How to implement Splash Screen in Android?
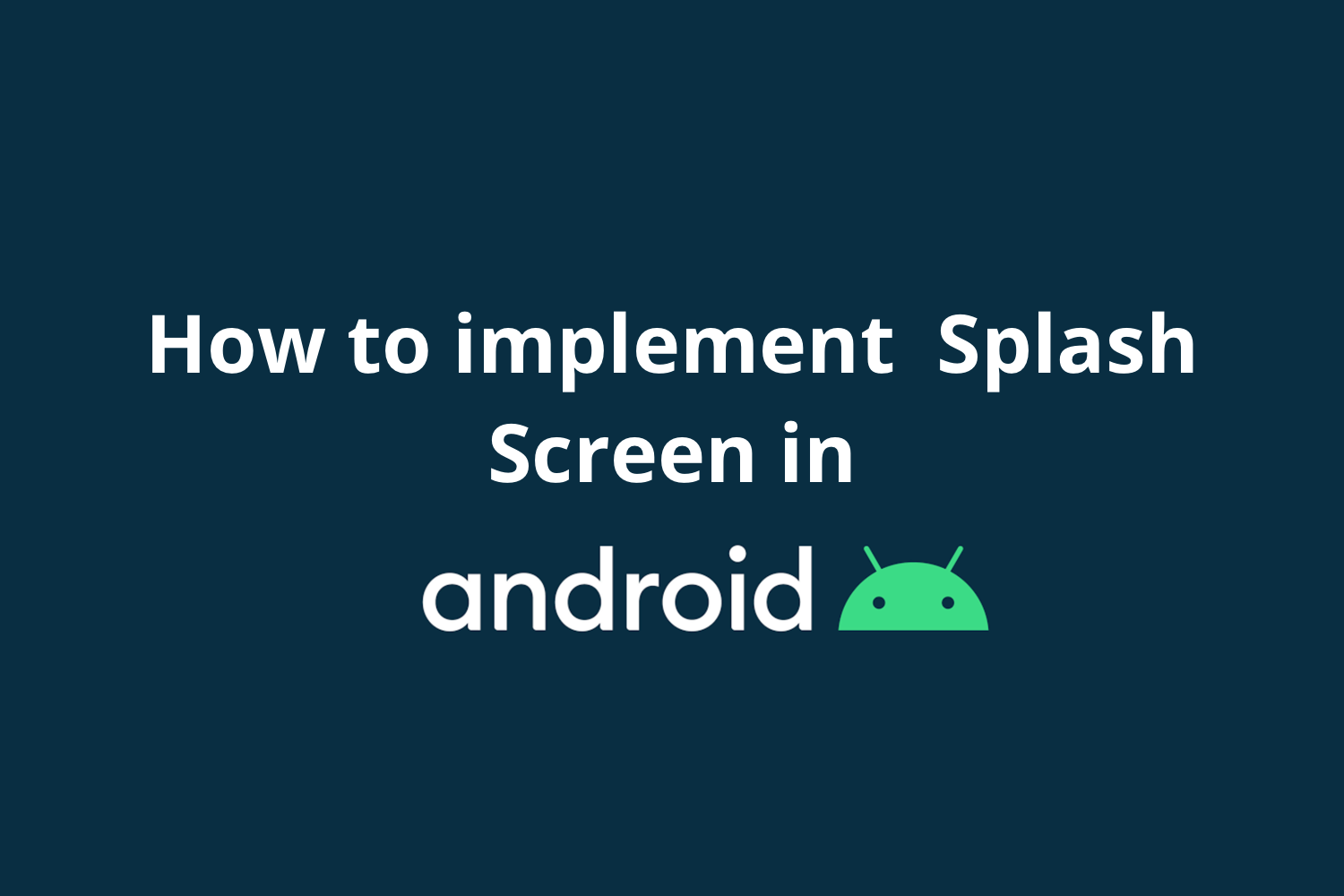
Ever seen a screen with company's logo while opening the app. These are like screens either to do some background task or to just showcase the company branding.After the Spalsh Screen, the main landing screen comes up after some seconds like the dashboard screen or any other screen.
To create a Splash Screen we have two ways,
- Traditional Way.
- The Reactive Way.
What we are gonna do in this blog?
First, we will create a project and in that we will have two Activities. SplashActivity and MainActivity . And when we run the app, in which we want to navigate from SplashActivity to MainActivity after some seconds.
Now, Let's discuss both the ways how to design Splash Screen
Traditional Way
- We have been doing this till data the traditional way using Handlers.
- Handlers are basically background threads which allows you to communicate with the UI thread (update the UI) after certain amount of time.
- We will set time to handler and call Handler().postDelayed, it will call run method of runnable after set time and redirect to MainActivity screen.
- Handlers used to schedule some task at some point in future.
Let's Now make the SplashActivity the main launcher activity from AndroidManifest.xml
<activity android:name=".SplashActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
and in SplashActivity, we will create a function startMainActivityUsingHandler,
private fun startMainActivityUsingHandler() {
handler.postDelayed(runnable, 5000)
}
and we will call startMainActivityUsingHandler() in onCreate of SplashActivity.
Here, handler and runnable are defined in init() method which gets called in onCreate of SplashActivity
private fun init() {
handler = Handler()
runnable = Runnable {
openMainActivity
}
}
This is how we use Handler to navigate from SplashActivity to MainActivity.
Note : When you have close the SplashActivity or press back button we need to remove all the pending callback and messages of the Handler in onStop()
override fun onStop() {
super.onStop()
handler.removeCallbacks(runnable)
handler.removeMessages(0)
}
Now, lets the discuss the Reactive Way.
Reactive Way
In this blog, we will be learning the new way to create Splash Screen using RxJava2.We will be using RxJava Timer operator in this blog. Timer operator is used when we want to do something after a span of time that we specify.
Sounds familiar?
It is similar to what Handler was doing it for us. Click here to learn more about Timer Operator.
Now, we will create a function startMainActivityUsingRxJava() in SplashAvtivit y and we will delete the startMainActivityUsingHandler() function.
private fun startMainActivityUsingRxJava() {
compositeDisposable.add(Observable.timer(5, TimeUnit.SECONDS)
.subscribe {
openMainActivity
})
}
In the above function, we will create an Observable that emits after a specified delay and then completes. It is added in Disposable so that we can dispose it onStop().
override fun onStop() {
super.onStop()
compositeDisposable.dispose()
}
Here, using Timer operator the task of openMainActivity will execute after 5 seconds.
This is how we can use RxJava to design our SplashActivity.
Happy learning
Team MindOrks :)