In-App Review in Android
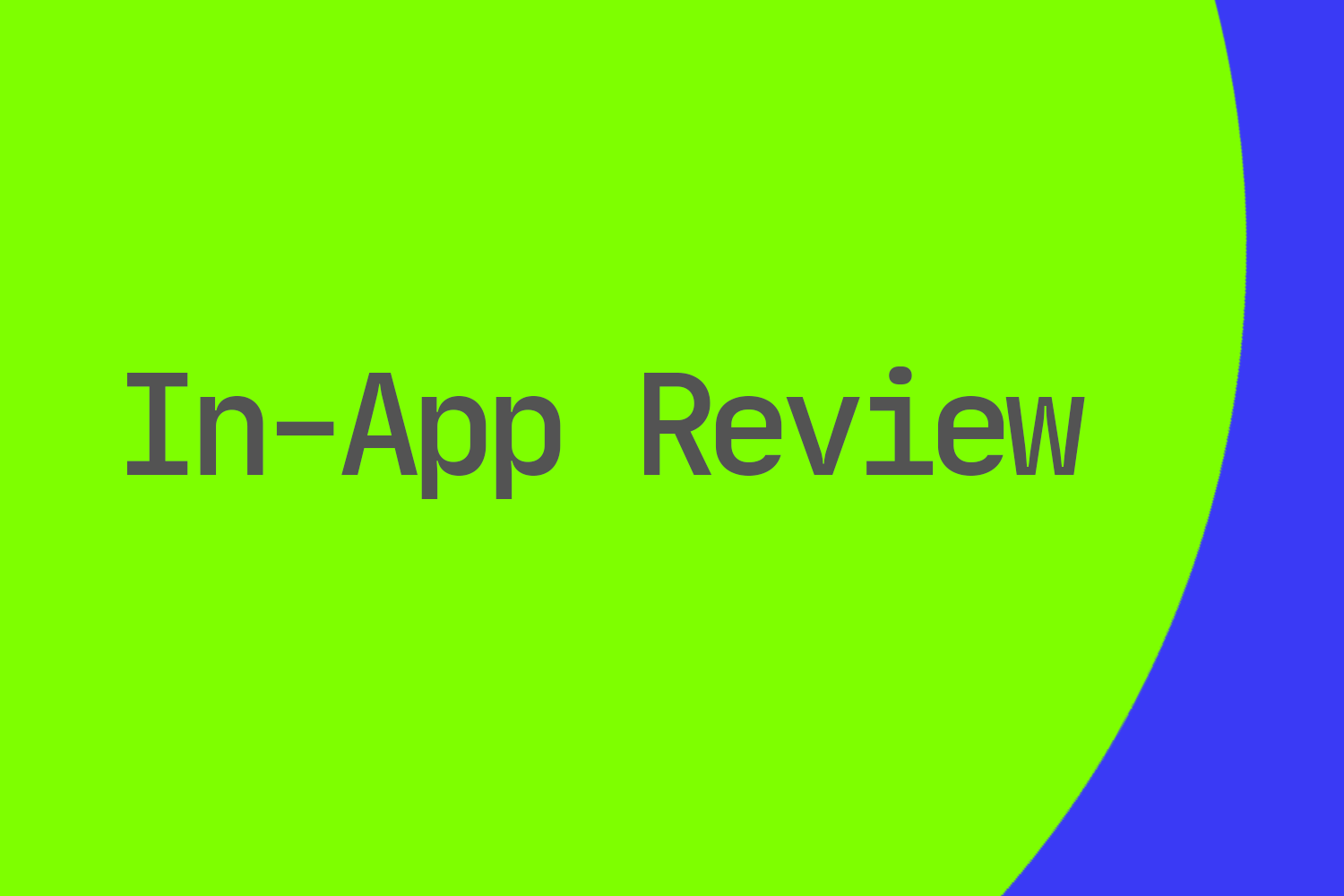
So, you released an app in the play store and users are liking it. But now we want the user's liking to be shown in the play store via reviews.
But the first question is why we need the reviews?
Having good reviews in your application makes a lot of impact on your product like good ranking in the play store and it also helps a new user to trust your app more.
But to date what we use to do is, we use to show some custom to take them to play store and expect users would give the review there.
But it has few issues like,
- The user might choose not to add any review and just leave.
- The user might drop from the app and that's a bad point as the user is leaving the app. We don't want our users to leave the application.
To solve these problems, Google launched an In-App Review API, as a part of the play core library which we can use to add reviews from our application itself without leaving the application and that provides a very smooth user experience as well to the users.
In this blog, we are going to learn,
- When to ask the user to add their review for the app.
- How to integrate the new In-App review API?
- How to test the InApp Review?
When to ask the user to add review the app.
Considering that the user has liked a feature in your application or has completed some level in your game, that might be a place to show the Review UI to the user.
Google has also provided some guidelines for where not to ask for the review like,
- We should not add custom UI alongside the Review UI flow.
- We should not prompt the user frequently for a review, it has quotas limit associated with it. Also, we should not have any call to action like a button to trigger the In-App Review API, the users might hit their quota by triggering again and again.
How to integrate the new In-App review API?
Let's start the integration of the API in our application.
Step 01:
As the In-App Review is a part of the Play core library, we need to add the required dependencies in our app's build.gradle file like,
implementation 'com.google.android.play:core:1.8.2'
implementation 'com.google.android.play:core-ktx:1.8.1'
Step 02:
Since the integration of dependencies is done, we need to figure out the correct place where we need to ask the user for a review. Make sure that you ask at a happy place where the user has achieved something like, For example,
- If you are an app who is in the rental space business and help people get roommates for their vacant space then the best place to ask review might be when you connect the user with a potential roommate.
