How to open a PDF file in Android programmatically?
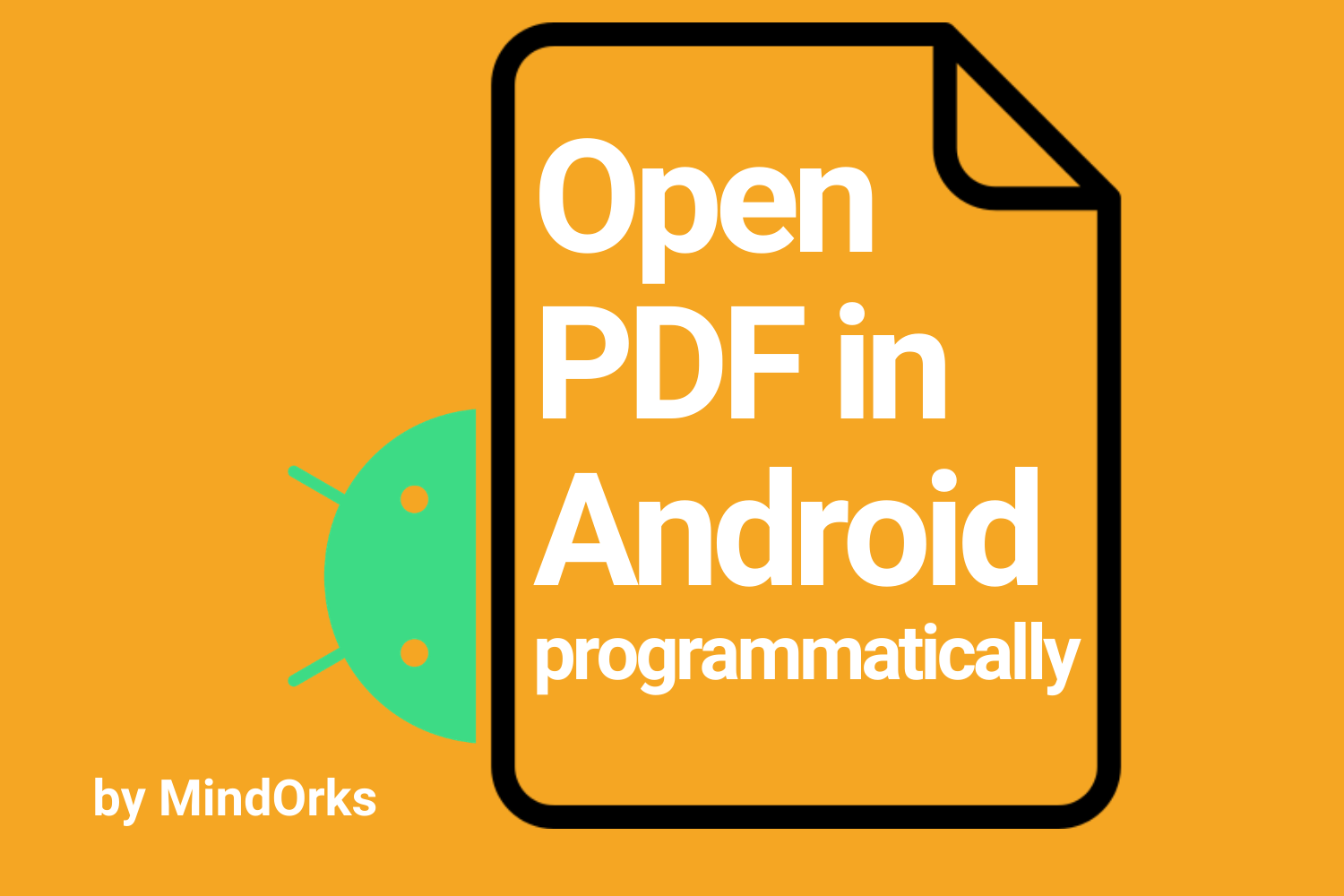
Opening and viewing documents in Android applications are very interesting and a must to have in every application. You can open any application present on your mobile and you will find that every application contains documents in some or the other way. And among these documents, the most popular and widely used document format is the PDF format.
