LiveData setValue vs postValue in Android
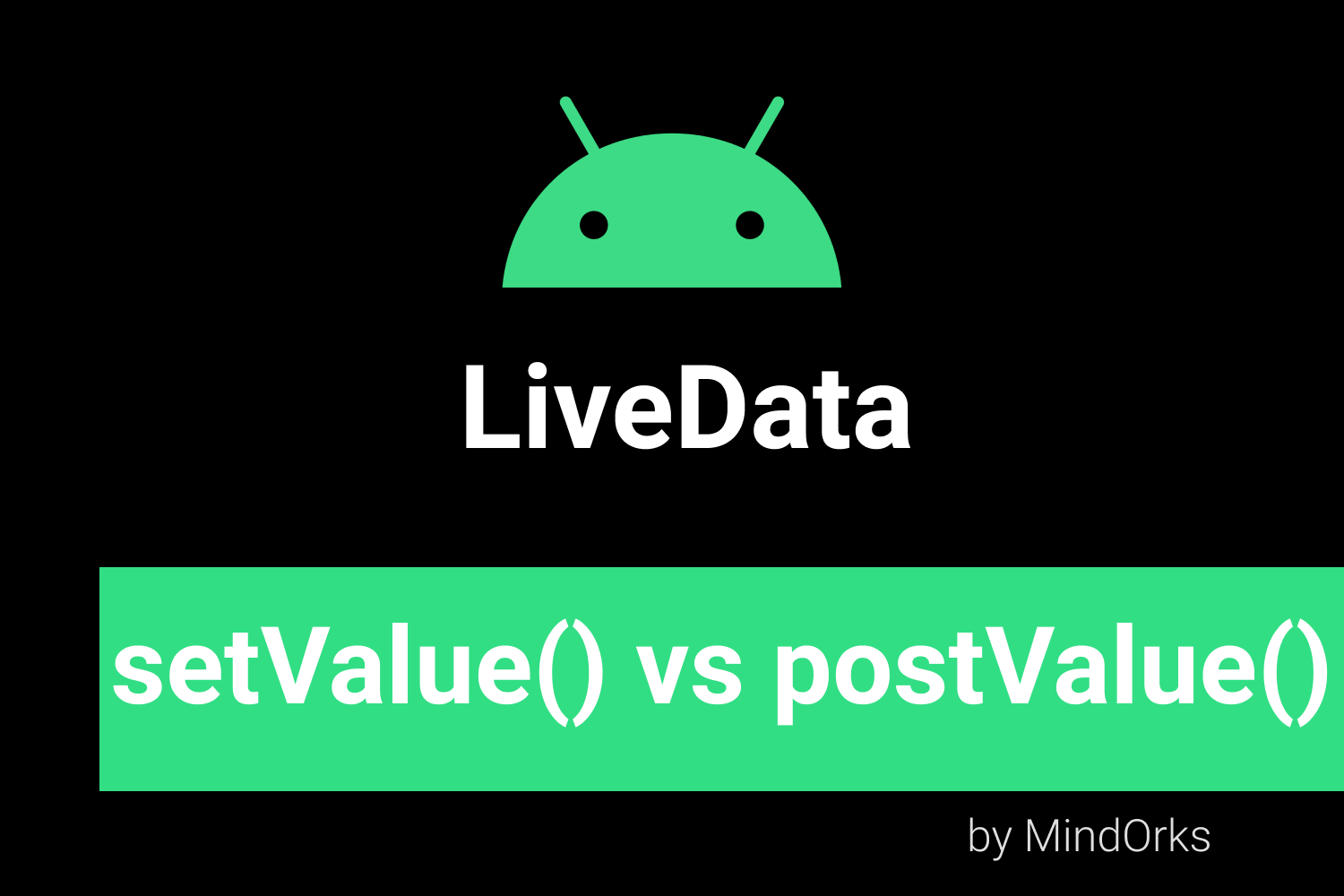
In Android, we deal with lots and lots of data. The data of one activity is being used in some other activity or fragment. For example, we can change the UI of an application when there is a change in some data. So, the basic idea is to have efficient communication between the data and the UI of the application and we all know that the best way to do this is by using LiveData which is a part of Android Jetpack.
