LiveData vs ObservableField in Android
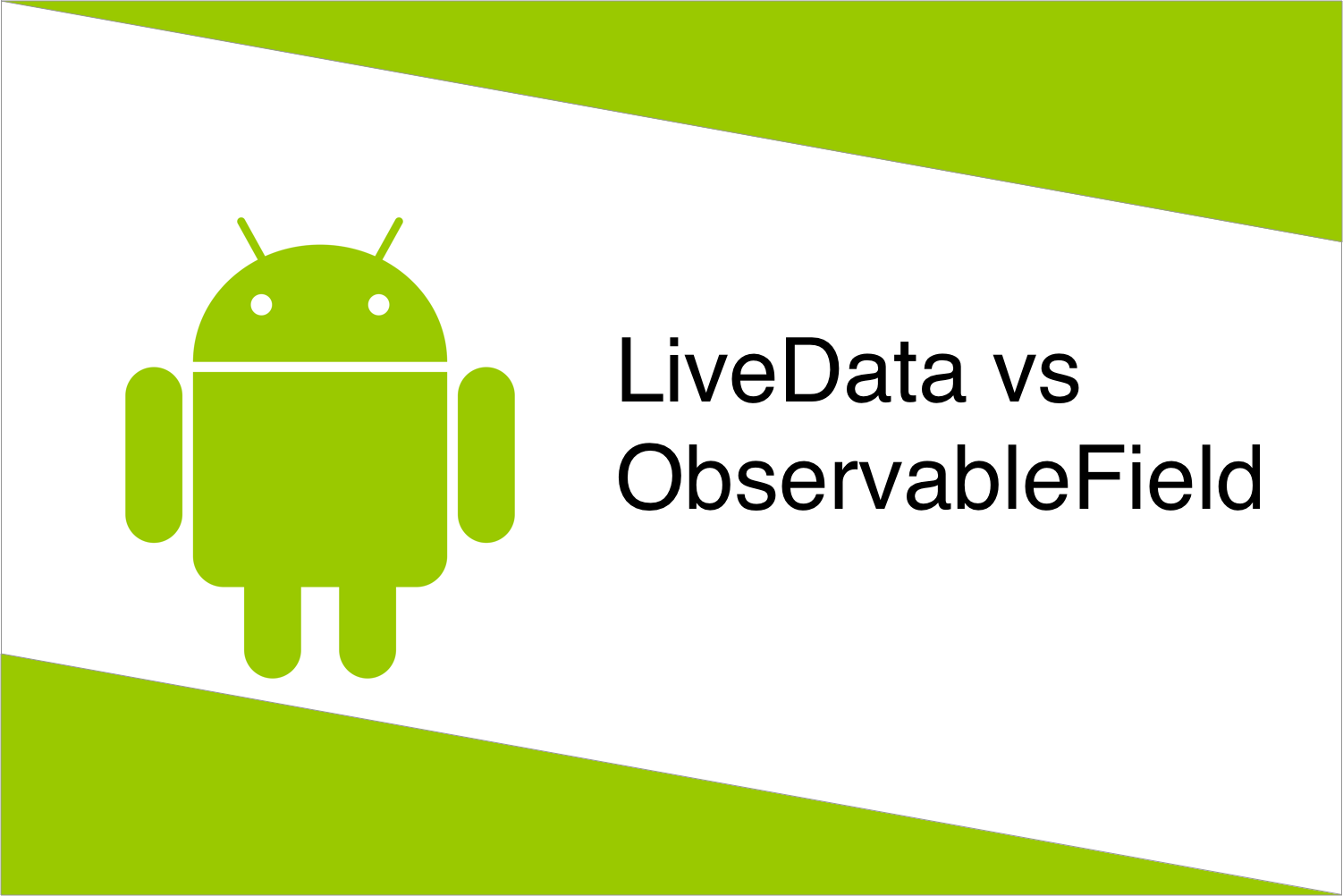
Ever received data from API and used the data to perform some action. We have done it using multiple ways, either just calling the API in the Fragment or Activity class and on its successful response we have just performed the action. Just wondering if we have to perform "n" number of API call, the View file (Activity/Fragment) will be loaded with lots of API calls
With the evolution of Android, there has a lot of patterns being followed like MVVM or MVP to remove the dependency(API calls) from View file.
In Google IO 2017, they launched the new form of Architecture on top of MVVM called Android Architecture Components . It contains a lot of amazing new features but one of them was LiveData.
LiveData
is an observable data holder class which just observes the changes in the View layer and updates the view.
But, before this there is a ObservableField on similar grounds like Livedata and it was also a type of Data holder which can be observed to the get the data.
Now, let's understand ObservableField and why LiveData was required even when ObservableField was present.
So , let's consider an example. We have an API Call from which we get some response and we have to show that reponse in our Activity.
public final ObservableField<String> userName = new ObservableField<>();
Now, let's add a callback to listen for changes to the Observable,
userNameField.addOnPropertyChangedCallback(userNameCallback);
Here, the nameFieldCallback looks like,
OnPropertyChangedCallback userNameCallback = new OnPropertyChangedCallback() {
@Override
public void onPropertyChanged(Observable observable, int i) {
//do the task here.
userName.set(/ **your data** /);
}
};
}
and to get the value from ObservableField, we use
userName.get()
So, to observe the name field we need to call the following function from our View file.
public String getName (){
return userName.get();
}
Now, let's discuss the LiveData
Now, LiveData is similar to ObservableField, a data holder. Let us use the above example to demonstrate LiveData.
We will declare the livedata using the following in ViewModel,
private MutableLiveData<String> name;
Now, to set the data in the LiveData, we use
name.setValue(/** your data **/)
and to return the liveData to get observed in View,
public LiveData<String> getName(){
return name;
}
Now, in the View(Activity/Fragment) file to observe it, we use,
mViewModel.getName().observe(this, name -> {
//do your taks
}
Here, we are observing the changes made to the LiveData.
But what exactly is the difference between ObservableField and LiveData?
- ObservableField <T> is not Lifecycle aware but LiveData is. That means LiveData will only update app component observers that are in an active lifecycle state and not which are inactive.
- We have to do manual handling of Lifecycle awareness in ObservableField
What are the benefits of Using LiveData?
- No memory Leaks : As the observers are bound to the lifecycle of the app, when not required the it is destroyed
- No Crashes due to Stopped Activity : As the Activity is not in use, so is the LiveData. So the data streaming stops which stops the crashes in the back stack.
- Configuration Changes : It handles the latest data when view is recreated due to screen rotation.
Happy Coding :)
Team MindOrks.