Getting Started with Sharing Shortcuts in Android Q
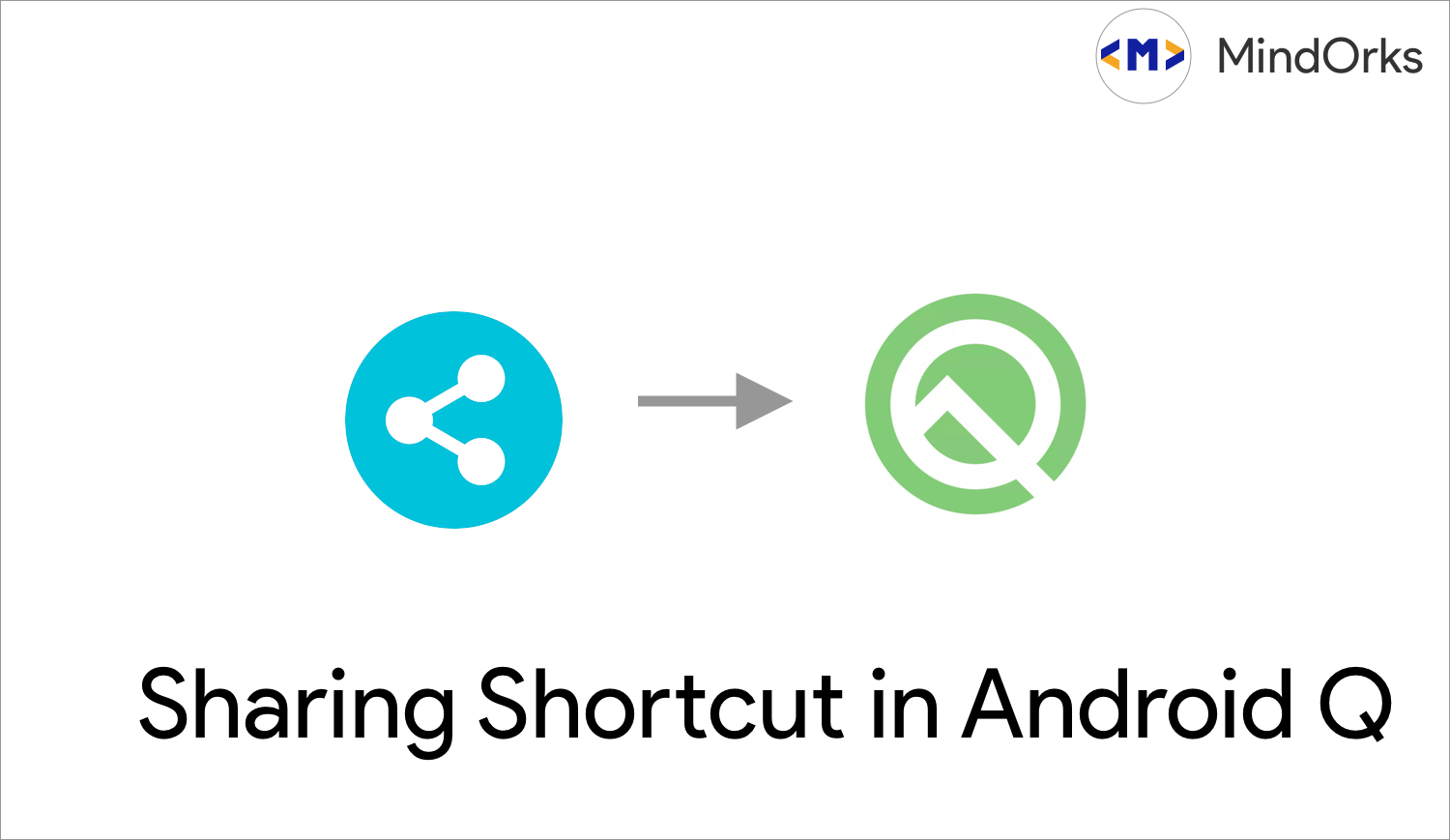
With Andorid Q launching with some awesome features, It also launched Sharing Shortcuts in Android Q. In Q, Direct Share API is replaced with Sharing Shortcut API .
What was Direct Share API?
With Android M, Android provided this feature in which we can define direct user targets. This targets were shared to user by via Share Menu. This feature allows users to share content to targets, such as contacts, within other apps. For example, the direct share target might launch an activity in another social network app, which lets the user share content directly to a specific friend or community in that app.
In Android Q, Direct Share API will still keep on working but with Low Priority then Sharing Shortcuts.
Differences between Direct Share API and Sharing Shourtcut API
- Sharing Shortcut API is faster compared to Direct Share API as it provides the list of targets before hand only.
- In Direct Share API, we required to write a bit of code for ChooserTarget Service but in Sharing Shortcut it is handled by the API itself.
