Animation in Android - Android Tutorial
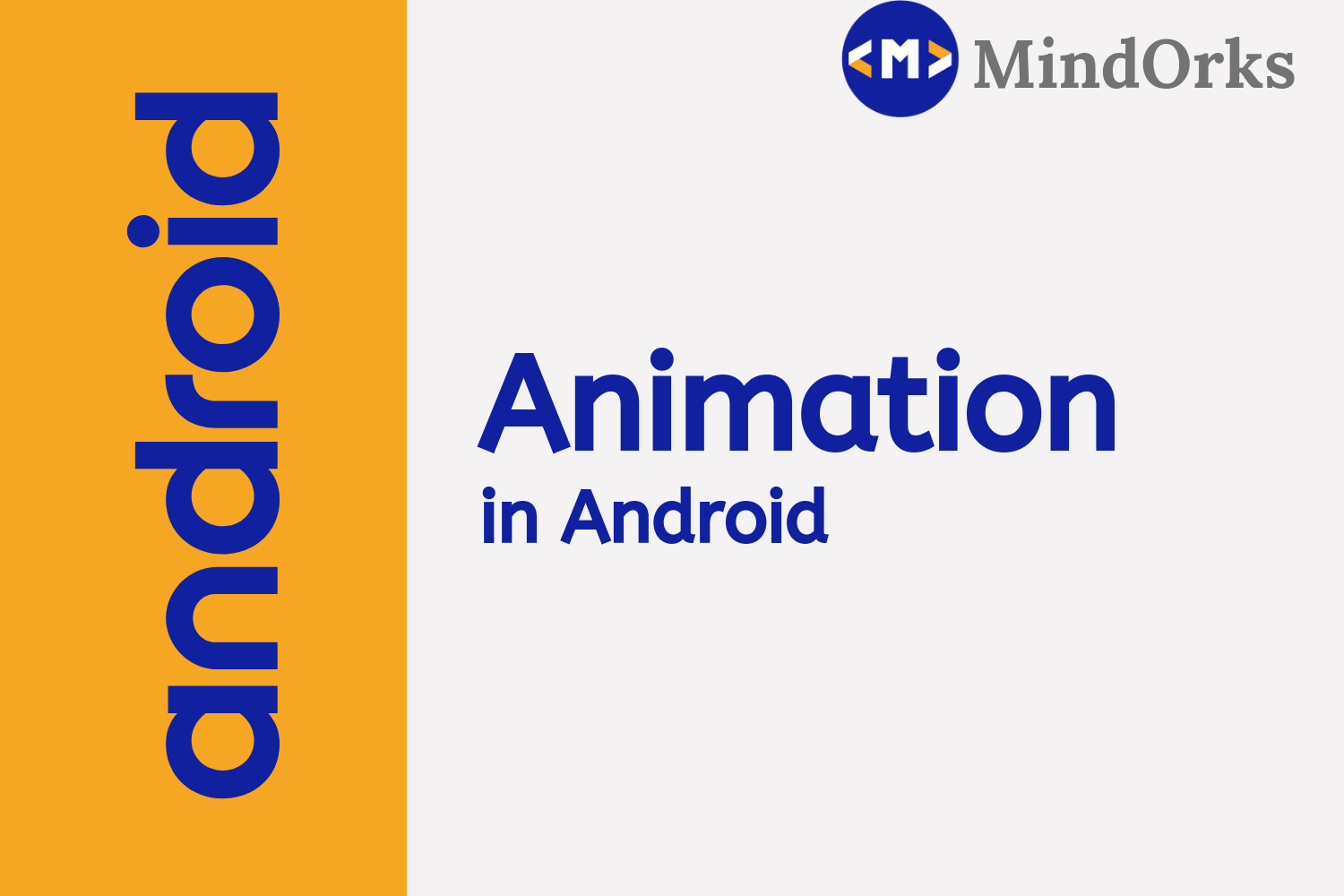
A picture is worth more than 1000 words. If you are using some icons or pictures or animations in your Android application then your users will interact with your application in a better way and things become easier to understand when you are using some pictorial representation rather than some textual representation. Nowadays, every big application is using animations to represent the content in a more understandable way. And when the Material Design concept came in existence, then these Animations also came into existence. For example, the moving car icon in the Uber app or the login screen of the Zomato app are some cool examples of animations in Android application. Here is a basic example of animation user in the play button:
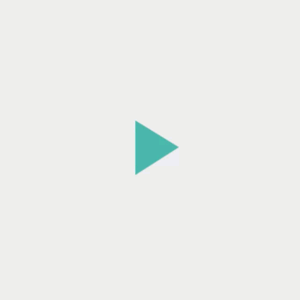
No doubt, we can use the feature of the play button without using animation also, but if you use animation then it looks more interactive i.e. by using animation, we are not abruptly changing the UI of the application.