PDF or Portable Document Format is a file format that has captured all the elements of a printed document. This is the most used document format. For example, in the Paytm application, you get your monthly expenses in the form of PDF document. So, if you also want to display some kind of document in your application, then you can open this PDF format document.
So, welcome to MindOrks and in this tutorial, we will learn how to open a PDF file in Android programmatically. We will cover the below topics in this tutorial:
- Project setup
- Ways of opening PDF in Android
- Making UI and adding Activities for the project
- Opening a PDF file using WebView
- Opening a PDF file using AndoirdPdfviewer library
- Project source code and What next?
Project setup
In this tutorial, we will make a project and try various ways of opening PDF file, Here we are going to set up our project:
- Start a new Android Studio Project
- Select Empty Activity and Next
- Name: Open-PDF-File-Android-Example
- Package name: com.mindorks.example.openpdffile
- Language: Kotlin
- Finish
- Your starting project is ready now
-
Under your root directory, create a package named
utils
.(right-click on root directory > new > package) -
In the utils package, create one object classes:
FileUtils
.(right-click on utils > new > Kotlin file/class > Object class)
Ways of opening PDF in Android
If you want to display PDF in your Android application, there are various ways of doing it. Some of the ways of opening the PDF can be:
- From Assets: Let’s take an example, if you want to display some icons in your application then you will put all your icons in the drawable folder and then you will use those icons in your application. Same is with the case of PDF files also. If you have some PDF file that is constant and you want to display it in your application then you can put that PDF file in the assets folder and use that PDF in your app. One example can be the Terms and Conditions file. The terms and conditions files are rarely changed. So, you can put that document in the assets folder and use it.
- From Device: The other way of opening a PDF is to open it from the device itself. Here, you can open the PDF files present in your mobile device. This is the most used approach for opening the PDF in an Android device.
- From the Internet: Here, you can open PDF files from the internet. All you need to do is just use the URL of the PDF file and after downloading the PDF file, you can open the PDF file in your mobile application.
So, we will look upon all these ways of viewing the PDF in your Android Application. Let’s make the UI of the project.
Making UI for the project
In our example, we are going to cover four different cases:
- Opening a PDF file using WebView
- Opening a PDF file from assets using AndroidPdfViewer library
- Opening a PDF file form storage using AndroidPdfViewer library
- Opening a PDF file from the internet using AndroidPdfViewer library
So, for the first point, we will use
WebViewActivity
and for 2nd, 3rd, and 4th point, we will be using
PdfViewActivtiy
.
Create two activities named
WebViewActivtiy
and
PdfViewActivity
.(right-click on root directory > new > Activity> Empty Activity)
Now, for the above four actions, create four buttons and assign the task to open activity by those four buttons.
