Android SharedPreferences in Kotlin
Welcome, here we are going to understand what SharedPreferences is. In Android, there are many ways to store and play with the data. SharedPreferences is one of the ways where you can store some data in the key-value pair.
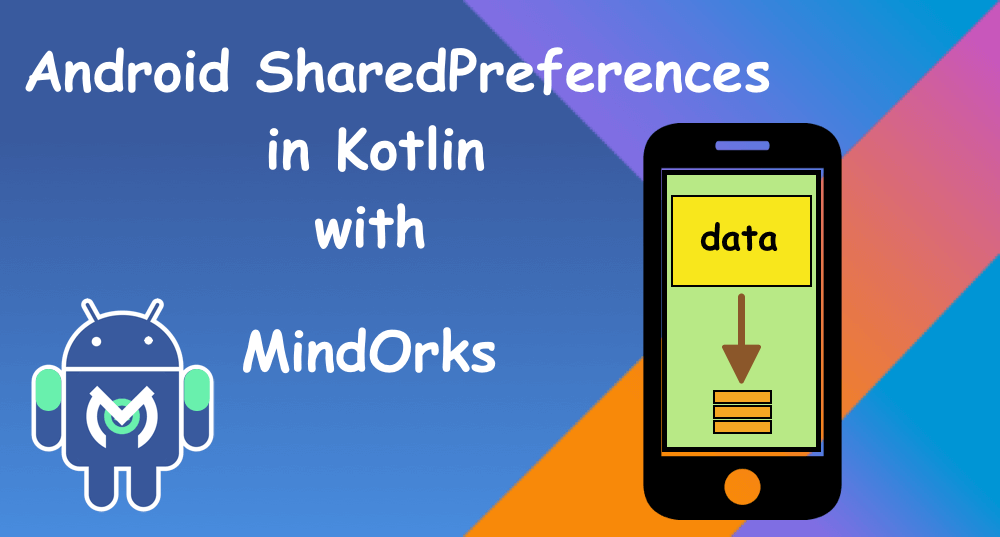
We are going to use our previously build MindOrks Intro application where we had developed an application in which four swipeable slides comes.
But, you have seen that those are the intro slides which only comes one time when you first time open your app, after that, you never see the slides.
How can we check this and tell our application that user had seen these slides once and now, never to open and directly go inside the app?
We need to use SharedPreferences when the first time user sees the intro slides we will save a true variable in it, and after that, whenever a user opens our application, we will check that shared pref variable is true or false for further actions.
Let’s start the development:Open MindOrks Intro project or get it from the GitHub.
Open the MainActivity which is our launcher activity which first executes when the app opens.
We need to create a shared pref instance, so call getSharedPreferences() method, this will point to the file where our variable gets stored.
private var PRIVATE_MODE = 0
private val PREF_NAME = "mindorks-welcome"
val sharedPref: SharedPreferences = getSharedPreferences(PREF_NAME, PRIVATE_MODE)
We need to pass two things:First, the key to the variable and Second, the mode, PRIVATE_MODE. Private mode will allow this variable to on accessed by this application.
Now, we will create the logic to check if the user had seen the slides or not.
if (sharedPref.getBoolean(PREF_NAME, false)) {
} else {
}
Here, we use the SharedPreferences object to get a boolean variable of key PREF_NAME. I
f we didn’t create this variable till now, by default, this would pass false to us, as we had mentioned. So, inside if we need to open our HomeActivity.
We had created Home Activity to open if the slides had seen by the user. And in else, we will set the layout of the slides and initiate viewPager.
Also, we need to put true in the SharedPreferences variable PREF_NAME, to not allowing run slides in the next time.
if (sharedPref.getBoolean(PREF_NAME, false)) {
val homeIntent = Intent(this, HomeActivity::class.java)
startActivity(homeIntent)
finish()
} else {
setContentView(R.layout.activity_main)
setViewPager()
val editor = sharedPref.edit()
editor.putBoolean(PREF_NAME, true)
editor.apply()
}
To write data in SharedPref, we use sharedPref edit instance. After writing the data, we need to call apply() method to affect the changes.
class MainActivity : AppCompatActivity() {
private var PRIVATE_MODE = 0
private val PREF_NAME = "mindorks-welcome"
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val sharedPref: SharedPreferences = getSharedPreferences(PREF_NAME, PRIVATE_MODE)
if (sharedPref.getBoolean(PREF_NAME, false)) {
val homeIntent = Intent(this, HomeActivity::class.java)
startActivity(homeIntent)
finish()
} else {
setContentView(R.layout.activity_main)
setViewPager()
val editor = sharedPref.edit()
editor.putBoolean(PREF_NAME, true)
editor.apply()
}
}
private fun setViewPager() {
val introViewPagerAdapter = IntroViewPagerAdapter(supportFragmentManager)
vpIntro.adapter = introViewPagerAdapter
val bg_color = resources.getIntArray(R.array.bg_color)
vpIntro.addOnPageChangeListener(object : ViewPager.OnPageChangeListener {
override fun onPageScrollStateChanged(state: Int) {
}
override fun onPageSelected(position: Int) {
}
override fun onPageScrolled(position: Int, positionOffset: Float, positionOffsetPixels: Int) {
vpIntro.setBackgroundColor(bg_color[position])
}
})
}
}
Let’s run this application; you will see the intro slides and the next time you open the app, you will directly go to the HomeActivity.
To view the intro slides, you need to reinstall the application as it will delete the shared pref data of our app. Try to explore more and share us with on our twitter or slack channel.