Downloading and Showing Image with Glide Library in Android
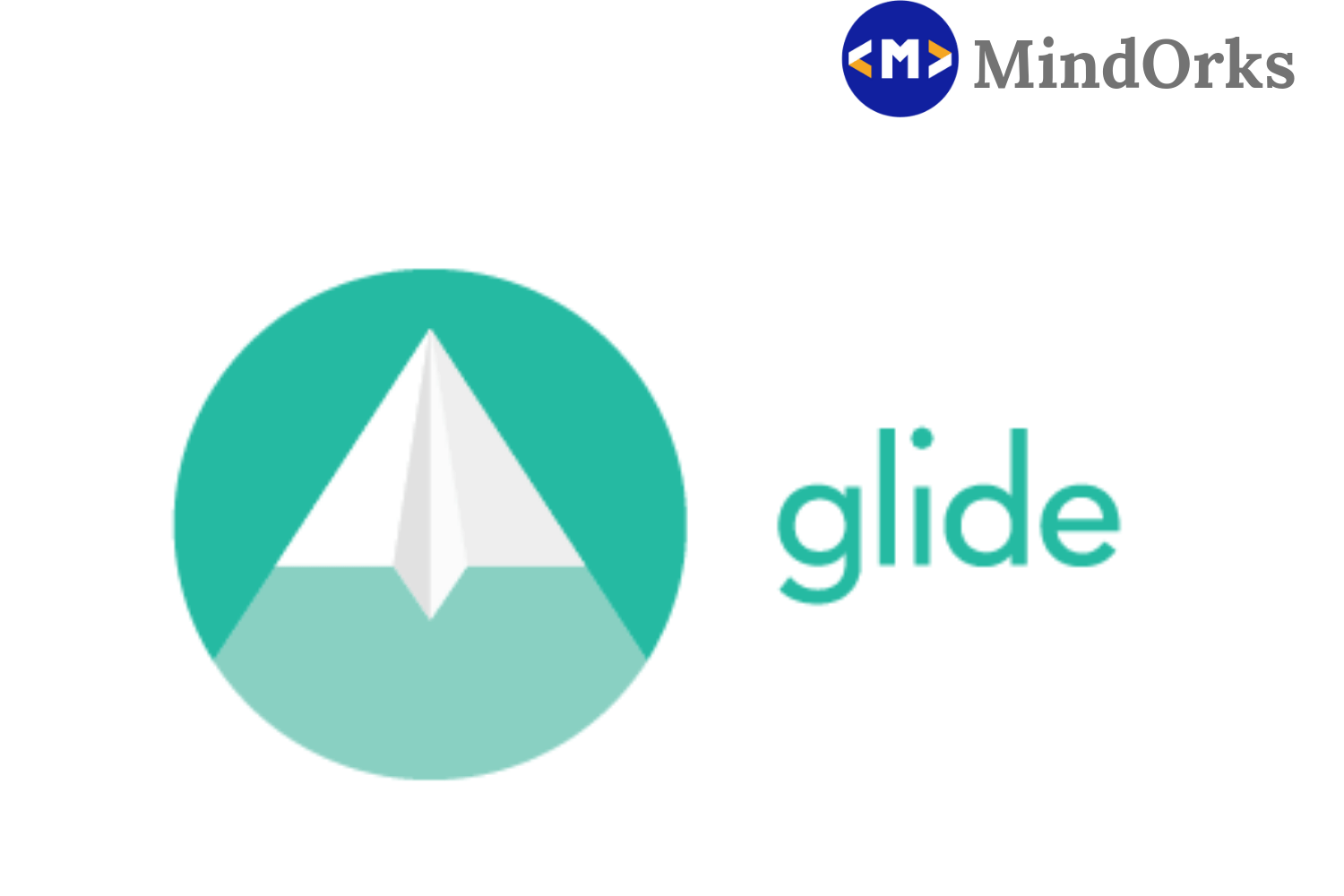
Today, we are having mobile applications for each and every task that we do throughout the day. And we love to use the application that is more interactive and have better UI. And the most important thing that makes an App more interactive is the use of images.
Yes, images play a major role in making the UI of an App more interactive and user-friendly. So, as an Android Developer, we should take care of using images in our App. We should handle each and every aspect of an image like the slow loading aspect, unresponsive image, memory issues and many more. If you are not handling these aspects, then your app will have a bad impression on your users.
In order to manage every aspect of images that are used in Android Application, Google recommended the use of Glide library in the Google I/O 2014. So, in this blog, we will learn how to use Glide libraries to download image and use it in our application. The outline of this blog is as follows:
- What is Glide?
- Features of Glide Library
- Glide usage in Android
- Some more features
- Conclusion
What is Glide?
To be more precise, Glide is an open source media management framework for Android that wraps media decoding, memory and disk caching, and resource pooling into a simple and easy to use interface.
