Android SQLite Database in Kotlin
The name of the database in Android is SQLite. SQLite is the name of the opensource SQL database that stores the data in the text file in the device. Android has its implementation.
Here, We will be learning about SQLite Database and we will also implement an application.
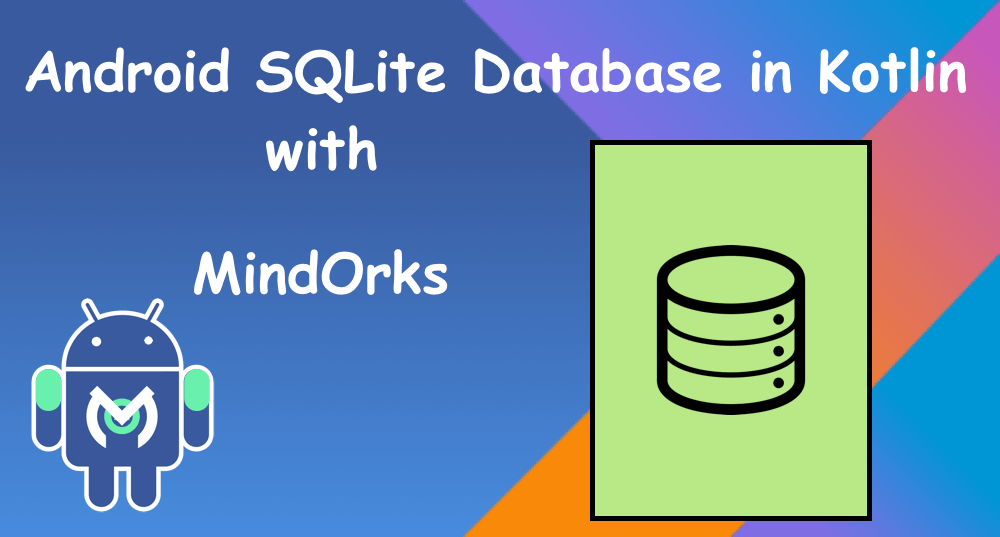
Let’s start with the implementation:We will be developing an app where a user can store a string, and it will display on the TextView on screen.Let’s first create our main screen
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/tvDisplayName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@+id/etName" />
<EditText
android:id="@+id/etName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
android:ems="10"
android:inputType="textPersonName"
android:text="Name"
app:layout_constraintBottom_toTopOf="@+id/tvDisplayName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/btnAddToDb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Add to database"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/btnShowDatafromDb" />
<Button
android:id="@+id/btnShowDatafromDb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Data"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/btnAddToDb"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Android provides us with the SQLiteOpenHelper class which helps us to interact with the SQLite Database. This class includes methods like create, insert, delete and update for data CRUD.
Let’s create a class called Name which will act as our model class
class Name {
var id: Int = 0
var userName: String? = null
constructor(id: Int, userName: String) {
this.id = id
this.userName = userName
}
constructor(userName: String) {
this.userName = userName
}
}
Let’s crea.te an OpenHelper class
class MindOrksDBOpenHelper(context: Context,
factory: SQLiteDatabase.CursorFactory?) :
SQLiteOpenHelper(context, DATABASE_NAME,
factory, DATABASE_VERSION) {
override fun onCreate(db: SQLiteDatabase) {
val CREATE_PRODUCTS_TABLE = ("CREATE TABLE " +
TABLE_NAME + "("
+ COLUMN_ID + " INTEGER PRIMARY KEY," +
COLUMN_NAME
+ " TEXT" + ")")
db.execSQL(CREATE_PRODUCTS_TABLE)
}
override fun onUpgrade(db: SQLiteDatabase, oldVersion: Int, newVersion: Int) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME)
onCreate(db)
}
fun addName(name: Name) {
val values = ContentValues()
values.put(COLUMN_NAME, name.userName)
val db = this.writableDatabase
db.insert(TABLE_NAME, null, values)
db.close()
}
fun getAllName(): Cursor? {
val db = this.readableDatabase
return db.rawQuery("SELECT * FROM $TABLE_NAME", null)
}
companion object {
private val DATABASE_VERSION = 1
private val DATABASE_NAME = "mindorksName.db"
val TABLE_NAME = "name"
val COLUMN_ID = "_id"
val COLUMN_NAME = "username"
}
}
Now, we will implement our add to database button
btnAddToDb.setOnClickListener {
val dbHandler = MindOrksDBOpenHelper(this, null)
val user = Name(etName.text.toString())
dbHandler.addName(user)
Toast.makeText(this, etName.text.toString() + "Added to database", Toast.LENGTH_LONG).show()
}
and also, our get all names from database button
btnShowDatafromDb.setOnClickListener {
tvDisplayName.text = ""
val dbHandler = MindOrksDBOpenHelper(this, null)
val cursor = dbHandler.getAllName()
cursor!!.moveToFirst()
tvDisplayName.append((cursor.getString(cursor.getColumnIndex(MindOrksDBOpenHelper.COLUMN_NAME))))
while (cursor.moveToNext()) {
tvDisplayName.append((cursor.getString(cursor.getColumnIndex(MindOrksDBOpenHelper.COLUMN_NAME))))
tvDisplayName.append("\n")
}
cursor.close()
}
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
btnAddToDb.setOnClickListener {
val dbHandler = MindOrksDBOpenHelper(this, null)
val user = Name(etName.text.toString())
dbHandler.addName(user)
Toast.makeText(this, etName.text.toString() + "Added to database", Toast.LENGTH_LONG).show()
}
btnShowDatafromDb.setOnClickListener {
tvDisplayName.text = ""
val dbHandler = MindOrksDBOpenHelper(this, null)
val cursor = dbHandler.getAllName()
cursor!!.moveToFirst()
tvDisplayName.append((cursor.getString(cursor.getColumnIndex(MindOrksDBOpenHelper.COLUMN_NAME))))
while (cursor.moveToNext()) {
tvDisplayName.append((cursor.getString(cursor.getColumnIndex(MindOrksDBOpenHelper.COLUMN_NAME))))
tvDisplayName.append("\n")
}
cursor.close()
}
}
}
Let’s try to run this app. Great Work!! You are saving the data in the database and showing it on the screen. Try to implement the delete query and explore more on this. Let us know on our slack and twitter channel.