Implementing Dark Mode Theme in Android
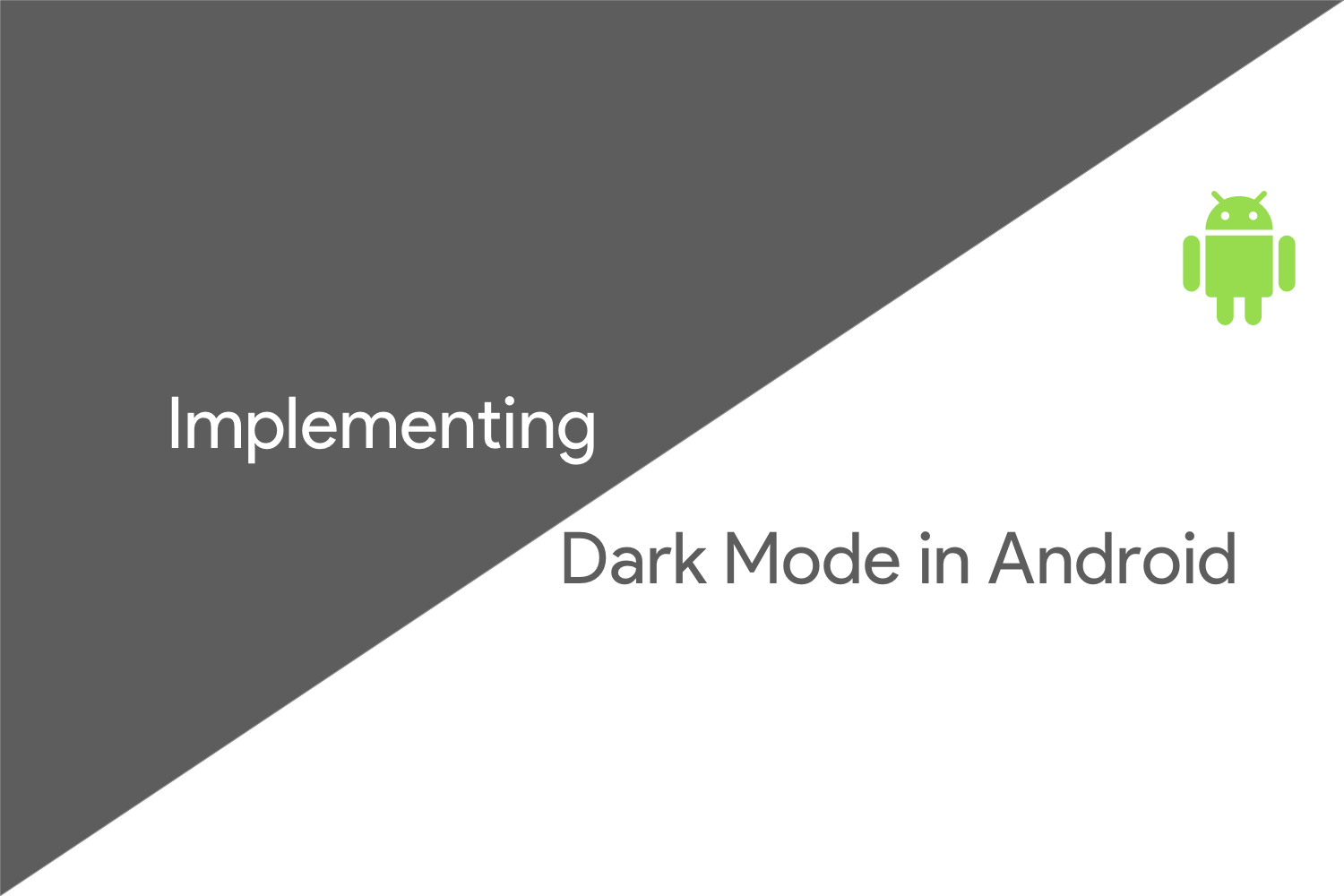
With Android Q launched by Google, Dark Theme was released with it. Dark Mode applies to both the system UI and the apps running in it.
Before starting let's understand why we needed the Dark Theme,
- Can reduce power usage by a significant amount. Some device manufacturer enable dark mode by default to the user.
- Improves eye smoothness for the user who are sensitive to bright light.
- Makes it easier for anyone to use a device in a low-light environment.
To enable Dark Mode in Android, Navigate to Settings -> Display -> Theme -> Dark Theme.
Let's Get Started
There are two ways to support Dark Theme,
To get the Dark Mode up and running your app should be targetting Android Q
- If you are using Support Library, set the app theme to
<style name="AppTheme" parent="Theme.AppCompat.DayNight">
- If you have upgraded the App theme to MaterialComponent, use
<style name="AppTheme" parent="Theme.MaterialComponents.DayNight">
and when you run the app you will see the Dark Mode in your app.
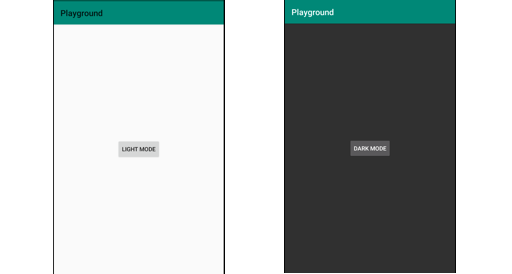
The above is the screenshot when you convert your style to Dark Mode.
Note : If you want to replace the color in the app, you need to create a custom values directory, called values-night and for image resources as well we will create drawables-night.
We can also let user to change the theme while using the app. The available options are,
- Light Theme
- Dark Theme
- Set by Battery Saver (the recommended default option for Android P or before)
- System default (the recommended default option for Android Q).
Now, to update the theme dynamically we have to use,
AppCompatDelegate.setDefaultNightMode(/**Your Mode**/)
Example,
object ThemeHelper {
private const val lightMode = "light"
private const val darkMode = "dark"
private const val batterySaverMode = "battery"
const val default = "default"
fun applyTheme(theme: String) {
when (theme) {
lightMode -> AppCompatDelegate.setDefaultNightMode(AppCompatDelegate.MODE_NIGHT_NO)
darkMode -> AppCompatDelegate.setDefaultNightMode(AppCompatDelegate.MODE_NIGHT_YES)
batterySaverMode -> AppCompatDelegate.setDefaultNightMode(AppCompatDelegate.MODE_NIGHT_AUTO_BATTERY)
default -> AppCompatDelegate.setDefaultNightMode(AppCompatDelegate.MODE_NIGHT_FOLLOW_SYSTEM)
}
}
}
In the above code, we can select the theme based on the modes.
Force the Dark :
If you don't want to upgrade the Theme in Android Q, and keep it as it is you need to add the following in the App's theme:
android:forceDarkAllowed="true"
and this will convert the whole app in Dark Mode.
Note: For Force Dark you app should target android-Q
If you need to exclude some layout or widget from dark mode just add the property in the layout,
android:forceDarkAllowed="false"
or we can do it programitcally using,
veiw.setForceDarkAllowed(true/false)
If we change the app's theme our configuration changes and because of that the Activity will be recreated. To handle the configuration,
<activity
android:name=".MainActivity"
android:configChanges="uiMode" />
we have to add configChanges property in the activity tag in Manifest and it will handle the configuration changes.
This is how we can upgrade the theme from light theme to dark theme.
Happy learning
Team MindOrks :)