How to communicate between fragments?
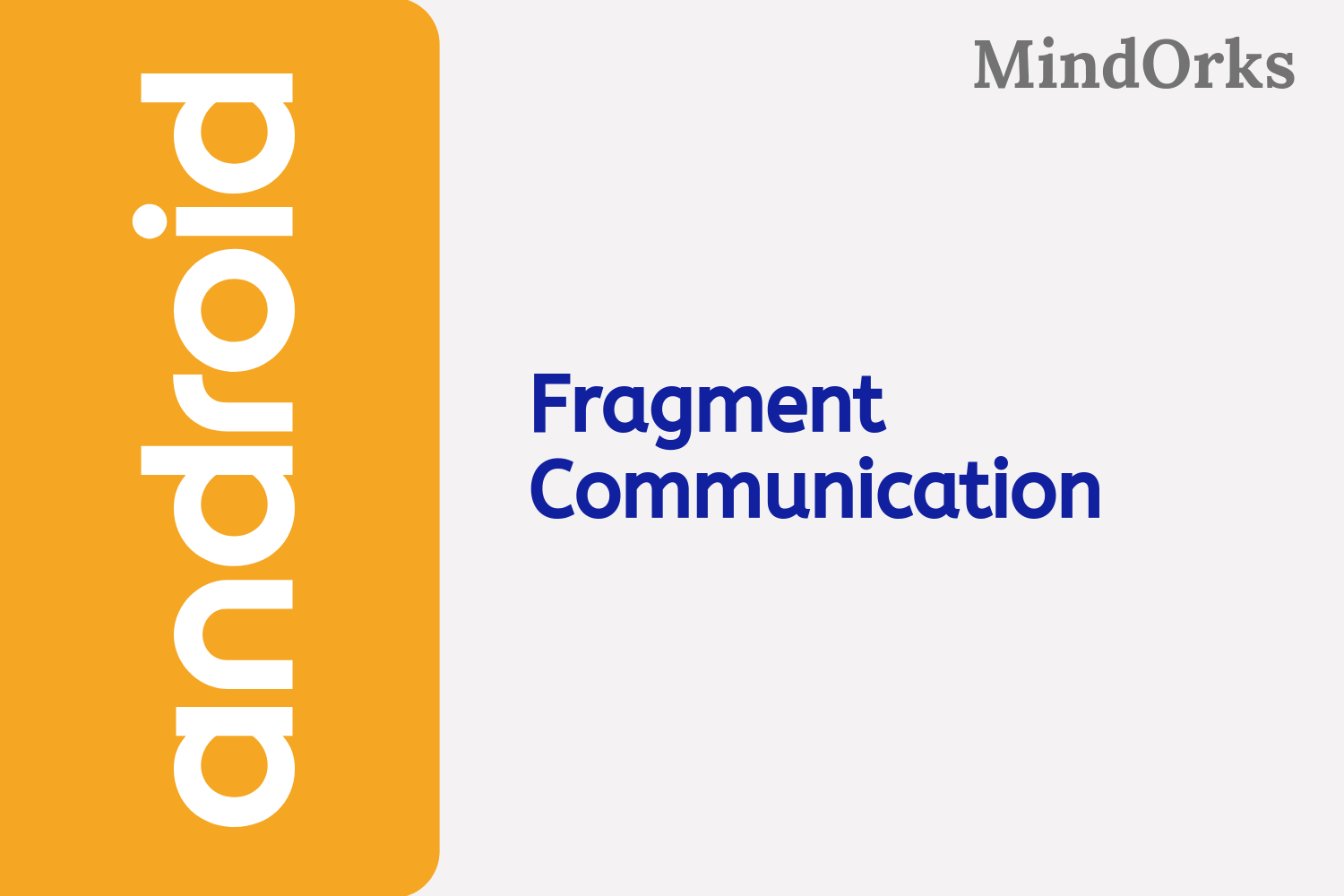
While developing an Android application, we can communicate with one Activity or Fragment to another. Communication is one of the important part in Android app development. To share data or communicate from one Activity to others is an easy task. There are many ways of doing so, for example, we can use the ViewModel class or can declare the variables to be shared as static. Another thing that can be done is you can share the data by using Intents. But if you want to share the data or communicate between two different Fragments then things become complex. You can’t treat a Fragment same as that of Activity. Like Activity, Fragments have their own life cycle. So, you should handle the lifecycle of Fragments while using it.