In other words, Glide Library is a popular android library for image downloading and caching and is developed by bumptech . It is basically focused on smooth scrolling. Many times, it is found that if you try to load an image of a very big size, then your app will not be smooth. The scrolling will not be smooth but by using Glide you can get rid of this problem.
The primary focus of Glide is to make scrolling smooth and as fast as possible. But Glide can also be used for fetching, resizing and displaying a remote image.
Features of Glide Library
Glide supports a large number of features. Some of these are:
- Use of GIF: In Glide, you can use GIF also. Gone are the times, when you put a static image to your app. Now, you can use GIF using Glide.
- Disk caching: Glide downloads the image from the given URL, resize it to the size of the image view where you want to display the image and stores it to the disk cache. Glide will store two different copies of same image if you are putting the same image in two different Image Views. That will increase the disk cache size, but it will increase the processing speed.
- Thumbnail support: With Glide, we can load multiple images into the same view at the same time i.e we can add thumbnails to our image view.
- Customization: The best thing about Glide is, it provides various configurations and customization options. So, you can change the Glide library as per your requirement.
Glide usage in Android
Int his section of this bolg, we will look upon the implementation of Glide in Android using Kotlin. To use Glide library in your Android application, first of all, add the Glide library dependency to your app/build.gradle file:
dependencies {
...
//glide library
implementation 'com.github.bumptech.glide:glide:4.9.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.9.0'
...
}
Now after adding the above dependency, sync your project. This will add the Glide library to your project. After that, add internet permission in AndroidManifest.xml . This is required because we need the internet to download the image:
<uses-permission android:name="android.permission.INTERNET"/>
Now, you are done with all the dependencies and permissions. It’s time to code for the Glide library. In the glide_example.xml write the following code to add a LinearLayout. We will use this Linearlayout to display the downloaded image:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
</LinearLayout>
Till now we are done with the UI part. Now in the GlideExample.kt file, add the below lines:
class GlideExample : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.glide_example)
val linearLayout = findViewById<LinearLayout>(R.id.linearLayout)
val imageView = ImageView(this)
Glide.with(this).load("https://moodle.htwchur.ch/pluginfile.php/124614/mod_page/content/4/example.jpg").into(imageView)
linearLayout.addView(imageView)
}
}
Let’s understand the above code. In the above code, we created an instance of LinearLayout to display the downloaded image. To simply load an image to LinearLayout, we call the with() method of Glide class and pass the context, then we call the load() method, which contains the URL of the image to be downloaded and finally we call the into() method to display the downloaded image on our ImageView .
Some more features
- Resizing image: You can resize an image using the override() method that will take the final dimensions of the image.
Glide.with(this)
.load("https://moodle.htwchur.ch/pluginfile.php/124614/mod_page/content/4/example.jpg")
.override(300, 200)
.into(resizeImage);
- Adding placeholder and error message: Adding a placeholder for an image is always a nice option because there may arise a case when the internet speed is slow and in that condition, your image will not be loaded in few seconds. So, to handle those situations we use some placeholder that will be displayed until your original image will be loaded.Also if there is some error during the image fetching then the user should know that some error has occurred. That error may arise due to the wrong URL or due to no internet connectivity. To set a placeholder and an error, use the following:
Glide.with(this)
.load("https://moodle.htwchur.ch/pluginfile.php/124614/mod_page/content/4/example.jpg")
.placeholder(R.drawable.placeholder) //placeholder
.error(R.drawable.error) //error
.into(placeholdeImage);
- Crop an image: You can crop an image using the centerCrop() method:
Glide.with(CropGlide.this)
.load("https://moodle.htwchur.ch/pluginfile.php/124614/mod_page/content/4/example.jpg")
.centerCrop()
.into(cropImage);
- Adding a progress bar: Adding a progress bar until the image is loading is always a good to have choice. So, to add a progress bar while the image is downloading, we use the same approach as used in error setting:
progressBar.setVisibility(View.VISIBLE);
Glide.with(this)
.load("https://moodle.htwchur.ch/pluginfile.php/124614/mod_page/content/4/example.jpg")
.listener(new RequestListener<Drawable>() {
@Override
public boolean onLoadFailed(Exception e, Object model, Target<Drawable> target, boolean isFirstResource) {
progressBar.setVisibility(View.GONE);
return false; // important to return false so the error placeholder can be placed
}
@Override
public boolean onResourceReady(Drawable resource, Object model, Target<Drawable> target, boolean isFromMemoryCache, boolean isFirstResource) {
progressBar.setVisibility(View.GONE);
return false;
}
})
.into(imageView);
There are many other method that you can use in Glide library, some of those are fitCenter(), centerCrop(), etc. You can find them here .
Conclusion
In this blog, we have seen how to use the Glide library to download and show an image in an ImageView. Images are loaded from the internet and we have given access for the App to connect the internet. All the complex work of loading and handling the images are done by the Glide library. So, without putting much effort, we learned how to handle images in our Andoird project and I am pretty sure that you will explore some more methods of Glide library.
Till then, Keep Learning!
Team MindOrks :)