The code for the
activity_main.xml
file is:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/secondary_color"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="56dp"
android:layout_margin="24dp"
android:gravity="center"
android:text="@string/opening_pdf"
android:textColor="@color/color_black"
android:textSize="32sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/buttonWebView"
android:layout_width="match_parent"
android:layout_height="56dp"
android:layout_margin="24dp"
android:background="@color/primary_color"
android:text="@string/open_in_webview"
android:textAllCaps="false"
android:textColor="@color/color_white"
android:textSize="20sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<Button
android:id="@+id/buttonAssets"
android:layout_width="match_parent"
android:layout_height="56dp"
android:layout_margin="24dp"
android:background="@color/primary_color"
android:text="@string/open_from_assets"
android:textAllCaps="false"
android:textColor="@color/color_white"
android:textSize="20sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonWebView" />
<Button
android:id="@+id/buttonStorage"
android:layout_width="match_parent"
android:layout_height="56dp"
android:layout_margin="24dp"
android:background="@color/primary_color"
android:text="@string/open_from_storage"
android:textAllCaps="false"
android:textColor="@color/color_white"
android:textSize="20sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonAssets" />
<Button
android:id="@+id/buttonInternet"
android:layout_width="match_parent"
android:layout_height="56dp"
android:layout_margin="24dp"
android:background="@color/primary_color"
android:text="@string/open_from_internet"
android:textAllCaps="false"
android:textColor="@color/color_white"
android:textSize="20sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/buttonStorage" />
</androidx.constraintlayout.widget.ConstraintLayout>
In the
MainActivity.kt
file, we call the desired activity with the corresponding buttons:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
setUpOnClickListener()
}
private fun setUpOnClickListener() {
buttonWebView.setOnClickListener {
val intent = Intent(this, WebViewActivity::class.java)
startActivity(intent)
}
buttonAssets.setOnClickListener {
val intent = Intent(this, PdfViewActivity::class.java)
intent.putExtra("ViewType", "assets")
startActivity(intent)
}
buttonStorage.setOnClickListener {
val intent = Intent(this, PdfViewActivity::class.java)
intent.putExtra("ViewType", "storage")
startActivity(intent)
}
buttonInternet.setOnClickListener {
val intent = Intent(this, PdfViewActivity::class.java)
intent.putExtra("ViewType", "internet")
startActivity(intent)
}
}
}
We are done with the UI part. Let's learn how to view PDF from WebView.
Opening a PDF file in Android using WebView
The very first and the easiest way of displaying the PDF file is to display it in the
WebView
. All you need to do is just put WebView in your layout and load the desired URL by using the
webView.loadUrl()
function.
So, add a WebView in the
activity_web_view.xml
file:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WebViewActivity">
<WebView
android:id="@+id/webView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Now, open the
FileUtils
class of the utils package that we created at the starting of this blog and add a function named
getPdfUrl
that will return the URL of the PDF which we are going to view in the WebView. Here, I am using the syllabus of
MindOrks Professional Course
. Add below method in
FileUtils
class:
fun getPdfUrl(): String {
return "https://mindorks.s3.ap-south-1.amazonaws.com/courses/MindOrks_Android_Online_Professional_Course-Syllabus.pdf"
}
Now, all we need to do is open the above URL in the WebView by calling the
webView.loadUrl
method. Following is the code of
WebViewActivity.kt
file:
class WebViewActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
webView.webViewClient = WebViewClient()
webView.settings.setSupportZoom(true)
webView.settings.javaScriptEnabled = true
val url = FileUtils.getPdfUrl()
webView.loadUrl("https://docs.google.com/gview?embedded=true&url=$url")
}
}
The last thing that you need to do is adding
INTERNET
permission to your application. So, add the below line in your
AndroidManifest.xml
file:
<uses-permission android:name="android.permission.INTERNET" />
Now, run the application on your mobile phone and the PDF will be displayed on the screen.
Note: The opening of PDF in WebView depends on your internet speed, so wait for sometimes if your internet is slow.
Opening a PDF file in Android using AndroidPdfViewer library
There are various libraries that can be used to display PDF files in our application. In our tutorial, we will learn how to open a PDF file from Assets, Phone Storage, and from the Internet by using the
AndroidPdfViewer
library.
Also, we will be using
PRDownloader
library by MindOrks to download files from the Internet and open it by
AndoridPdfViewer
.
