How to check the visibility of software keyboard in Android?
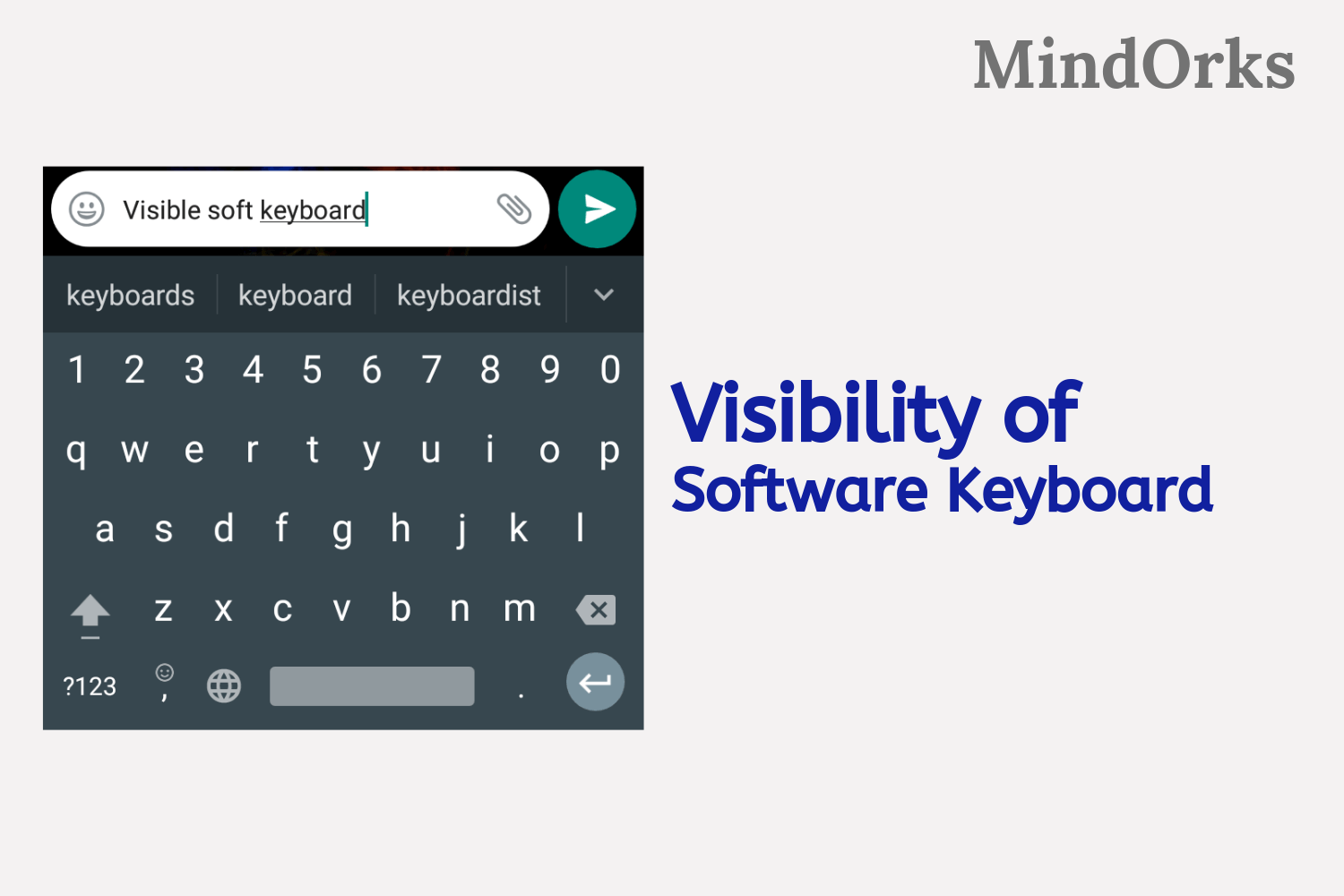
Keyboards are one of the basic elements of any Android application. Usually, we use keyboards for taking inputs from the user. These keyboards that are used in the Android application are called soft keyboards and Android system provides you keyboard for taking input from the user in any EditText or something like that. Also, if the user has given the input then that keyboard will be made invisible from the screen. So, in this blog, we will learn how to check if the software keyboard is visible in the Android application or not. If it is visible then how to make this keyboard invisible or how to close the keyboard. So, let’s get started.
Create a new project in Android Studio and name it as per your choice. In my case, I am using HideKeyboard as the name of my project.
As always, after creating a project in Android studio, our next task is to add the UI of the application. So, in the activity_main.xml file, add the below code:
<?xml version = "1.0" encoding = "utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rootview"
tools:context=".MainActivity">
<EditText
android:id="@+id/editext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintEnd_toEndOf="parent"
android:layout_marginEnd="8dp"
app:layout_constraintStart_toStartOf="parent"
android:layout_marginStart="8dp">
</EditText>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hide Keyboard"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
After writing the code for the UI part now let’s move on to the logic part of the application.
So, here we will use the concept of viewTreeObserver . With the help of viewTreeObserver, we can find if there is some change in the viewGroup in a particular activity or not. After that, we can find the height of the current screen and then the height of the keyboard and by using this height we can determine if the keyboard is visible or not. So, let’s look at the code of the MainActivity.kt file:
class MainActivity : AppCompatActivity(), View.OnClickListener {
lateinit var constraintLayout:ConstraintLayout
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var button = findViewById<Button>(R.id.button)
val editText = findViewById<EditText>(R.id.editext)
editText.requestFocus()
constraintLayout = findViewById(R.id.rootview)
constraintLayout.viewTreeObserver.addOnGlobalLayoutListener {
val rec = Rect()
constraintLayout.getWindowVisibleDisplayFrame(rec)
//finding screen height
val screenHeight = constraintLayout.rootView.height
//finding keyboard height
val keypadHeight = screenHeight - rec.bottom
if (keypadHeight > screenHeight * 0.15) {
Toast.makeText(this@MainActivity, "VISIBLE KEYBOARD", Toast.LENGTH_LONG).show()
} else {
Toast.makeText(this@MainActivity, "NO KEYBOARD", Toast.LENGTH_LONG).show()
}
}
button.setOnClickListener(this)
}
@RequiresApi(api = Build.VERSION_CODES.O)
override fun onClick(v:View) {
when (v.id) {
R.id.button -> hideSoftkeybard(v)
}
}
//function to hide keyboard
private fun hideSoftkeybard(v:View) {
val inputMethodManager = getSystemService(INPUT_METHOD_SERVICE) as InputMethodManager
inputMethodManager.hideSoftInputFromWindow(v.windowToken, 0)
}
}
Here, we are using hideSoftKeyboard to hide the keyboard if the keyboard is visible on clicking the button.
So, finally, run the application and see the output on your mobile device.
In this blog, we learned how to check if the keyboard is visible or not on the screen. Also, we have if the keyboard is visible then how to close it. That’s it for this blog.
To learn more on some cool Android topics, you can refer to MindOrks blogging website .
Keep Learning :)
Team MindOrks!