Type Aliases in Kotlin
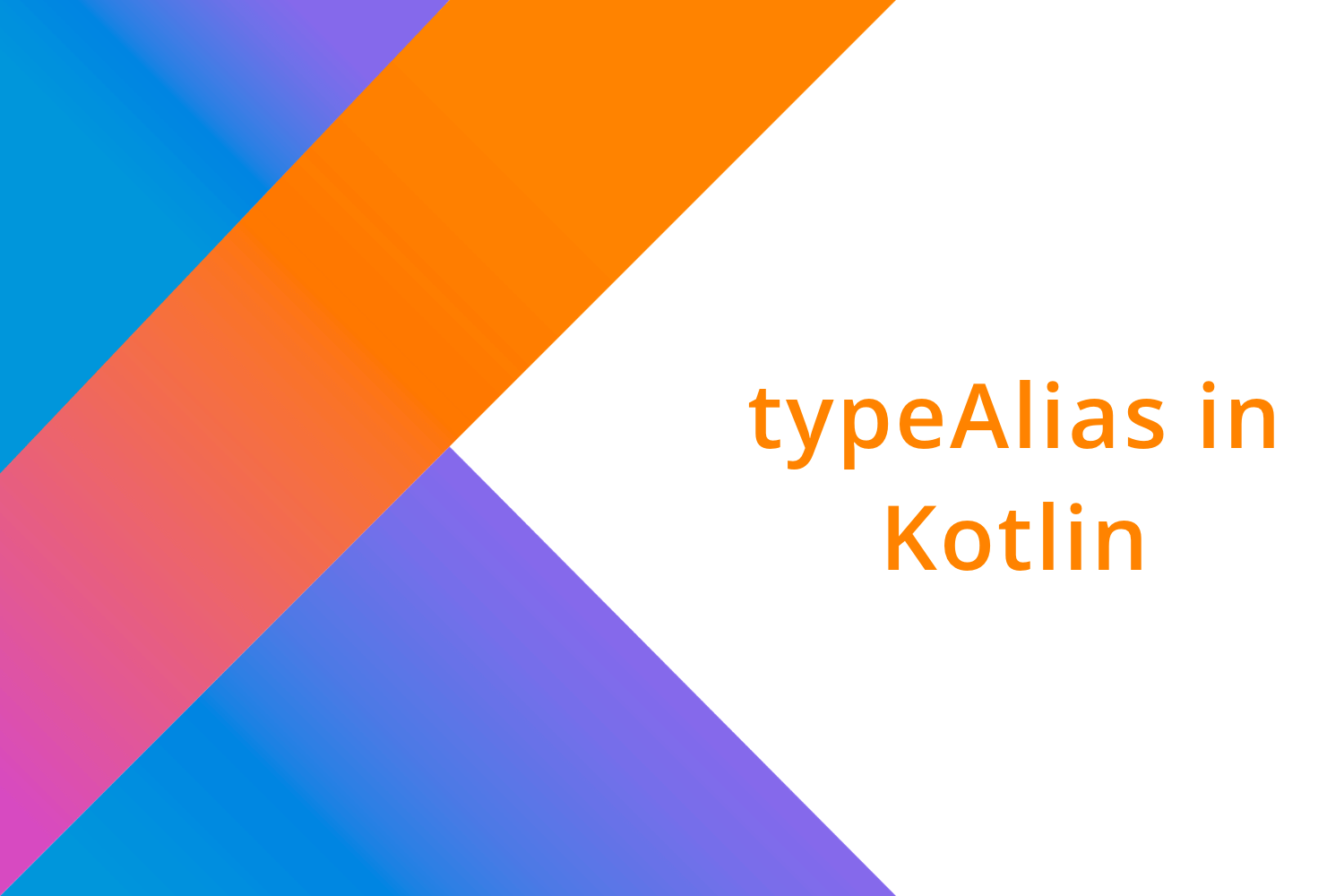
In a project, we might declare variables of the same datatype multiple times like declaring a variable of Api response class type in Android or maybe using the same function again and again which might not make your code readable.
Using type alias , in Kotlin we can make our code more readable for the developers.
In this blog, we will see how to use type alias and what is the benefit of it. We are going to learn about,
- What are Type Aliases in Kotlin?
- How to use it?