So, in this blog, we will learn how we can implement Animations in Android. So, let’s get started.
What is Animation?
If we look at the definition of Animation from Wikipedia, then it says something like this:
Animation is a method in which pictures are manipulated to appear as moving images.
So, we can think of animations as moving images. For example, if you want to do some UI change in your application and if you change the UI directly then it will look very ugly. Look at the below image taken from the Android Developer Website:
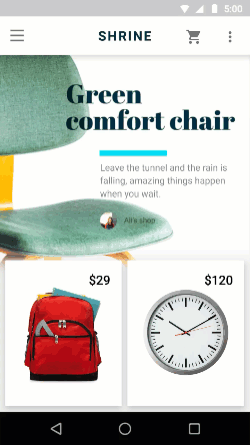
Here, if you are clicking on the Clock item, then you are opening a new Activity but if you open the activity directly then a sudden change in UI will be imposed and this may lead to bad user experience. So, by using animation, you can change the UI by having some cool layout transition animation.
For a better understanding of Animation, I have divided the whole blog into three categories:
- Basic Animation
- Animate Drawable Graphics
- Animation between Activities
So, let’s learn these, one by one.
Basic Animation using XML
The best part about animation that I like is the fact that you can use animation just by writing some XML code. Yeah and this is the need because in Animation, you are dealing with the UI part and in order to have Animation, XML codes can be used.
In order to have a thorough knowledge of Animation, you should know some of the important XML attributes that can be used in your application to have a perfect Animation. Some of these attributes are:
- android:duration: It is used to specify the duration of animation i.e. how long a particular Animation will continue.
- android:interpolator: It is used to define the rate of change of Animation i.e. how fast a particular UI will change to another UI.
- android:startOffset: When you are having a number of animations one after the other, then you have to specify some time to a particular animation and that animation will wait for that duration and after that, it will start.
- android:repeatMode: This is used to repeat a particular animation.
- android:repeatCount: This is used to specify the repeat count of a particular animation. You can set the repeat count of an animation to be infinite , if you want infinite repetition.
Now, we are done with the introduction part. Let’s implement some basic animations in our project. Follow the best steps:
Step 1: Open Android Studio and create a new project with Empty Activity template.
Step 2: After creating the project, the very first thing that you have to do is making an anim resource directory where you can store all your animation files. So, go to res directory and right click on it, then click on New > Android Resource Directory and after that choose resource type as anim and then click on OK .
Step 3: Now, you have to create some Animation files in the anim folder that you have created in the previous step. So, right click on res/anim and select New > Animation Resource File and then enter the file name and click on OK . Here, we will see how to use the Fade In animation. So, my file name is fade_in.xml .