Adding dependencies and permissions
Open the app level
build.gradle
and add the below dependencies of AndroidPdfViewer and PRDownloader:
implementation 'com.github.barteksc:android-pdf-viewer:2.8.2'
implementation 'com.mindorks.android:prdownloader:0.6.0'
Since we will be reading pdf from INTERNET. Open the AndoidManifest.xml file and add the below:
<uses-permission android:name="android.permission.INTERNET" />
Adding PDFView
The AndroiPdfViewer provides a PDFView to display PDF files in it. So, write the below code in
actvity_pdf_view.xml
:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".PdfViewActivity">
<com.github.barteksc.pdfviewer.PDFView
android:id="@+id/pdfView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<ProgressBar
android:id="@+id/progressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/pdfView" />
</androidx.constraintlayout.widget.ConstraintLayout>
We can use the AndroidPdfViewer to open the PDF from:
- Assets folder
- Phone storage
- Internet
So, we need to write the code to connect the button click of
MainActivity
with the above events. Create a function named
checkPdfAction()
and write the below code:
private fun checkPdfAction(intent: Intent) {
when (intent.getStringExtra("ViewType")) {
"assets" -> {
// perform action to show pdf from assets
}
"storage" -> {
// perform action to show pdf from storage
}
"internet" -> {
// perform action to show pdf from the internet
}
}
}
Call the above method from the
onCreate()
:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_pdf_view)
checkPdfAction(intent)
}
Let's learn how to display PDF from assets, storage, and internet.
Assets Folder
Firstly, we will look upon how to view PDF, stored in the Assets Folder.
Creating an assets folder
Create an assets folder by right-clicking on main > New Folder > Assets Folder and paste the PDF document into it.
PDF file name: MindOrks_Android_Online_Professional_Course-Syllabus.pdf
Create
getPdfNameFromAssets()
method
Create a method named
getPdfNameFromAssets
in the FileUtils class. This method will return the name of the PDF file present in the assets folder:
fun getPdfNameFromAssets(): String {
return "MindOrks_Android_Online_Professional_Course-Syllabus.pdf"
}
Now, in the
PdfViewActivity.kt
file, create a method
showPdfFromAssets
which will take the file name in string format and will use the
fromAssets()
method of AndroidPdfViewer library to display PDF:
private fun showPdfFromAssets(pdfName: String) {
pdfView.fromAsset(pdfName)
.password(null) // if password protected, then write password
.defaultPage(0) // set the default page to open
.onPageError { page, _ ->
Toast.makeText(
this@PdfViewActivity,
"Error at page: $page", Toast.LENGTH_LONG
).show()
}
.load()
}
Call the above method from the
checkPdfAction
and pass the file name by calling the
getPdfnameFromAssets
method of
FileUtils
class:
private fun checkPdfAction(intent: Intent) {
when (intent.getStringExtra("ViewType")) {
"assets" -> {
showPdfFromAssets(FileUtils.getPdfNameFromAssets())
}
"storage" -> {
// open pdf from storage
}
"internet" -> {
// open pdf from internet
}
}
}
Finally, run the application on your mobile device and see the output.
From Phone Storage
Now, we will look upon how to open PDF files from the Phone’s storage. So, we have to launch an intent to find the file having PDF format and the selected file will be displayed in the
PDFView
by calling the
fromUri
method.
Create a function
selectPdfFromStorage()
in the
PdfViewActivity.kt
file and add the below code:
companion object {
private const val PDF_SELECTION_CODE = 99
}
private fun selectPdfFromStorage() {
Toast.makeText(this, "selectPDF", Toast.LENGTH_LONG).show()
val browseStorage = Intent(Intent.ACTION_GET_CONTENT)
browseStorage.type = "application/pdf"
browseStorage.addCategory(Intent.CATEGORY_OPENABLE)
startActivityForResult(
Intent.createChooser(browseStorage, "Select PDF"), PDF_SELECTION_CODE
)
}
Once, the user selects a PDF, the
onActivityResult
will be called:
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == PDF_SELECTION_CODE && resultCode == Activity.RESULT_OK && data != null) {
val selectedPdfFromStorage = data.data
showPdfFromUri(selectedPdfFromStorage)
}
}
Now, create a method named
showPdfFromUri
that will take a Uri and display the PDF:
private fun showPdfFromUri(uri: Uri?) {
pdfView.fromUri(uri)
.defaultPage(0)
.spacing(10)
.load()
}
Now, you can check the output by running your application on your mobile device and select the desired PDF.