The most commonly used methods of LiveData is
setvalue
and
postValue
. By name, these two functions are quite confusing i.e. it seems that both are used for the same purpose. But in reality, it is very different.
So, in this blog, we will start with a quick recap about
LiveData
and then we will learn the difference between
setValue
and
postValue
methods.
What is LiveData?
If we look at the definition of LiveData from the Android Developer Website, then it says that:
LiveData is an observable data holder class. Unlike a regular observable, LiveData is lifecycle-aware, meaning it respects the lifecycle of other app components, such as activities, fragments, or services.
In a simple way, LiveData is basically a data holder and it is used to observe the changes of a particular view and then update the corresponding change.
So, LiveData is used to make the task of implementing ViewModel easier. The best part about LiveData is that the data will not be updated when your View is in the background and when the view will come in the foreground, then it will get the updated data only.
Some of the advantages of LiveData includes:
- No memory leaks
- Views always get the up to date data
- No crash due to stopped activities
Learn more about LiveData from here.
Difference between setValue() and postValue()
So, if you are familiar with LiveData, then you must have encountered with these two methods of LiveData i.e.
setValue()
and
postValue()
. So, one question that might have come in your mind is why is there a need for two methods
setValue
and
postValue
for the same functionality? Let’s find out by taking an example.
Suppose you want to update some value using the LiveData. So, you will be having two options i.e. either you can update the data on the Main thread or you can update the data using the background thread. So, the use-case of
setValue
and
postValue
depends on these two situations only.
While using the Main thread to change the data, you should use the
setValue
method of the MutableLiveData class and while using the background thread to change the LiveData, you should use the
postValue
method of the MutableLiveData class.
So, the duty of the
postValue
method is to post or add a task to the main thread of the application whenever there is a change in the value. And the value will be updated whenever the main thread will be executed. So, basically, it is requesting the main thread to set the new updated value and then notify the observers.
While the
setValue
method is used to set the changed value from the main thread and if there are some live observers to it, then the updated value will also be sent to those observers as well.
This
setValue
method must be called from the main thread.
So, here are some of the points that you must think before using
setValue
and
postValue
:
-
If you are working on the main thread, then both
setValue
andpostValue
will work in the same manner i.e. they will update the value and notify the observers. -
If working in some background thread, then you can't use
setValue
. You have to usepostValue
here with some observer. But the interesting thing aboutpostValue
is that the value will be change immediately but the notification that is to be sent to observers will be scheduled to execute on the main thread via the event loop with the handler.
Let’s take an example,
// setValue
liveData.setValue("someNewData")
liveData.setValue("againNewData")
// postValue
liveData.postValue("someNewData")
liveData.postValue("againNewData")
In the above example, the
setValue
is called from the main thread and the
postValue
is called from some background thread.
Since the
setValue
is called twice, so the value will be updated twice and the observers will receive the notification regarding the updated data twice.
But for
postValue
, the value will be updated twice and
the number of times the observers will receive the notification depends on the execution of the main thread.
For example, if the
postValue
is called 4 times before the execution of the main thread, then the observer will receive the notification only once and that too with the latest updated data because the notification to be sent is scheduled to be executed on the main thread. So, if you are calling the
postValue
method a number of times before the execution of the main thread, then the value that is passed lastly i.e. the latest value will be dispatched to the main thread and rest of the values will be discarded.
Another thing to care about
postValue
is that, if the field on which the
postValue
is called is not having any observers and after that, you call the
getValue
, then you will not receive the value that you set in the
postValue
because you don't have an observer here.
Conclusion
In this blog, we learned how to use LiveData to implement the concept of ViewModel in our project. LiveData is used to notify the user if there is a change in the value of objects of LiveData. For this purpose, we use two methods i.e.
setValue
and
postValue
. We also learned about these two methods. So, I hope you enjoyed this blog. For more information about ViewModels, you can read our blog on
ViewModel
.
Keep Learning :)
Team MindOrks!