackTrace()
}
}
private fun getValue(tag: String, element: Element): String {
val nodeList = element.getElementsByTagName(tag).item(0).childNodes
val node = nodeList.item(0)
return node.nodeValue
}
}
Now, run the application on your mobile phone and try to add more data to your XML file i.e. the example.xml file.
Conclusion
In this blog, we learned about XML parsing in Android. We learned how to parse the data present in the XML format in Android Application. We saw how to use XMLPullParser to do XML parsing. At last, we did one example of XML parsing. You can get more contents about XML parsing at the Android Developer Website .
Keep Learning :)
Team MindOrks!