- Benefits of using typeAlias
What are Type Aliases in Kotlin?
typeAlias in Kotlin means a way to give an existing type a new name. It provides an alternative naming to your existing type to reflect a more readable code.
How to use it?
Now, we will understand the use of typeAlias with examples.
Case 01.
Consider we have an example where we have triple, like,
Triple<String, String, Int>
Now, let's say this triple will have user details like firstName , lastName , and age .
And then, we will have a function of return type Triple returning the user details like,
fun fetchUser():Triple<String, String, Int>{
return Triple("Himanshu","Singh",26)
}
Now, if we have to write this function multiple times in different classes, again and again, the code will not look good. In fact, returning Triple every time we create the same function we have to pass the return type of Triple .
To make the code aesthetically correct, we use typeAlias .
Here, we will make a typeAlias for the Triple like,
typealias User = Triple<String, String, Int>
Now, we can update the function like,
fun fetchUser(): User {
return Triple("Himanshu", "Singh", 26)
}
Here, you can see, now we have a return type of User in place of Triple . This makes our code more explanatory that now what we are returning is the detail of User .
This is why we use typeAlias to make our code more explanatory and readable.
Case 02.
Consider an example, when we have to load the resource from drawable or let's say we load XML file from our layout folder.
For both of them, we use R.drawable.drawable_name or R.layout.layout_name and then load it to the view. In our projects, we use a lot of drawables and layouts and hence we have to repeat R.drawable and R.layout a lot of times.
We can convert them using typeAlias to reduce the re-writing again and again. To move it to typeAlias we use,
typealias Drawable = R.drawable
typealias Layout = R.layout
Here, if you see we created Drawable and Layout typeAliases pointing to R.drawable and R.layout respectively.
Now, to use it in our project we will use it as,
setContentView(Layout.activity_main)
and for drawable we will use it like,
imageView.setImageDrawable(ContextCompat.getDrawable(this, Drawable.ic_launcher_background))
Here, you can see we have replaced R.drawable and R.layout with its typeAliases.
Case 03.
Now, consider an example when we have to make a custom TextView which might have some additional feature then the TextView provided by Android.
So, I will create a TextView class inside our customView package that would have our implementation of textView.
Now, while using it, we might have declared a variable like,
lateinit var textView :com.mindorks.example.customView.TextView
The reason here is we have named the View class as TextView similar to what Android has, i.e. TextView.
Here, we have a name conflict of TextViews. Generally, when resolving the conflict what we do is in the import statement we update it like,
import com.mindorks.example.customView.TextView as MyTextView
and then in place of writing the conflicting name we just use MyTextView .
But, we can also do it in a better way by using typeAlias .
To solve this conflict with typeAlias we will create a typeAlias for our textView implementation like,
typealias MyTextView = com.mindorks.example.customView.TextView
and now to use it, we will use it as,
lateinit var textView : MyTextView
Here, we are using MyTextView as type as we created an alias for it.
While decompiling it to Java equivalent, it will still keep it as,
com.mindorks.example.customView.TextView
and typeAlias would not be there.
Case 04.
Let's say we have an RecyclerView adapters and in all of them we need to setup a click listener. The listener will pass the position and the clicked data of that position on the click.
So, let's create a typeAlias like,
typealias ItemClickListener<T> = (position: Int, data: T) -> Unit
Here, we created a ItemClickListener which takes a position and data of generic. It can be used at different adapters of RecyclerView.
Now, to use this in RecyclerView Adapter for displaying the list of Post we will declare it like,
lateinit var itemClickListener: ItemClickListener<Post>
Now, on the click listener of the item in RecyclerView adapter, we will add,
itemClickListener(position, data)
where, data is the Post data of the item at the specific position which is clicked.
Now, to utilise the listener, we will call it in our view class like,
postAdapter.itemClickListener = { position, data ->
// do something on item click at that position
}
This will get you the position of the list where it was clicked along with the data of type post at that position.
Benefits of using typeAlias
We should use typeAlias to,
- Make our code more readable
- While creating an alternative name, it doesn't create a new type.
Note: typeAlias should be declared from the top level of our Kotlin code and not like inside of a class or a function. Declaring it inside function/class will throw an error.
This is all about typeAlias in Koltin.
Happy learning.
Team MindOrks :)