PDF from Internet
Lastly, our aim is to view the PDF files from the Internet. We will first download the PDF by using the
PRDownloader
and then use this file to display the PDF on your
PdfViewActiviy
by using the same process as used for Assets and Storage but here you have to use
fromFile()
to add display the PDF.
So, we need to download the file first by using the PRDownloader library. Initialise it in the
onCreate()
method of
PdfViewActivity
:
PRDownloader.initialize(applicationContext)
Now, you need to download the file from the INTERNET by using the
download()
method of PRDownloader. So, create a function named
downloadPdfFromInternet()
in the PdfViewActivity. This function will take the URL, directory path, and file name of the file to be downloaded.
private fun downloadPdfFromInternet(url: String, dirPath: String, fileName: String) {
PRDownloader.download(
url,
dirPath,
fileName
).build()
.start(object : OnDownloadListener {
override fun onDownloadComplete() {
Toast.makeText(this@PdfViewActivity, "downloadComplete", Toast.LENGTH_LONG)
.show()
val downloadedFile = File(dirPath, fileName)
progressBar.visibility = View.GONE
showPdfFromFile(downloadedFile)
}
override fun onError(error: Error?) {
Toast.makeText(
this@PdfViewActivity,
"Error in downloading file : $error",
Toast.LENGTH_LONG
)
.show()
}
})
}
The
onDownloadComplete
method will be called when the file is downloaded. So, call the
showPdfFromFile
method and pass the downloaded file to the method:
private fun showPdfFromFile(file: File) {
pdfView.fromFile(file)
.password(null)
.defaultPage(0)
.enableSwipe(true)
.swipeHorizontal(false)
.enableDoubletap(true)
.onPageError { page, _ ->
Toast.makeText(
this@PdfViewActivity,
"Error at page: $page", Toast.LENGTH_LONG
).show()
}
.load()
}
Finally, call the
downloadPdfFromInternet
method from the
checkPdfAction
method but we need the URL, directory name and file name of the file to be downloaded. We can get the URL by calling the
getPdfUrl
method of
FileUtils
class. Now, make a function
getRootDirPath
method in the
FileUtils
class that will return the root directory:
fun getRootDirPath(context: Context): String {
return if (Environment.MEDIA_MOUNTED == Environment.getExternalStorageState()) {
val file: File = ContextCompat.getExternalFilesDirs(
context.applicationContext,
null
)[0]
file.absolutePath
} else {
context.applicationContext.filesDir.absolutePath
}
}
Now, call the
downloadPdfFromInternet
method from
checkPdfAction
method of
PdfViewActivity
:
private fun checkPdfAction(intent: Intent) {
when (intent.getStringExtra("ViewType")) {
"assets" -> {
showPdfFromAssets(FileUtils.getPdfNameFromAssets())
}
"storage" -> {
selectPdfFromStorage()
}
"internet" -> {
progressBar.visibility = View.VISIBLE
val fileName = "myFile.pdf"
downloadPdfFromInternet(
FileUtils.getPdfUrl(),
FileUtils.getRootDirPath(this),
fileName
)
}
}
}
Finally, run the application and try to verify all the three options i.e. assets, storage and internet options to view PDF in Android Application. Try to replace the PDF link used in the above example with your own PDF URL.
There are many other methods present in the AndroidPdfViewer library. You can explore all the methods from here .
Project source code and What next?
You can get the source code of the whole project from here .
You can find more open-source project by MindOrks from here .
Have a look at our Interview Kit for company preparation.
Do share this tutorial with your fellow developers to spread the knowledge. You can read more blogs on Android on our blogging website .
Apply Now: MindOrks Android Online Course and Learn Advanced Android
Happy Learning :)
Team MindOrks!