Android ViewPager in Kotlin
Welcome! Welcome!! , Hope you had fun learning about fragments!Here, we are going to use the fragments and develop something which you can be proud of, which you had seen in most of the apps, which also looks interesting and make you feel connected with the application.
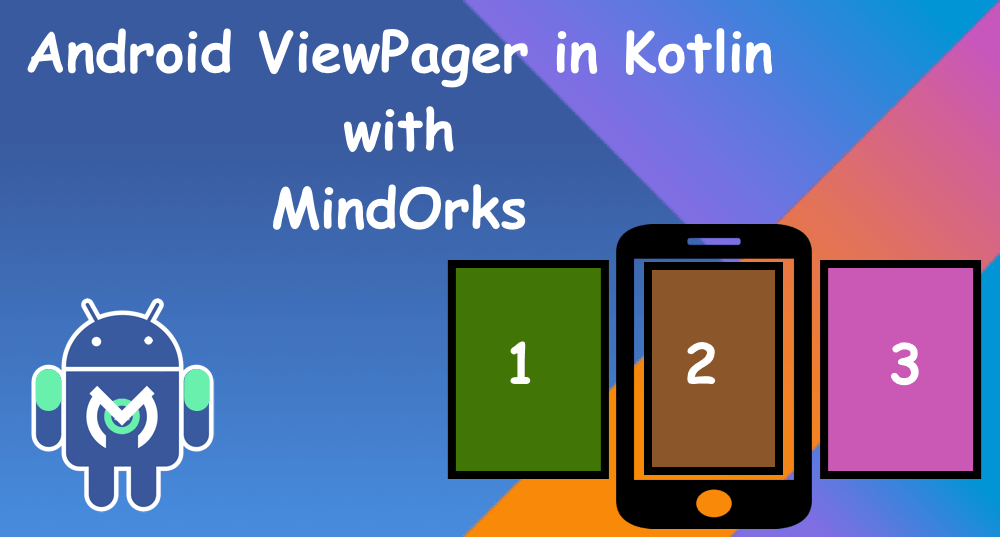
MINDORKS INTRO
Yes, we are going to create an app which will have a colourful introduction slider. To develop this app, you need to complete our previous chapters where you can learn about fragments and activities.
Let’s start with the development.
- Create a new project. We are giving “MindOrks Intro” as the name of our app. Also, setup Constraint Layout as we did in the earlier chapters if not configured.
-
Let’s first talk about the colours. We will require four different colours for the background of 4 different fragments.
<! — Screens background color →
<array name=”bg_color”>
<item name=”bg_screen1">#f64c73</item>
<item name=”bg_screen2">#20d2bb</item>
<item name=”bg_screen3">#3395ff</item>
<item name=”bg_screen4">#c873f4</item>
</array>
3. Let’s create a layout for the fragment.
<?xml version=”1.0" encoding=”utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android=”http://schemas.android.com/apk/res/android"
xmlns:app=”http://schemas.android.com/apk/res-auto"
android:layout_width=”match_parent”
android:layout_height=”match_parent”><TextView
android:id=”@+id/tvTitle”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
app:layout_constraintBottom_toBottomOf=”parent”
app:layout_constraintEnd_toEndOf=”parent”
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toTopOf=”parent”/></androidx.constraintlayout.widget.ConstraintLayout>
4. Now, we need to create a fragment class and bind this fragment layout with it. We are creating a IntroFragment class here and in onCreate() method we are binding the layout with this class.
class IntroFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?) =
inflater.inflate(R.layout.fragment_intro_screen, container, false)!!
}
5. We need a adapter class which will do most of our work for the ViewPager like counting how many fragments to attach, creating the instance of those framgments etc.
6. We are creating an
IntroViewPagerdapter
class here and implementing the FragmentStatePagerAdapter class. It will require to override two methods.
class IntroViewPagerAdapter(supportFragmentManager: FragmentManager) : FragmentStatePagerAdapter(supportFragmentManager) { override fun getItem(position: Int): Fragment {} override fun getCount(): Int {
return 4
}
}
We had return the 4 in getCount method as we need four fragments to attach.
7. Now, we will be creating a method in fragment class which will return us its instance with data in it as arguments.
companion object {
fun newInstance(title: String): Fragment {
val fragment = IntroFragment()
val args = Bundle()
args.putString(“title”, title)
fragment.arguments = args
return fragment
}}
8. In our onActivityCreate() method we can retrieve this data and set to our textView.
override fun onActivityCreated(savedInstanceState: Bundle?) {
super.onActivityCreated(savedInstanceState)
tvTitle.text = arguments!!.getString(“title”)
}
9. From our adapter class we need to call newInstance method in order to get the fragment.
override fun getItem(position: Int): Fragment {
return IntroFragment.newInstance(“Title” + position)
}
10. Now, we need some view which can hold our framgents in main screen layout. We will be using ViewPager.
<androidx.viewpager.widget.ViewPager
android:id=”@+id/vpIntro”
android:layout_width=”0dp”
android:layout_height=”0dp”
app:layout_constraintBottom_toBottomOf=”parent”
app:layout_constraintEnd_toEndOf=”parent”
app:layout_constraintStart_toStartOf=”parent”
app:layout_constraintTop_toTopOf=”parent”/>
11. Now, wire everything with our ViewPager which is on the main screen. Creating the adapter object and set to the viewPager.
var introViewPagerAdapter = IntroViewPagerAdapter(supportFragmentManager)
vpIntro.adapter = introViewPagerAdapter
12. Lastly, we need to change color when the fragment changes. We need to get the color array from colors.xml and check which fragment is visible and then update the viewPager background color.
val bg_color = resources.getIntArray(R.array.bg_color) vpIntro.addOnPageChangeListener(object: ViewPager.OnPageChangeListener{
override fun onPageScrollStateChanged(state: Int) {}override fun onPageSelected(position: Int) {}override fun onPageScrolled(position: Int, positionOffset: Float, positionOffsetPixels: Int) {
vpIntro.setBackgroundColor(bg_color[position])
}
})
Try run this application on your device and swipe left and right. You will the change of the text and background color. This is just the intro about how the fragment works and how the ViewPager is helpful view to make a list of fragments.
Explore more on this and share with us on our twitter and slack channel.