Step 4: Our next step is to add the animation that we want to perform in the file that we have added in the previous step. Add the below code for Fade In animation in your fade_in.xml file:
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true">
<alpha
android:duration="5000"
android:fromAlpha="0.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="1.0" />
</set>
Step 5: Add the UI for the MainActivity. Here we will add the desired UI of the MainActivity. Here, I am adding one TextView (to apply animation on) and one Button(to start the animation). So, the code for the activity_main.xml file looks like:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintTop_toBottomOf="@+id/text"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginEnd="8dp" android:layout_marginRight="8dp"
app:layout_constraintStart_toStartOf="parent"
android:layout_marginLeft="8dp"
android:layout_marginStart="8dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toBottomOf="parent"
android:text="Click Me"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Step 6: After adding the UI, our last step is to connect the animation when the Button is clicked. So, the code of MainActivty.kt file looks like:
class MainActivity : AppCompatActivity() {
//TextView
lateinit var txtMessage: TextView
//Button
lateinit var btn: Button
// Animation
lateinit var animFadein: Animation
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
txtMessage = findViewById<TextView>(R.id.text)
btn = findViewById<Button>(R.id.btn)
//loading Animation
animFadein = AnimationUtils.loadAnimation(
applicationContext,
R.anim.fade_in)
//handling aniamtion on button click
btn.setOnClickListener {
txtMessage.setVisibility(View.VISIBLE)
// starting the animation
txtMessage.startAnimation(animFadein)
}
}
}
loadAnimation() method is used to load the desired animation i.e. in our case, it is fade_in.xml file.
startAnimation() method is used to start an animation on a particular component.If you want to perform some operations before, after or during the Animation, then you can implement the AnimationListener in your call and then override the below function for the same:
- onAnimationStart(): Will be called when the Animation will start.
- onAnimationEnd(): Will be called when the Animation will end.
- onAnimationRepeat(): Will be called when you repeat an Animation.
All you need to do is implement the AnimationListener and then set the animation listener. Our final code after implementing the AnimationListener will be:
class MainActivity : AppCompatActivity(), Animation.AnimationListener {
//TextView
lateinit var txtMessage: TextView
//Button
lateinit var btn: Button
// Animation
lateinit var animFadein: Animation
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
txtMessage = findViewById<TextView>(R.id.text)
btn = findViewById<Button>(R.id.btn)
//loading Animation
animFadein = AnimationUtils.loadAnimation(
applicationContext,
R.anim.fade_in)
//set animation listener
animFadein.setAnimationListener(this)
//handling aniamtion on button click
btn.setOnClickListener {
txtMessage.setVisibility(View.VISIBLE)
// starting the animation
txtMessage.startAnimation(animFadein)
}
}
override fun onAnimationRepeat(p0: Animation?) {
//To change body of created functions use File | Settings | File Templates.
}
override fun onAnimationEnd(p0: Animation?) {
//To change body of created functions use File | Settings | File Templates.
}
override fun onAnimationStart(p0: Animation?) {
//To change body of created functions use File | Settings | File Templates.
}
}
Run your application on mobile device and see the Animation.
In the next part of this section, we will look upon some basic animations XML file. You can use these animations in the same manner as used in the above example. Add the below files in your anim folder.
Animation for Fade Out
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<alpha
android:duration="5000"
android:fromAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:toAlpha="0.0" />
</set>
Animation for ClockWise rotation
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:duration="5000" >
</rotate>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:startOffset="5000"
android:fromDegrees="360"
android:toDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="5000" >
</rotate>
</set>
Animation for Blink
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<alpha android:fromAlpha="0.0"
android:toAlpha="1.0"
android:interpolator="@android:anim/accelerate_interpolator"
android:duration="600"
android:repeatMode="reverse"
android:repeatCount="infinite"/>
</set>
Animation for Zoom In
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:fromXScale="1"
android:fromYScale="1"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="3"
android:toYScale="3" >
</scale>
</set>
Animation for Zoom out
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true" >
<scale
xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:pivotX="50%"
android:pivotY="50%"
android:toXScale="0.5"
android:toYScale="0.5" >
</scale>
</set>
Animation for Move To move one component form one position to other
<set
xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/linear_interpolator"
android:fillAfter="true">
<translate
android:fromXDelta="0%p"
android:toXDelta="75%p"
android:duration="800" />
</set>
Animation for Bounce
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true"
android:interpolator="@android:anim/bounce_interpolator">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="0.0"
android:toXScale="1.0"
android:toYScale="1.0" />
</set>
Animate Drawable Graphics
Apart from the basic Animation, these days, animations are used in Drawable resources i.e. Animations are being used in the Vector assets or Image Assets. Below is an example of the animation used in Drawable resources:
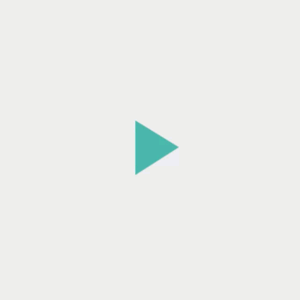
Here, the animation is applied to the Play Button icon.There are two ways of achieving this:
- Use AnimationDrawable
- Use AnimatedVectorDrawable
Use AnimationDrawable
One of the basic ways of animation is to play or put one image after the other. So, in this case, we are having a series of images that are coming one after the other. For example, if you are having five images i.e. Image1, Image2, Image3, Image4, and Image5 then play one image after the other.