In this blog, we will learn how to communicate between Fragments in our Android application. So, let’s get started.
A quick revision about Fragments
A Fragment represents a behavior or portion of any user interface. For example, if you are having one Activity, then your activity may have a number of Fragments that have their own life cycle. You can think of the Whatsapp application. In the main screen, you can see four fragments present in the form of tab layout i.e. camera, chat, status and call. A Fragment must be hosted in an Activity and if the Activity will be destroyed then all the Fragments present in that activity will also be destroyed. Also, every Fragment present in an Activity has its own life cycle. So, due to this, care must be taken while sending data from one Fragment to the other. So, let’s see how we can achieve this communication.
Fragment Communication
The communication between fragments should not be done directly. There are two ways of doing so.
- With the help of ViewModel
- With the help of Interface
To have a sharing of data between Fragments, either you can use a shared ViewModel that is shared between all the Fragments or you can make an Interface and then use this interface to communicate between fragments.
The ViewModel process is very simple and you can learn how to communicate between Fragments using ViewModel by reading our Shared ViewModel blog at MindOrks .
But you should know every possible way of doing so. In this blog, we will be learning about the Interface way of Fragment communication.
So, basically we will be having one Activity and in that activity, we will be having two Fragments. Our aim is to send the data from one Fragment to the other with the help if Interface.
The idea is very simple. We can summarize the whole step into the below points:
- Make an Interface in your FragmentA
- Implement the Interface of the FragmentA in your Activity
- Call the Interface method from your Activity
- In your Activity, call your FragmentB to do the required changes
So, let’s implement this one by one. Firstly, add an Interface to your FragmentA:
class FragmentA : Fragment() {
lateinit var mCallback: TextClickedListener
//defining Interface
interface TextClickedListener {
fun sendText(text: String)
}
fun setOnTextClickedListener(callback: TextClickedListener) {
this.mCallback = callback
}
fun yourMethodofSendingText() {
//here you can get the text from the edit text or can use this method according to your need
mCallback.sendText("YOUR TEXT")
}
}
}
Here, we have made one method named yourMethodofSendingText() where you can implement the code of sending the data i.e. you can get the text value form the EditText or something else.
Our next step is to use the method of the FragmentA in our MainActivity. So, we will implement the interface of FragmentA in the Activity. The code for the MainActivity will be:
class MainActivity :Activity(), FragmentA.TextClicked {
fun onAttachFragment(fragment: Fragment) {
if (fragment is FragmentA) {
fragment.setOnTextClickedListener(this)
}
}
override fun sendText(text: String) {
// Get FragmentB
val callingFragment = getSupportFragmentManager().findFragmentById(R.id.fragment_b) as FragmentB
//calling the updateText method of the FragmentB
callingFragment.updateText(text)
}
}
Here, we are calling the updateText() method of the FragmentB by finding the fragment instance with findFragmentById. updateText() method is used to update the TextView or something else.
So, code for the FragmentB will be:
class FragmentB : Fragment() {
fun updateText(text: String) {
// Here you can perform the required action
//this action can be updating the text of the TextView with the "text" string
//or any relevant action
}
}
Here, in the updateText method, you can perform your desired operation. This operation can be updating the text of the TextView present in the FragmetnB or any relevant action that you want to perform on the data received from the FragmentA.
Conclusion
In this blog, we learned how to communicate between Fragments in our Activity. There are two ways of doing so. One is with the help of Shared ViewModel and other is with the help of Interfaces. We have seen the Interface way of doing the same. For the ViewModel approach, you can read our blog of Shared ViewModel . Hope you enjoyed this blog.
Keep Learning :)
Team MindOrks!