Understanding open Keyword in Kotlin
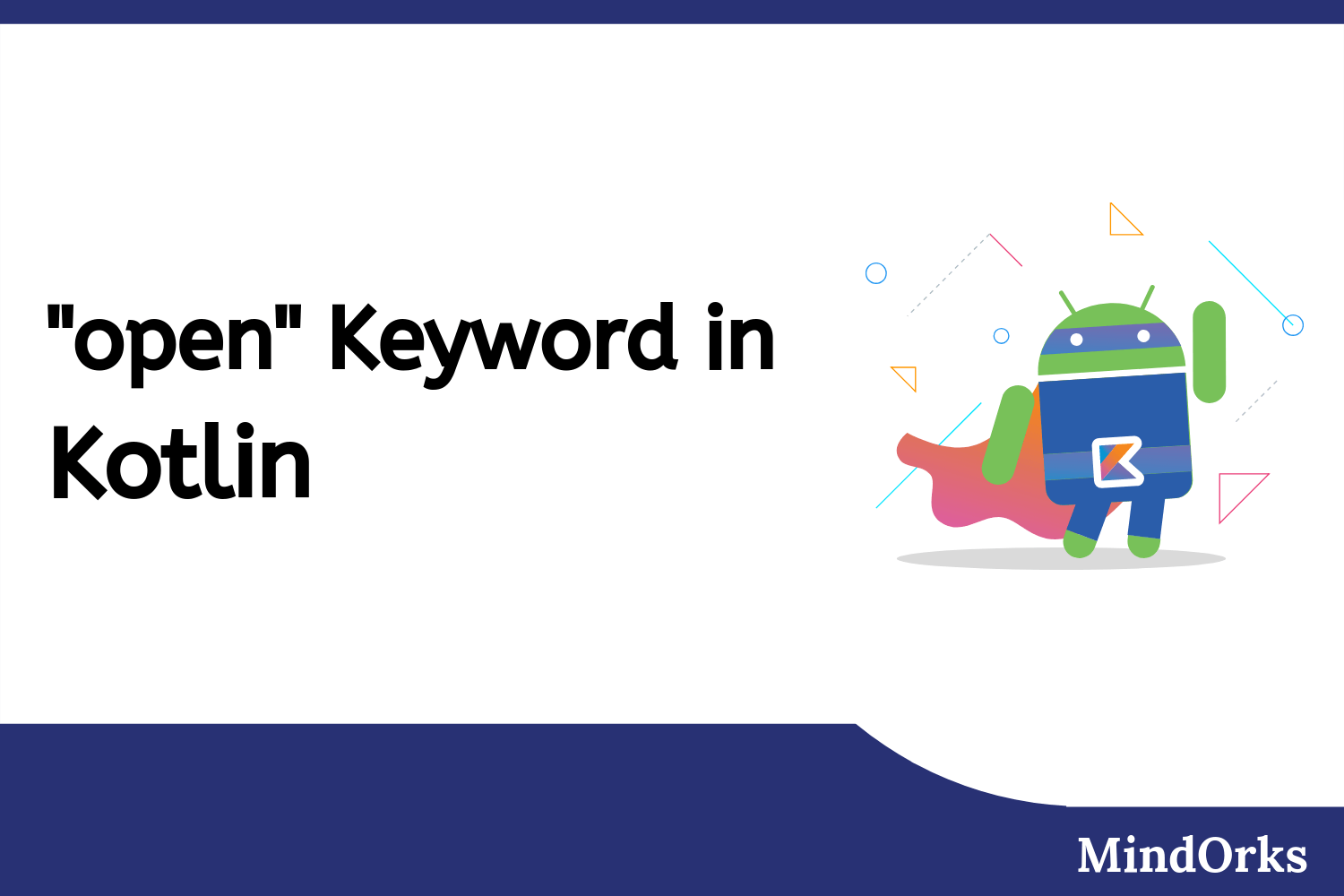
In this blog, you will learn about the “ open ” keyword in Kotlin. You will learn how and why to use open keyword with the class name, function name, and variable name.
