Difference between List and Array types in Kotlin
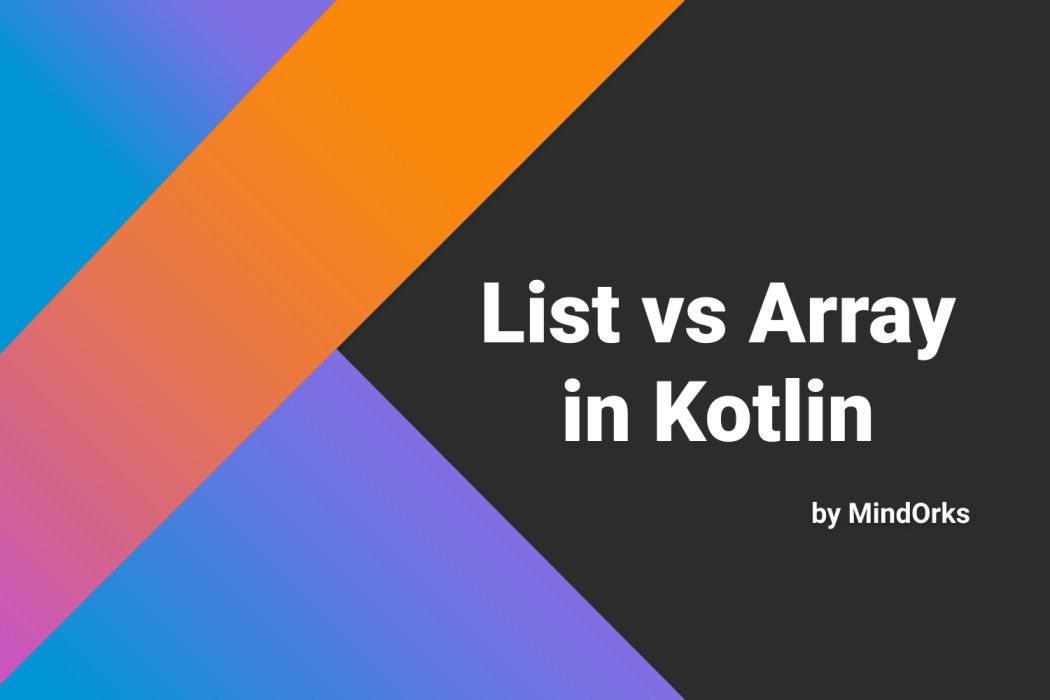
In Kotlin or any other programming languages, whenever we want to store similar types of data then all of us preferably use Arrays or Lists. Many developers think that both are the same thing and can be used interchangeably. But many developers are confused about when to use which one. So, is an Array and a List the same or they are different?? Don't worry, we will together learn and find the difference.
So, welcome to MindOrks! In this blog, we will learn the difference between List and Array in Kotlin. By the end of the blog, all your confusions will be cleared and you will know when to use Array and when to use List.
Let's start the blog by taking an example. Suppose we want to store all the courses offered by MindOrks. To do so, we can either create different variables for different courses or we can make an array or a list of courses and add the courses into it. For example:
val courses = arrayOf<String>("Android Beginners", "Android Professionals")
or
val courses = listOf<String>("Android Beginners", "Android Professionals")
So, following are the differences between List and Array:
-
If you are using
Array<T>
, then the data will be stored in a sequential manner in the storage i.e. a continuous block of storage will be allocated to store the data. But if we talk aboutList<T>
, then it an interface which may have different implementations such asArrayList<T>
,LinkedList<T>
, etc and memory representation of theseList<T>
depends on the implementation i.e. whether it is anArrayList<T>
orLinkedList<T>
or something else. -
Suppose in future, if we want to change the name of the "Android Beginners" course to "Android Basics". Then if we are using an Array of
courses
then we can simply update the array by assigning the new value because Arrays are mutable in nature . But Lists are immutable in nature . So, you can't update theList<T>
values. In order to update the values, you need to useMutableList<T>
.
val courses = arrayOf<String>("Android Beginners","Android Professionals")
courses[0] = "Android Basics" // no error
but
val courses = listOf<String>("Android Beginners","Android Professionals")
courses[0] = "Android Basics" // error
-
What if in future we want to add some more courses that may be a "React-Native" course? In this case, if we are using Arrays, then we can't add any further value to our
courses
variable because arrays have a fixed size and we can't increase or decrease the size of an array. But if we are usingMutableList<T>
, then we can add some values by using theadd
method and remove some values by using theremove
method.
val courses = mutableListOf<String>("Android Beginners","Android Professionals")
courses.add("React-Native")
-
In Kotlin,
Array<T>
is invariant i.e. it doesn't let us assign anArray<String>
Array<Any>
. -
To avoid the cost of boxing/unboxing operation, primitive data types such as int, char, double are used with arrays. There are specialized classes for these primitive arrays i.e.
IntArray
,CharArray
,DoubleArray
, etc. But Lists generally don't have optimizations for primitive datatypes.
So, these are some of the differences between a List and an Array. If you have a fixed size data then arrays can be used. While if the size of the data can vary, then mutable lists can be used.
Do share this tutorial with your fellow developers to spread the knowledge.
Apply Now: MindOrks Android Online Course and Learn Advanced Android
Learn System Design for your next Interview from here.
Happy Learning :)
Team MindOrks!
Also, Let’s connect on Twitter , Linkedin , Github , and Facebook