- The new API uses a push model, versus the pull model used in the old DirectShare API. This makes the process of retrieving direct share targets much faster when preparing the ShareSheet. From the app developer's point of view, when using the new API, the app needs to provide the list of direct share targets ahead of time, and potentially update the list of shortcuts every time the internal state of the app changes (for example, if a new contact is added in a messaging app).
In this blog, we will learn how to implement the Sharing Shortcut API step by step
Step 01
First we have to implement share target element in XML under res/xml folder.
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<share-target android:targetClass="com.mindorks.app.ShareActivity">
<data android:mimeType="text/plain" />
<category android:name="com.example.android.sharingshortcuts.category.TEXT_SHARE_TARGET" />
</share-target>
</shortcuts>
Here,
- <share-target> tag defines the shortcut and in this code it will be handled by ShareActivity and will respond to content type text/plain.
- <data> tag defines the type of data
- < category> tag defines the category of the data used to match an app's published shortcuts with its share target definitions.
Step 02
1. We have to add the following to create a reference in Manifest file to support old Direct Share API for backward copatibility
2. The App Manifest should contain the meta data to work with compatible library.
<activity android:name=".ShareActivity">
<!-- This activity can respond to intents with an action SEND and a content type text/plain -->
<intent-filter>
<action android:name="android.intent.action.SEND"/>
<category android:name="android.intent.category.DEFAULT"/>
<data android:mimeType="text/plain"/>
</intent-filter>
<!-- Needed if you import the sharetarget androidx library. It allows for this activity to be taken into account as a chooser target provider -->
<meta-data android:name="android.service.chooser.chooser_target_service"
android:value="androidx.sharetarget.ChooserTargetServiceCompat"/>
</activity>
Step 03
Now we have to add <meta-data> tag in our launched activity to create the reference of Share Shortcut.
<activity android:name=".LauncherActivity">
...
<meta-data android:name="android.app.shortcuts"
android:resource="@xml/shortcuts"/>
</activity>
Step 04
Now we have to add our shortcuts, to make sure when the sharesheet opens it is already there.
fun addShareShortcuts(context: Context) {
val shortcutInfoList = mutableListOf<ShortcutInfoCompat>()
shortcutInfoList.add(
ShortcutInfoCompat.Builder(context, /* A unique id for this shortcut */)
.setShortLabel("text")
.setPerson(Person.Builder()...build())
.setIcon(/* Icon */).setLongLived()
.setCategories(/* One or multiple categories defined in res/xml/shortcuts.xml */)
.setIntent(Intent(Intent.ACTION_DEFAULT))
.build())
ShortcutManagerCompat.addDynamicShortcuts(context, shortcutInfoList)
}
Here,
- setShortLabel sets the text for shortcut
- setPerson adds a relevant person which is needed for the the shortcut. (if we want to add multiple person, we can use setPersons() )
- setLongLived means when a shortcut is set to be long lived, it means that system services can still access it from the cache even after it has been removed as a dynamic shortcut.
Now, to test just try sharing a text from chrome and you will see a screen like following,
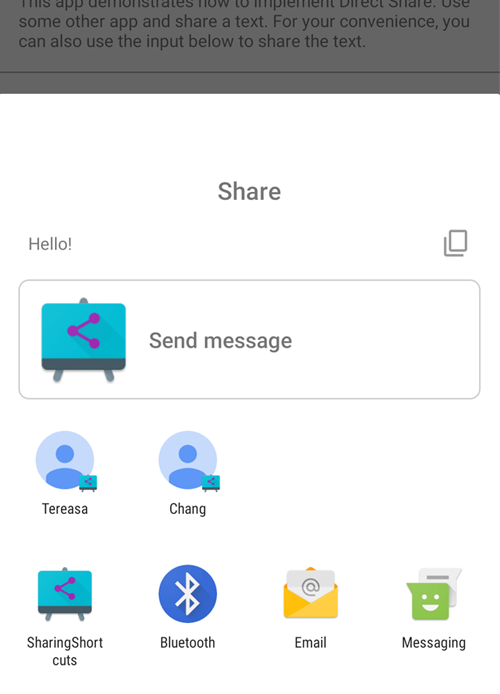
Credit : Google Sample
and also if we long press the App icon we will se the following,
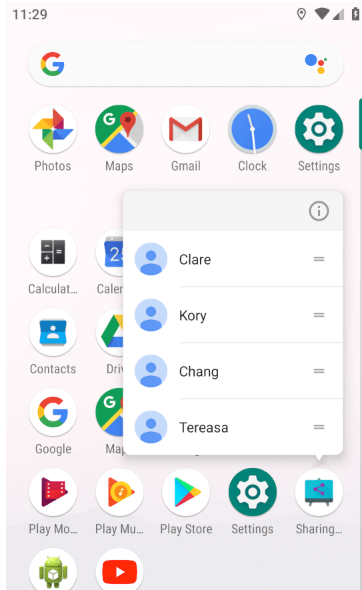
Credit: Google Sample
That is how we can get started in using Sharing Shortcuts in our app.
You can learn more from here
Happy Learning :)
Team MindOrks