Operator Overloading in Kotlin
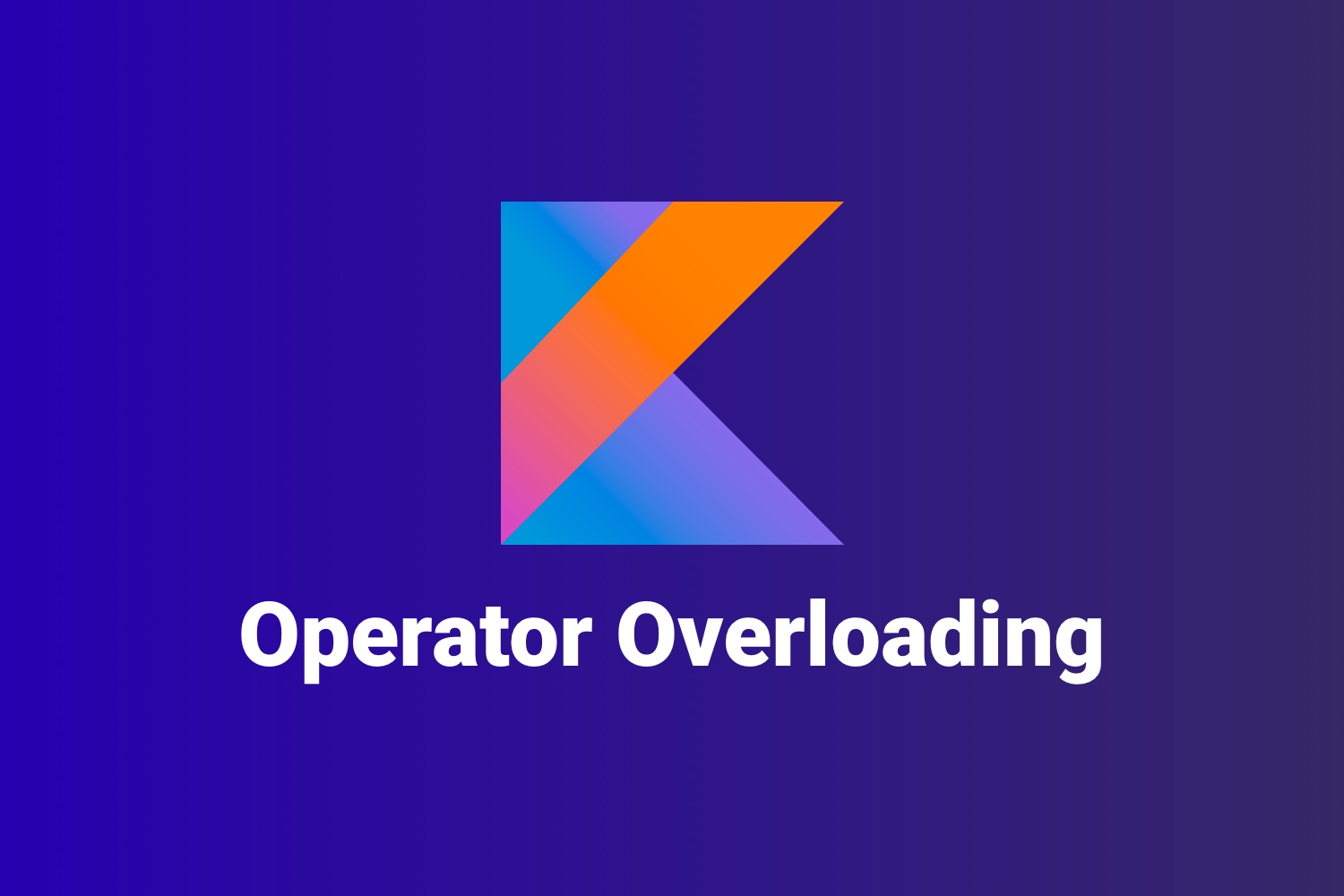
In this tutorial, we are going to learn Operator Overloading in Kotlin by covering the following:
- What is Operator Overloading?
- Learning it with the help of an example.
What is Operator Overloading?
The operator is basically a symbol for performing a certain operation on one or more operands. Generally, we perform operations or actions through the function, but we can also perform operations by the symbols. Those symbols are operators.
These all symbols(operators) have corresponding functions. When we use the symbol, internally the corresponding function to that symbol gets executed.
All operators have their corresponding functions, hence, in simple words, we can say they have their default behaviors. Kotlin language gives us the opportunity to modify the default behaviors of some of the operators.
Now we come to know that, what an awesome language Kotlin is!
Remember the symbolic representation of the operator and it’s corresponding function’s name both are fixed.
Learning it with the help of an example
We have data class
Pen
having a property
inkColor
and method
showInkColor()
data class Pen(val inkColor:String){
fun showInkColor(){ println(inkColor)}
}
and one extension method
combine()
fun Pen.combine(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
this extension function returns us new
Pen
having colors from both
Pens
.
Our code that uses the above data class and extension method is
fun main() {
val bluePen = Pen(inkColor = "Blue")
bluePen.showInkColor()
val blackPen = Pen(inkColor = "Black")
blackPen.showInkColor()
val blueBlackPen = bluePen.combine(blackPen)
blueBlackPen.showInkColor()
}
We would like to improve the readability of our code by using operator overloading. We indirectly come to know the use of operator overloading is. let’s highlight it. Operator overloading improves code readability.
We can apply the Operator overloading with the member function and extension function.
In our example, we are applying it with extension function combine() . So, we will first look at operators that Kotlin allows us to overload, and depending upon our code suitability and use case we need to choose one operator. For our case, the + operator makes sense. Also, note down the corresponding method name for this operator. the corresponding method name is plus(). Now, we are just a few steps away from using operator overloading.
Change the method name to the operator corresponding method name.
In our case, the corresponding method name of + operator is plus() . Hence, refactoring method name this
fun Pen.combine(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
to
fun Pen.plus(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
Use operator modifier before the fun keyword in a method declaration
changing this
fun Pen.plus(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
to
operator fun Pen.plus(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
Use operator(symbol) at the place where that method is calling
change this
val blueBlackPen = bluePen.plus(blackPen)
to
val blueBlackPen = bluePen + blackPen
the default behavior of + operator is changed.
Congratulations! we have successfully implemented an Operator overloading.
Our whole code is
fun main() {
val bluePen = Pen(inkColor = "Blue")
bluePen.showInkColor()
val blackPen = Pen(inkColor = "Black")
blackPen.showInkColor()
val blueBlackPen = bluePen + blackPen
blueBlackPen.showInkColor()
}
operator fun Pen.plus(otherPen: Pen):Pen{
val ink = "$inkColor, ${otherPen.inkColor}"
return Pen(inkColor = ink)
}
data class Pen(val inkColor:String){
fun showInkColor(){ println(inkColor)}
}
Output:
Blue
Black
Blue, Black
For further reading about Operator overloading, please refer to the official documentation .
Do share this blog and you can reach out to me on Twitter and Linkedin .