To achieve this feature, you can use the AnimationDrawable class API. Here, the XML file for this is stored in the res/drawable directory and the XML file consists of a <animation-list> which contains a series of <items> that will be played or put one after the other.
Below is an example of the XML file which is added in res/drawable :
<animation-list xmlns:android="http://schemas.android.com/apk/res/android"
android:oneshot="true">
<item android:drawable="@drawable/rocket_thrust1" android:duration="200" />
<item android:drawable="@drawable/rocket_thrust2" android:duration="200" />
<item android:drawable="@drawable/rocket_thrust3" android:duration="200" />
</animation-list>
Here, android:oneshot is used to tell if the list is to be repeated or not. If the value is true then after the animation of the last element, the whole animation will be stopped. But if the value is set to false , then the animation will continue again and again.
android:drawable is used to identify the element that is to be animated i.e. these are elements that will be showed when we start the animation. The rocket_thrust1 will be showed and after that reocket_thrust2 will be showed and finally, rocket_thrust3 will be shown. All the animation will be showed in a duration that is written in the android:duration i.e. 200. By doing so, you will get a visualization of animation.
Below is the code for our MainActivity.kt file to apply the above animation on an ImageView. Here, we are using AnimationDrawable :
private lateinit var rocketAnimation: AnimationDrawable
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.main)
val rocketImage = findViewById<ImageView>(R.id.rocket_image).apply {
setBackgroundResource(R.drawable.rocket_thrust)
rocketAnimation = background as AnimationDrawable
}
rocketImage.setOnClickListener({ rocketAnimation.start() })
}
UseAnimatedVectorDrawable
You can apply animation to your VectorDrawable by using the AnimatedVectorDrawable . So, basically add some Vector Assets in your project and use animationon it.
To achieve this, you have to add three XML files in your project:
- A vector drawable in the res/drawable directory. You can create a vector drawable by right-clicking on res/drawable and then New > Drawable resource file and enter the desired name
<!-- res/drawable/vectordrawable.xml -->
<!-- Source: Android Developer Website -->
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:height="64dp"
android:width="64dp"
android:viewportHeight="600"
android:viewportWidth="600">
<group
android:name="rotationGroup"
android:pivotX="300.0"
android:pivotY="300.0"
android:rotation="45.0" >
<path
android:name="v"
android:fillColor="#000000"
android:pathData="M300,70 l 0,-70 70,70 0,0 -70,70z" />
</group>
</vector>
Other way of creating vector assets in your res/drawable directory. Just right click on the res directory and click on New > Vector assets and then follow the steps.
- An animated vector drawable that is used to animate the attributes of the vector drawable i.e. <group> , <item> , etc. The main aim of this file is to apply certain animation to a certain component. For example, if you are having an attribute A in your vector drawable file and your want some Animation anim to be applied on the attribute A, then you define these things in your animated vector drawable present in the res/drawable directory.
