Understanding LiveData in Android
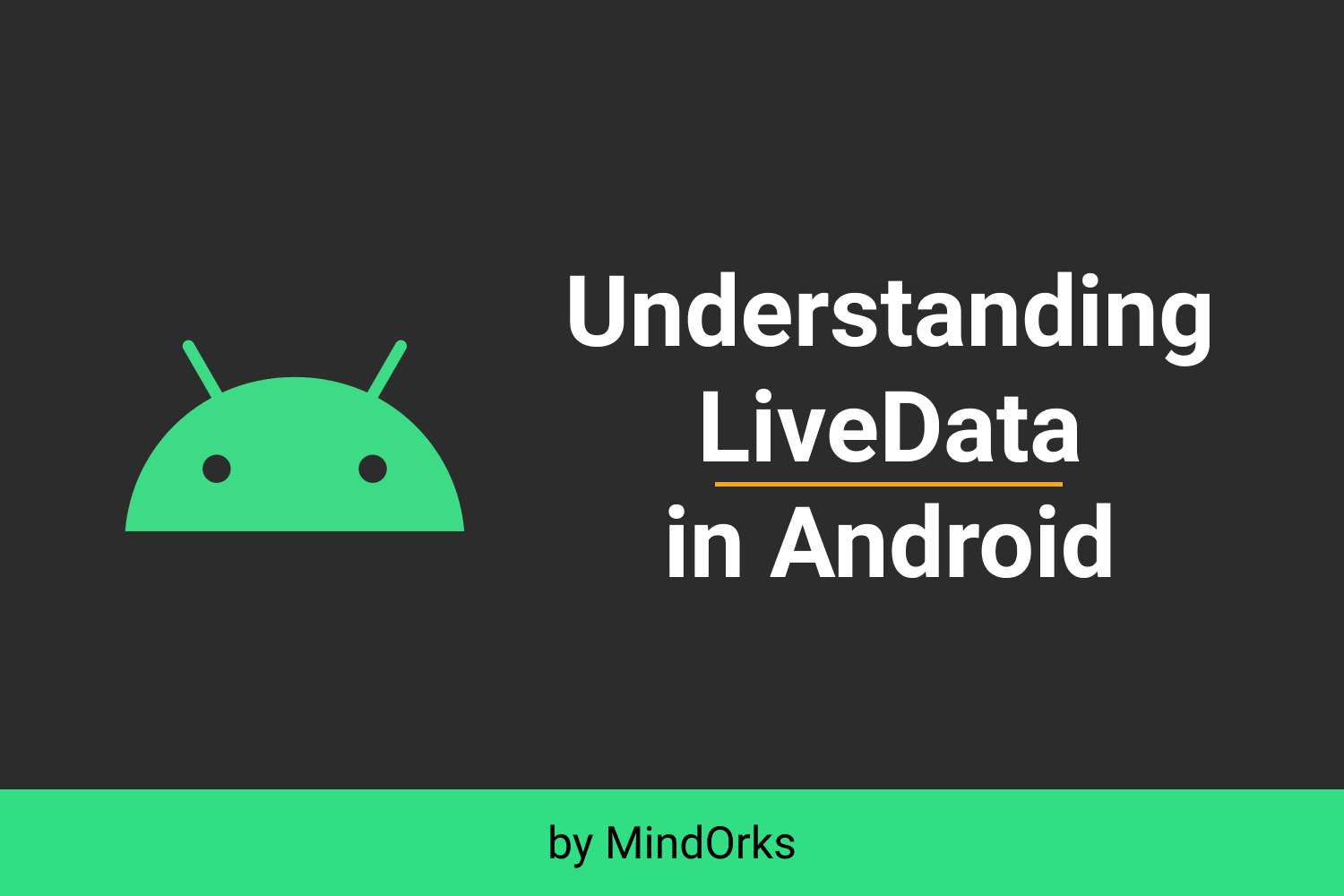
Updating the UI of the application due to change in some data is one of the most used tasks in Android. For example, whenever you like some post on Instagram, then the like count will be increased and also the color of the like button will be changed to red from white. So, here we are changing the UI based on some data and there are many other examples of these kinds of UI change due to some change in data in Android.