- If you are a gaming app, then maybe show them after completing a level or something similar to it.
They would happily rate your application and leave a good review as they already had a good experience for what they were looking for.
Step 03:
Now, since we have figured out the best trigger point to ask for a review, let us start integrating the Review API itself.
First, we need to create the instance of
ReviewManager
which would help us to start the API. We create the instance using,
val manager = ReviewManagerFactory.create(context)
Now, using this manager object we have to request the flow to launch the In-App review flow. We do it like,
val request = manager.requestReviewFlow()
Once this is done, we can check if the request was successful in its onCompleteListener then let's start the in-app flow like,
request.addOnCompleteListener { request ->
if (request.isSuccessful) {
val reviewInfo = request.result
} else {
//Handle the error here
}
}
And when we get the reviewInfo object, we just start the review process like,
request.addOnCompleteListener { request ->
if (request.isSuccessful) {
val reviewInfo = request.result
val flow = manager.launchReviewFlow(this, reviewInfo)
flow.addOnCompleteListener { _ ->
//Continue your application process
}
} else {
//Handle the error here
}
}
Inside the addOnCompleteListener, we just keep the application's flow running irrespective of what the results are.
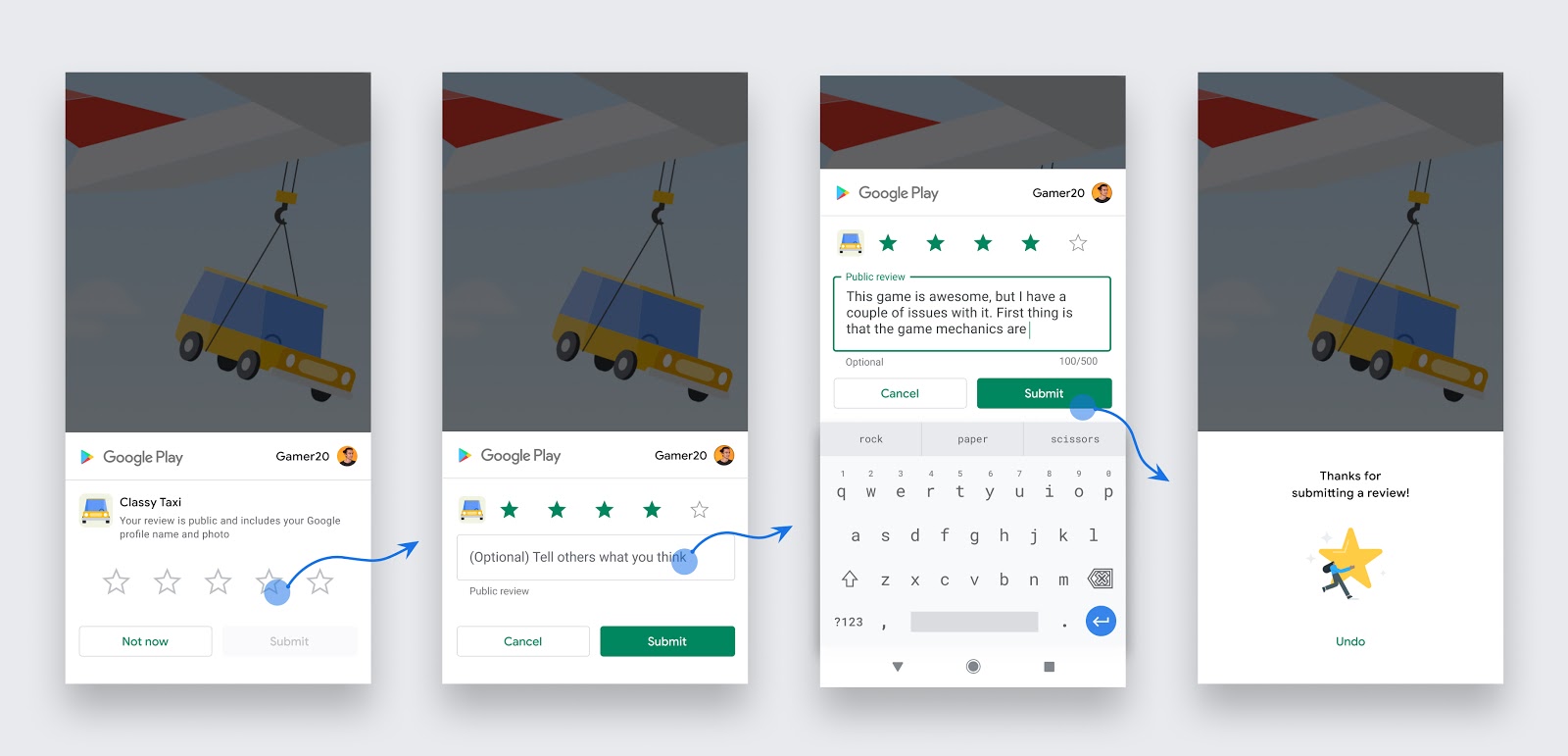
Image Source: developer.android.com
Now, we are done setting up the In-App Review in our application.
How to test the In-App Review?
Now, since we have integrated the flow, we need to test it out and make sure it works fine for us. To do it, we have a couple of ways to test it out like,
- Upload your application in the internal test track and test it out.
- Upload your application in internal app sharing and test the review flow.
Now, while testing it in test cases to check if the review flow is completed and the app's flow is executing in the expected flow then we used FakeReviewManager in place of ReviewManagerFactory like,
val manager = FakeReviewManager(this)
This would not trigger the UI but only take care of faking the result by giving us the fake ReviewInfo object.
Point to take care of while using In-App Review API
- You will see the Review UI only if your primary account has not already reviewed the app.
Happy Learning :)
Show your love by sharing this blog with your fellow developers.