We all know that Inheritance is the backbone of every Object-Oriented Programming language. It is a process of deriving or using the properties and characteristics of one class by the other. In simple words, if a class named ClassA is having some variables and functions and another class named ClassB is inheriting the ClassA , then, ClassB will use the variables and methods of ClassA . Here, ClassA is called the Parent Class and ClassB is called the Child Class . Inheritance enables re-usability. Following is an example of Inheritance:
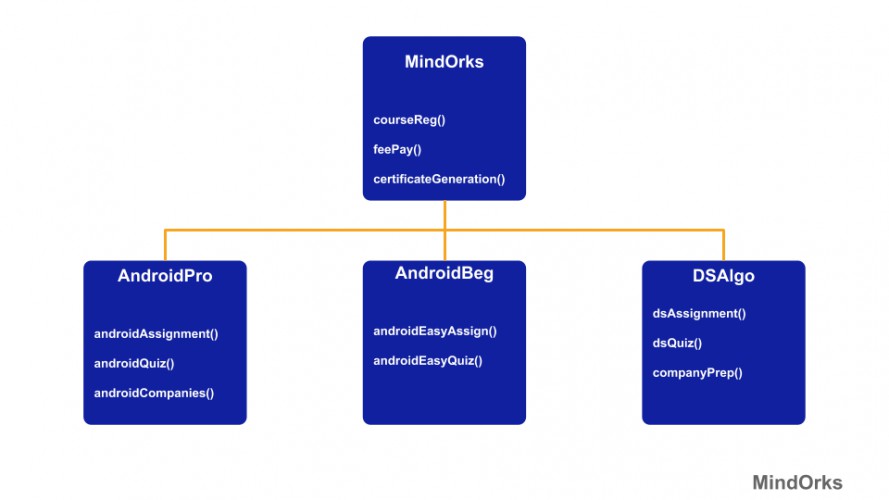
As you know that MindOrks has various online courses like Android for Professionals , Android for Beginners , and Data Structures & Algorithms . So, these are different courses but they share something. For enrolling in any of the three courses, you have to first register yourself, then you have to pay for the course and after completion of the course, your certificate will be generated. Now, think of the same situation in the Object-Oriented manner. Think of MindOrks as one class, AndroidPro (for the course named as “ Android for Professionals ”) as one class, AndroidBeg (for the course named as “ Android for Beginners ”) as one class, and DSAlgo (for the course named as “ Data Structures & Algorithm ”) as one class. Now, AndroidPro, AndroidBeg, and DSAlgo has three steps in common i.e. courseReg(), feePay(), and certificateGeneration(). So, instead of implementing these three methods separately in all the three classes, we can implement these methods in the MindOrks class and then use the methods by inheriting the MindOrks class. Here, MindOrks is the Parent class and AndroidPro, AndroidBeg, and DSAlgo are the Child class.
Inheritance in Kotlin
In Kotlin, to inherit one class from the other, you can use the below syntax:
//Base Class
open class MindOrks {
}
//Derived class
class AndroidPro : MindOrks(){
}
Here, in the above example, we are inheriting the class MindOrks from the class AndroidPro . So, MindOrks is the Parent class and AndroidPro is the Child class.
The “open” Keyword
In Kotlin, all the classes are final by default i.e. they can’t be inherited by default. This is opposite to what we learned in Java. In Java, you have to make your class final explicitly.
So, to make a class inheritable to the other classes, you must mark it with the open keyword otherwise you will get an error saying “ type is final so can’t be inherited ”.
“open” Keyword with Functions
Just like classes, all the functions in Kotlin are by default final in nature i.e. you can’t override a function when the function is final in nature.
Function Overriding is a process of redefining the functions of a Base class in a Child class. Also, in the child class, you have to use the override modifier.
Following is an example of a function overriding in Kotlin:
open class MindOrks {
//use open keyword to allow child class to override it
open fun courseName(){
println("Course Name")
}
}
class AndroidPro : MindOrks(){
//use the override keyword to override the function
override fun courseName() {
println("Android for Professionals")
}
}
Here, in the above example, the Parent class MindOrks is having a function named courseName() and this MindOrks class is inherited by the AndroidPro class. In the AndroidPro class we are overriding the method courseName() and we are redefining the body of the function.
“open” Keyword with Variables
Just like classes and functions, the variables in Kotlin are by default final in nature. So, to override it in the Child class, you need to set the variables as open in the Base class. Following is an example of the same:
open class MindOrks {
//use open keyword to allow child class to override it
open val courseId: Int = 0
//use open keyword to allow child class to override it
open fun courseName(){
println("Course Name")
}
}
class AndroidPro : MindOrks(){
//use the override keyword to override the variable
override val courseId: Int = 1
//use the override keyword to override the function
override fun courseName() {
println("Android for Professionals")
}
}
In the above example, the courseId in the MindOrks class is set to 0 but in the Child class i.e. in AndroidPro class, the value is changed to 1.
Summary
In Kotlin, the classes, the functions, and the variables are final in nature by default i.e. they can’t be inherited from any other class. So, to make it inheritable from other classes, we use the open keyword with the class, function, and variable name.
Hope you learned something new today.
Do share this blog with your fellow developers to spread the knowledge. You can read more blogs on Android on our blogging website .
Apply Now: MindOrks Android Online Course and Learn Advanced Android
Happy Learning :)
Team MindOrks!
Also, Let’s connect on Twitter , Linkedin , Github , and Facebook