<!-- res/drawable/animvectordrawable.xml -->
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/vectordrawable" >
<target
android:name="rotationGroup"
android:animation="@anim/rotation" />
<target
android:name="v"
android:animation="@anim/path_morph" />
</animated-vector>
At last, you have to add the Object Animator in your res/animator directory. Here, you actually define the animation to be applied on any component. To create an Animator directory go to res and right click on it. After that click on New and Android Resource Directory and select the resource type as animator and click on OK . After that go to res/animator and add the resource file of the name of your choice. Following is an example of the post:
<!-- res/animator/rotation.xml -->
<!-- Source: Android Developer Website -->
<objectAnimator
android:duration="6000"
android:propertyName="rotation"
android:valueFrom="0"
android:valueTo="360" />
Animation between Activities
When we are making an Android Application, then we deal with various Activities and one Activity is started after the other with the help of some triggers. But if you directly open an activity from another activity then there will be a sudden change in UI and this may result in bad user experience. So, we should apply some kind of Animation that can be used to have a fluent transition from one activity to the other. You can achieve this by enabling Activity Transitions in your application. You can apply animation to your transition in the application:
Enter Transition: An enter transition is used to define the animation that is used when a view of the activity is entering a screen.
Exit Transition: An exit transition is used to define the animation that is used when a view of the activity is exiting the screen.
Following are the entry and exit transition supported by Android:
- explode: It is used to move the view in and out in the screen from the center of the screen.
- slide: It is used to slide the view between edges in the screen of the application.
- fade: It is used to change the opacity of the view while entering into the screen.
Following is an example of Screen Transition used in Android Application:
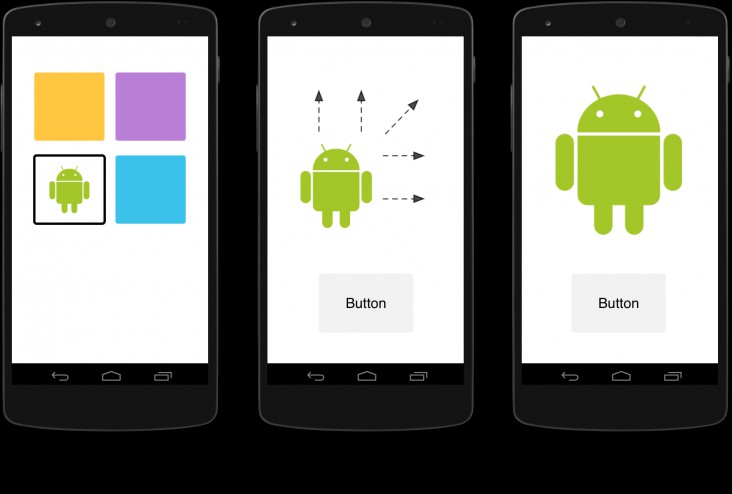
Here, when you click on the Android icon, then a new Activity will be opened by using Activity Transition.
Now, let’s start the coding part.
The Activity transition API is available for the API level 21 or higher. So, our first step should be to check for the version of API being used:
// Check if we're running on Android 5.0 or higher
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
// Apply activity transition
} else {
// Swap without transition
}
After checking for the Android Version, our next step is to add the entry and exit transition for the activity using windowActivityTransitions
<style name="BaseAppTheme" parent="android:Theme.Material">
<!-- enable window content transitions -->
<item name="android:windowActivityTransitions">true</item>
<!-- specify enter and exit transitions -->
<item name="android:windowEnterTransition">@transition/explode</item>
<item name="android:windowExitTransition">@transition/explode</item>
<!-- specify shared element transitions -->
<item name="android:windowSharedElementEnterTransition">
<transitionSet xmlns:android="http://schemas.android.com/apk/res/android">
<changeImageTransform/>
</transitionSet></item>
<item name="android:windowSharedElementExitTransition">
<transitionSet xmlns:android="http://schemas.android.com/apk/res/android">
<changeImageTransform/>
</transitionSet></item>
</style>
Our last step is to start the Activity by enabling transition:
startActivity(intent,
ActivityOptions.makeSceneTransitionAnimation(this).toBundle())
Phew! Such a long blog. But I am sure, you learned a lot from this blog.
Conclusion
In this blog, we learned about Animations in Android. We learned how we can add some basic Animation form in our Android Application. Adding animation to our application results in more user interaction and making the application more attractive. So, having some kind of animation in our application is good to have practice.
You can understand the details of Motion in Android by referring to the Material Design website .
Also, there are a lot of libraries that can be used to implement some cool animations. You can find the top animation libraries here .
Keep Reading:)
Team MindOrks!