How to programmatically take a screenshot on Android?
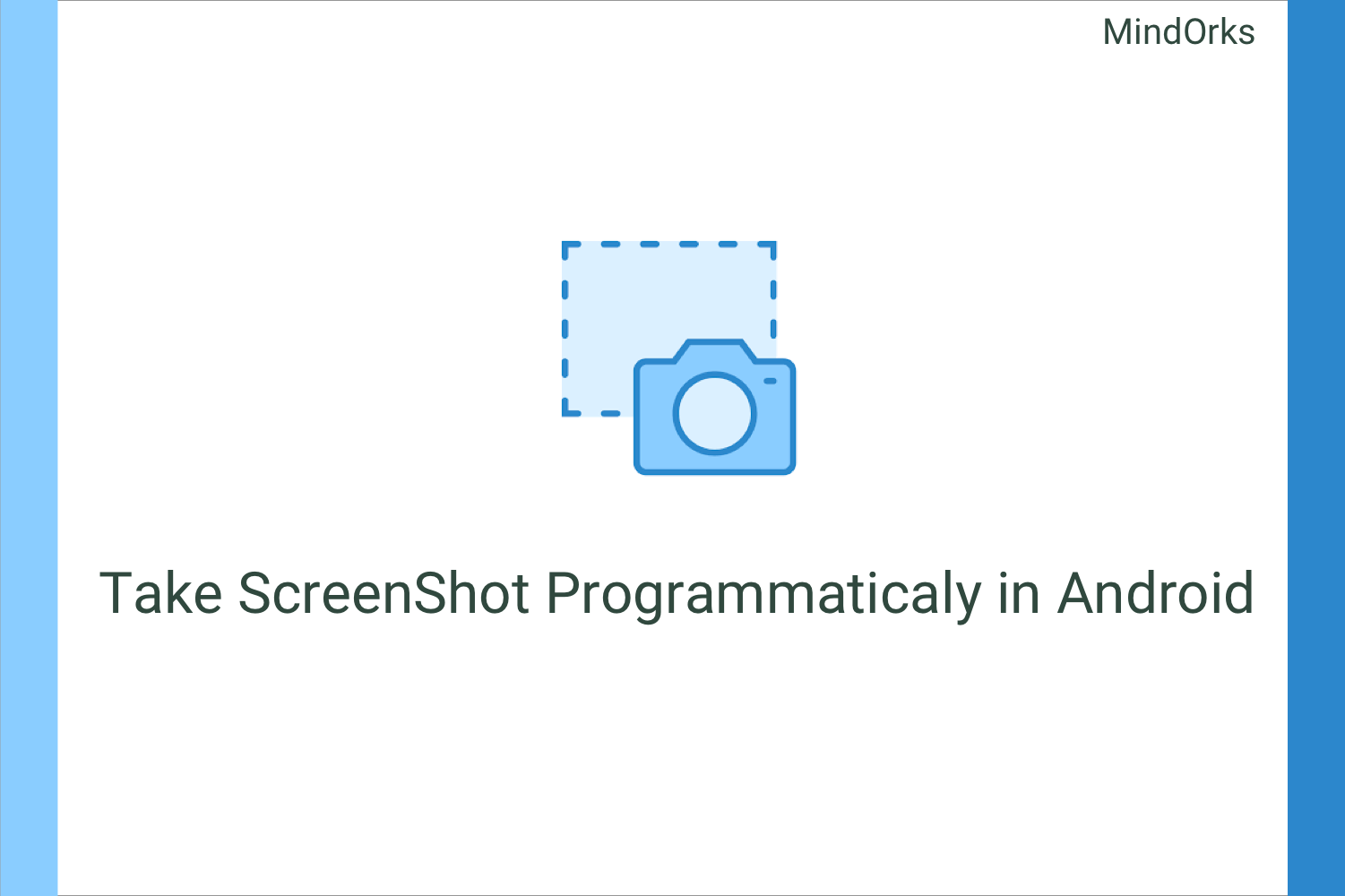
In this blog, we will learn to take screenshot of any view particular view or any layout programmaticaly.
But first we will create a layout which we want to take screenshot of,
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/container"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="I am screenshot"
android:id="@+id/textView"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
</android.support.constraint.ConstraintLayout>
and in the activity, we will create a function called
getScreenShot(/** your view **/)
Here, view is the layout view which we want to take screenshot. In our code, we have view with id as container.
Our getScreenShot method will generate a bitmap and we can perform actions on it
private fun getScreenShot(view: View): Bitmap {
val returnedBitmap = Bitmap.createBitmap(view.width, view.height, Bitmap.Config.ARGB_8888)
val canvas = Canvas(returnedBitmap)
val bgDrawable = view.background
if (bgDrawable != null) bgDrawable.draw(canvas)
else canvas.drawColor(Color.WHITE)
view.draw(canvas)
return returnedBitmap
}
Here, view we will take the id of ConstraintLayout (i.e. container ). In this method, we will first create a empty bitmap which we have to return as value for the function. Then we construct a Canvas which takes the bitmap to draw onto it. In bgDrawable, it takes the background of the view. And now, we will draw the view on canvas using view.draw(canvas). Finally, we return the bitmap we have create as it will return the Bitmap of the view.
Now, in the Activtiy file to call the above function like the following,
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val constraintLayout: ConstraintLayout = findViewById(R.id.container)
getScreenShot(constraintLayout)
}
private fun getScreenShot(view: View): Bitmap {
val returnedBitmap = Bitmap.createBitmap(view.width, view.height, Bitmap.Config.ARGB_8888)
val canvas = Canvas(returnedBitmap)
val bgDrawable = view.background
if (bgDrawable != null) bgDrawable.draw(canvas)
else canvas.drawColor(Color.WHITE)
view.draw(canvas)
return returnedBitmap
}
}
and it will generate output as,
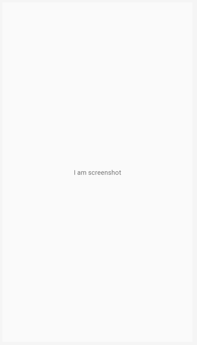
Now, to generate the Bitmap of the complete Activity with the toolbar as well, we call the function getScreenShot() like,
val activityView = window.decorView.rootView
getScreenShot(activityView)
THe above code will generate the following output,
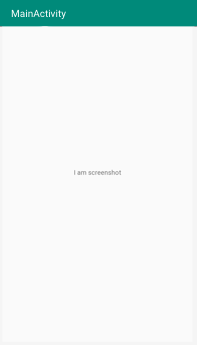
Now, you can perform multiple actions with the bitmap which got generated. You can either save it to local storage in your device or can perform action like edit, etc.
This is useful to generate bitmaps for any view you want, you can also generate screenshot of any particular widget like ImageView or TextView.
Happy learning
Team MindOrks :)