So, these changes in the views of your app can be carried out with the help of ViewModel and to make the working of a ViewModel easy, Google introduced the concept of LiveData . LiveData is a part of Android Jetpack and in this blog, we are going to understand the concept of LiveData in Android.
What is LiveData?
Let's first look at the definition mentioned in the official Android documentation:
LiveData is an observable data holder class. Unlike a regular observable, LiveData is lifecycle-aware, meaning it respects the lifecycle of other app components, such as activities, fragments, or services. This awareness ensures LiveData only updates app component observers that are in an active lifecycle state.
Let's have a simpler definition now.
In simple words, LiveData is basically a data holder and it is used to observe the changes of a particular view and then update the corresponding change. It is lifecycle-aware i.e. whenever a data is updated or changed then the updates will be sent to only those app components which are in active state. If the app component is not active and in the future, if it becomes active, then the updated data will be sent to that app component. So, you need not worry about the lifecycle of the app component while updating data.
For example, if you are not using LiveData and you are updating some TextView with some value, then on rotation of the screen, you need to again set the new value in the TextView because the Activity will be recreated on screen rotation. But if you are using LiveData, all you need to do is just update the value and that's it. No need to care about the lifecycle.
So, here are the things that will encourage you to use LiveData in your application:
- You can use LiveData with libraries like Room Persistent Library, Coroutines, etc.
- Once a data is modified/changed, the observers will be notified about the change
- But before notifying the observers, LiveData will check if the observer is live or not. If it is live, then the notification will be sent otherwise not. In this way, the crash due to stopped activities will be stopped.
- While using LiveData, you need not worry about unsubscribing any observers.
- If the inactive observer will be resumed in future, then the latest data will be sent to that observer.
- No need to worry about activity recreation due to screen rotation because only the updated data will be sent.
Four basic steps to work with LiveData
In order to use LiveData in your project, all you need to do is follow the below four steps:
- Firstly, for holding the data, you need to create a LiveData instance in your ViewModel.
-
After creating the instance of LiveData, you need to set the data in the LiveData by using methods like
setValue
andpostValue
. - After that, you need to return the LiveData so that it can be observed by some observer in the views like Activity or Fragments.
-
And finally, you need to observe the data in your views with the help of
observe
method. In the observer, you need to define all the changes in the UI that you want to perform on data change.
After following the above four steps, whenever there is a change in the data stored in LiveData then all the observers associated with the LiveData will be notified if they are live otherwise they will be notified when they come into the resume state.
So, let’s see how we can code for the above four steps:
Step 1: Creating an instance of LiveData
Here, we are going to use
MutableLiveData
that will be used for updating the
name
.
private MutableLiveData<String> name;
Step 2: Set the data in LiveData
Here, we need to change/update the data in LiveData. The MutableLiveData publicly exposes two methods i.e.
setValue
and
postValue
to set the data in LiveData.
So, here are some of the points that you must think before using
and
setValue
:
postValue
-
If you are working on the main thread, then both
setValue
postValue
-
If working in some background thread, then you can't use
setValue
postValue
postValue
[ Learn more about the difference between setValue() and postValue() from here]
Here is the code for updating LiveData using
setValue
and
postValue
:
viewModel.name.setValue(/** updated data **/);
or
viewModel.name.postValue(/** updated data **/);
Step 3: Returning LiveData
After setting the data, you need to return the LiveData so that it can be observed in some view.
public LiveData<String> getName(){
return name;
}
Step 4: Observe the data in some view
Finally, you need to observe the data in some view by using observers. One thing to be noted here is that only those observers will be notified that are active. When an observer comes from an inactive to an active state, then the updated data will be sent to it.
viewModel.getName().observe(this, name -> {
//do some UI updation or other tasks
}
This is how you can use LiveData by just following the above four steps.
Conclusion
So, in this blog, we learned about LiveData and how to use it. We saw that if there is a change in data then that data will be reflected to all the observers associated with it but it only notifies the changes to the observers that are live or in the active state and not to that observer that are in the inactive state.
Hope you liked this blog. To learn more about some of the cool topics of Android, you can visit our blogging website . Also, you can learn how to use LiveData in Room persistent library from here .
Keep Learning :)
Team MindOrks!