Understanding Init block in Kotlin
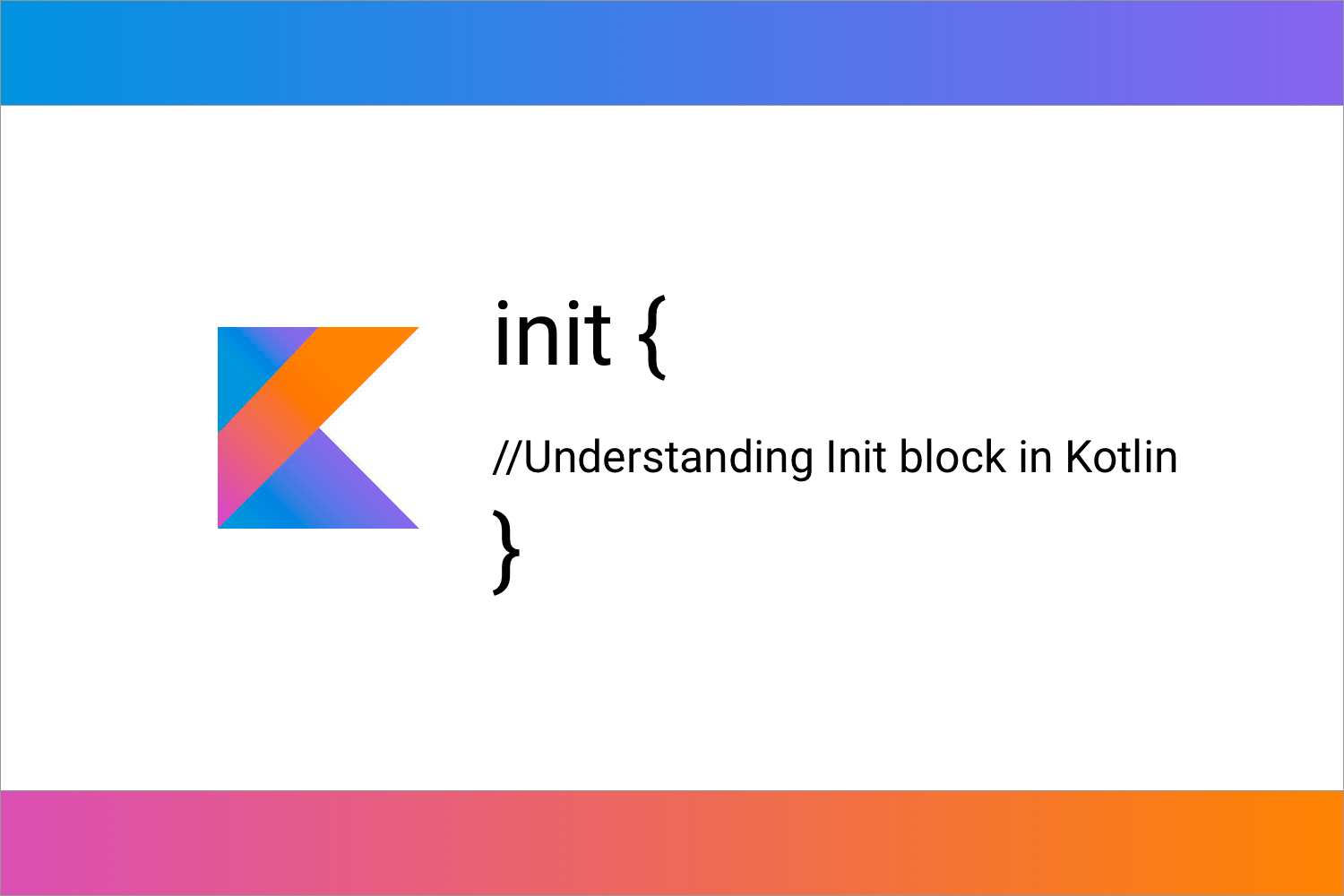
In this blog, we will learn about Init block in Kotlin. But, before starting if you are familiar with Java, you must be familiar with the concepts of Constructors.
Constructor is a block of code which get initialised when the object is created.
class SumOfNumbers {
SumOfNumbers() {
}
}
In Java, the constructor has the same name as of the class. But in Kotlin we have something different for constructors i.e Primary and Secondary constructors. You can read more about them here .
class Person(name:String,age:Int) {
}
This is an example of a Kotlin class having a primary constructor. But like java, if we have to perform some task in constructor how can we do that in Kotlin? Because this is not possible in the primary constructor.
Either we can use secondary constructor or we can use init block. Here, in this block, we will talk about the Init Block.
Let us understand the init block with an example.
In the above person class, we have to check if the person is older than me or not. We can do it using,
class Person(name: String, age: Int) {
val isOlderThanMe = false
val myAge = 25
init {
isOlderThanMe = age > myAge
}
}
Here, we have initialized two variables isOlderThanMe and myAge with a default value is false and 25 respectively.
Now, in the init block, we check the age from the primary constructor with myAge and assign the value to isOlderThanMe . i.e. if the age is greater than 25, value assigned will be true else false.
To check this,
var person = Person("Himanshu", 26)
print(person.isOlderThanMe)
This will print the desired result. As when we have initialized the Person class with passing data name as Himanshu and age as 26. The init block will also get called as the object is created and the value of isOlderThanMe has been updated based on the condition.
Points to Note:
- The init block is always called after the primary constructor
- A class file can have one or more init blocks executing in series i.e. one after another.
Happy learning
Team MindOrks :)